I missed this part in function's docs.
As I see it, another bad decision of MQL5 team.
Why on earth add the terminal 0 character to the string, it's beyond me.
It took me a while to figure out the bug in my code,
but it boils down to StringToShortArray() function
this is the test:
What's going on?
The docs about this function, don't mention anything about the length change
Is this a bug? or is this really the decision of mql5 developers?
The debugger does not seem to allow one to inspect the array variables during runtime (unless I am missing a trick) so I added a printf loop to see what was in your array - there does seem to be a blank position at the end.
When I looked at help for StringToShortArray, I noticed there are extra arguments which specify the start and end of the string - in particular i t:
"count=-1
[in] Number of array elements to copy. Defines length of a resulting string. Default value is -1, which means copying up to the array end, or till terminal 0.Terminal 0 will also be copied to the recipient array, in this case the size of a dynamic array can be increased if necessary to the size of the string. If the size of the dynamic array exceeds the length of the string, the size of the array will not be reduced."
Therefore by specifying this you can avoid the trailing blank(s): StringToShortArray(s, a, 0, StringLen(s));
Here is the code if you wanna play around with it:
//+------------------------------------------------------------------+
void OnStart()
{
//---
test_strings();
}
//+------------------------------------------------------------------+
void test_strings()
{
ushort a[];
int alen = 0;
string s = "michael";
StringToShortArray(s, a, 0, StringLen(s));
printf("string '%s' has len %i array a has len %i", s, StringLen(s), ArraySize(a));
//result: string 'michael' has len 7 array a has len 8
for (int i=0; i < ArraySize(a); i++)
{
printf("a[%d] = %s Ascii Value (dec)= %s", i, CharToStr (a[i]), string (a[i]) );
}
}
thanks for point that out,
i tested your way too,
StringToShortArray(s, a, 0, StringLen(s));
and this way, ArraySize(a) shows 7, as expected
but this style defeats the purpose of what StringToShortArray() should do by default
Programmer should not take care of this detail, if by default, the intuitive way is for a[] to have 7 codes, for each digits
that's why i said: why on earth did they choose to add that 0 or blank at the end?
it's totally unintuitive, but MQL5 style since i am programming in it, they always insist to mess defaults, just because.
"noooo, we cannot follow best practices, we are unique" :D
yeah ok
power to Ctrader, I hope they will take over metatrader someday :D
why on earth did they choose to add that 0 or blank at the end?
- en.wikipedia.org
thanks for point that out,
i tested your way too,
and this way, ArraySize(a) shows 7, as expected
but this style defeats the purpose of what StringToShortArray() should do by default
Programmer should not take care of this detail, if by default, the intuitive way is for a[] to have 7 codes, for each digits
that's why i said: why on earth did they choose to add that 0 or blank at the end?
it's totally unintuitive, but MQL5 style since i am programming in it, they always insist to mess defaults, just because.
"noooo, we cannot follow best practices, we are unique" :D
yeah ok
power to Ctrader, I hope they will take over metatrader someday :D
Yes - every language has it peculiarities.
Some of the worst are the big software companies who ship code which is undocumented or failing... After many frustrating experiences over the years I find it just better to accept the imperfections and find ways to make it work the way you want :)
Metatrader should help traders be better traders, not experts in clang
Newer languages (eg. python, Golang, javascript, etc) make it so much easier in my experience - perhaps one day we will see implementations in newer languages...
The point is that a most dll's require null terminated strings. You'll see plenty of libraries that convert MQL strings to null terminate strings for DLL consumption using these techniques. Example:
https://github.com/dingmaotu/mql4-lib/blob/master/Lang/Native.mqh#L171:L177
void StringToUtf8(const string str,uchar &utf8[],bool ending=true) { if(!ending && str=="") return; int count=ending ? -1 : StringLen(str); StringToCharArray(str,utf8,0,count,CP_UTF8); }
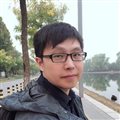
- dingmaotu
- github.com

- Free trading apps
- Over 8,000 signals for copying
- Economic news for exploring financial markets
You agree to website policy and terms of use
It took me a while to figure out the bug in my code,
but it boils down to StringToShortArray() function
this is the test:
What's going on?
The docs about this function, don't mention anything about the length change
Is this a bug? or is this really the decision of mql5 developers?