Hi guys,
I am working on an EA, that uses a break even function.
It uses a for loop to select the orders and make operations with them.
I open 3 order simultaneously and when the first is closed, we start modifying the rest, but from the 2 orders left(this happens with buy and sell), only one is selected, as if the last ticket order does not exist. All orders haev the same parameters - Open price, lot size, TP,SL. The first if only takes one order.
The strange thing is that when i make a test, and i know which order number to check, so i say if OrdertTcket == 12 (i know the order ticket), and print OrderTicket it returns 11, not as said 12 . So this troubles me
Hope you can help
Change the for() to:
for (int i=0; i < OrdersTotal() ; i++) {
The error on your code is because you are subtracting 1 order from ordersTotal
OrdersTotal()-1
Change the for() to:
The error on your code is because you are subtracting 1 order from ordersTotal
Why it is an error ?
Orders Total returns the amount of orders, i get order indexes, this is why i substract 1.
I tested this before, doesnt work !
Any ideas ?
Hi guys,
I am working on an EA, that uses a break even function.
It uses a for loop to select the orders and make operations with them.
I open 3 order simultaneously and when the first is closed, we start modifying the rest, but from the 2 orders left(this happens with buy and sell), only one is selected, as if the last ticket order does not exist. All orders haev the same parameters - Open price, lot size, TP,SL. The first if only takes one order.
The strange thing is that when i make a test, and i know which order number to check, so i say if OrdertTcket == 12 (i know the order ticket), and print OrderTicket it returns 11, not as said 12 . So this troubles me
Hope you can help
Your function OpenPositions() is changing the order selected.
Thanks for your answer , but how so ?
int OpenPositions() { int cnt=0; for(int i=OrdersTotal()-1;i>=0;i--) { if(!OrderSelect(i,SELECT_BY_POS,MODE_TRADES)) continue; if(OrderMagicNumber()==magic && OrderSymbol()==Symbol()) { cnt++; } } return cnt; }
Thanks for your answer , but how so ?
Hi guys,
I am working on an EA, that uses a break even function.
It uses a for loop to select the orders and make operations with them.
I open 3 order simultaneously and when the first is closed, we start modifying the rest, but from the 2 orders left(this happens with buy and sell), only one is selected, as if the last ticket order does not exist. All orders haev the same parameters - Open price, lot size, TP,SL. The first if only takes one order.
The strange thing is that when i make a test, and i know which order number to check, so i say if OrdertTcket == 12 (i know the order ticket), and print OrderTicket it returns 11, not as said 12 . So this troubles me
Hope you can help
Hello,
for buy position value is 0, and for sell value is 1.
https://docs.mql4.com/constants/tradingconstants/orderproperties
So.....
void Breakeven() { for(int i=OrdersTotal()-1;i>=0;i--) { if(!OrderSelect(i,SELECT_BY_POS,MODE_TRADES)) continue; if(OrderMagicNumber()==magic && OrderSymbol()==Symbol()) { if(OrderType()==0 && OrderStopLoss()!=openlevel)//for buy { bool modify=OrderModify(OrderTicket(),OrderOpenPrice(),openlevel,OrderTakeProfit(),0); if(!modify){Print("Order ",OrderTicket()," not modified ,op2,,error ",GetLastError());} else{Print("Order ",OrderTicket()," modified !");} Print("--------"); } if(OrderType()==1 && OrderStopLoss()!=level1)//for sell { bool modify=OrderModify(OrderTicket(),OrderOpenPrice(),level1,OrderTakeProfit(),0); if(!modify){Print("Order ",OrderTicket()," not modified,op1 ,error ",GetLastError());} Print("++++++++"); } } } }
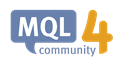
- docs.mql4.com
Hello,
for buy position value is 0, and for sell value is 1.
https://docs.mql4.com/constants/tradingconstants/orderproperties
So.....
hi, thanks for your answer. The issue is that i am not comparing order types, i am comparing the amount of orders opened
Think about it and you will finish to understand.
i have beed thinking about it for a few days. Im confused.
Still thanks for your answer, but it is not very helpful.
I write in the forum only after printing, testing, checking every value. And only when i find something i can not resolve ,i write.
Well, if you know the answer , but still want to keep it for yourself and not help, ok and thanks for everything
i have beed thinking about it for a few days. Im confused.
Still thanks for your answer, but it is not very helpful.
I write in the forum only after printing, testing, checking every value. And only when i find something i can not resolve ,i write.
Well, if you know the answer , but still want to keep it for yourself and not help, ok and thanks for everything
I don't keep anything, I said you the problem.
Forum on trading, automated trading systems and testing trading strategies
Last Order being missed in a for loop
Alain Verleyen, 2019.08.29 04:25
Your function OpenPositions() is changing the order selected.
- Free trading apps
- Over 8,000 signals for copying
- Economic news for exploring financial markets
You agree to website policy and terms of use
Hi guys,
I am working on an EA, that uses a break even function.
It uses a for loop to select the orders and make operations with them.
I open 3 order simultaneously and when the first is closed, we start modifying the rest, but from the 2 orders left(this happens with buy and sell), only one is selected, as if the last ticket order does not exist. All orders haev the same parameters - Open price, lot size, TP,SL. The first if only takes one order.
The strange thing is that when i make a test, and i know which order number to check, so i say if OrdertTcket == 12 (i know the order ticket), and print OrderTicket it returns 11, not as said 12 . So this troubles me
Hope you can help