-
double StopLoss =1.5*iATR(NULL,0,14,0); double TakeProfit=iATR(NULL,0,14,0);
Global and static variables work exactly the same way in MT4/MT5/C/C++.- They are initialized once on program load.
- They don't update unless you assign to them.
- In C/C++ you can only initialize them with constants, and they default to zero. In MTx you should only initialize them with constants.
There is no
default
in MT5 (or MT4 with strict
which you should always use.)
MT4/MT5 actually compiles with non-constants, but the order that they are initialized is unspecified and don't try to use any price or server related functions in OnInit (or on load,) as there may be no connection/chart yet:
- Terminal starts.
- Indicators/EAs are loaded. Static and globally declared variables are initialized. (Do not depend on a specific order.)
- OnInit is called.
- For indicators OnCalculate is called with any existing history.
- Human may have to enter password, connection to server begins.
- New history is received, OnCalculate called again.
- New tick is received, OnCalculate/OnTick is called. Now TickValue, TimeCurrent, account information and prices are valid.
- Unlike indicators, EAs are not reloaded on chart change so you must reinitialize them, if necessary.
external static variable - Inflation - MQL4 programming forum
- Why did you post your MT4 question in the Root / MT5
EA section instead of the MQL4 section, (bottom of the Root
page?)
General rules and best pratices of the Forum. - General - MQL5 programming forum
Next time post in the correct place. The moderators will likely move this thread there soon. -
double Buy1_2=(hf0>hf1);
What does double= bool mean? -
for (int i = 0; i < Total; i ++) { OrderSelect(i, SELECT_BY_POS, MODE_TRADES); if(OrderType() <= OP_SELL && OrderSymbol() == Symbol()) { IsTrade = True;
- Using OrdersTotal directly and/or no Magic number filtering on your OrderSelect loop means your code
is incompatible with every EA (including itself on other charts and manual trading.)
Symbol Doesn't equal Ordersymbol when another currency is added to another seperate chart . - MQL4 programming forum
MagicNumber: "Magic" Identifier of the Order - MQL4 Articles - In the presence of multiple orders (one EA multiple charts, multiple EAs, manual trading,) while you are waiting for the current
operation (closing, deleting, modifying) to complete, any number of other operations on other orders could have concurrently
happened and changed the position indexing:
- For non-FIFO
(non-US brokers), (or the EA only opens one order per symbol,) you can
simply count down, in a position loop, and you won't miss orders. Get in the habit of always counting down.
Loops and Closing or Deleting Orders - MQL4 programming forum
For In First Out (FIFO rules-US brokers,) and you (potentially) process multiple orders per symbol, you must find the earliest order, close it, and on a successful operation, reprocess all remaining positions.
CloseOrders by FIFO Rules - Strategy Tester - MQL4 programming forum - Page 2 #16 - and check OrderSelect in case earlier positions were deleted.
What are Function return values ? How do I use them ? - MQL4 programming forum
Common Errors in MQL4 Programs and How to Avoid Them - MQL4 Articles - and if you (potentially) process multiple orders, must call RefreshRates() after server calls if you want to use, on the next order / server call, the Predefined Variables (Bid/Ask) or (be direction independent and use) OrderClosePrice().
- For non-FIFO
(non-US brokers), (or the EA only opens one order per symbol,) you can
simply count down, in a position loop, and you won't miss orders. Get in the habit of always counting down.
-
Don't double
post! You already had this thread open.
General rules and best pratices of the Forum. - General - MQL5 programming forum
Several issues with this code. You need to fix them one by one and run it in a tester for debugging. If you don't know how to program, post a job in the
freelance section.
https://www.mql5.com/en/job
- MaxTrades is set to 2 and stays this value, yet only trades will open if it's <2 which is never true. There's a
counter missing to count all open trades, say
OpenTrades, and then instead of MaxTrades<2 you need to place OpenTrades<MaxTrades (although on a 2nd
look I think the code will only work with 1 open trade.)
- Replace the keywords extern, init/deinit and start with input, OnInit/OnDeinit and OnTick.
- The check for OperationsAllowed is wrong when StartHour > LastHour. Should be:
if(StartHour>LastHour && (Hour()>=StartHour || Hour()<=LastHour)) OperationsAllowed=true;
- This belongs into OnInit(). Do not initialize StopLoss together with the global variables.
StopLoss =1.5*iATR(NULL,0,14,0); TakeProfit=iATR(NULL,0,14,0);
There are some more issues but fix this first.
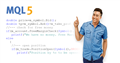
- www.mql5.com
Do not double post
It is selfish of you to waste other people's time
I have deleted your other posts and moved a reply to here.
Do not double post
It is selfish of you to waste other people's time
I have deleted your other posts and moved a reply to here.

- Free trading apps
- Over 8,000 signals for copying
- Economic news for exploring financial markets
You agree to website policy and terms of use