Hi
I have been trying to modify this bit of sample code for use. I keep getting the Invalid Price error. Does anyone have any ideas
Thanks
This part doesn't make sense, and it's the reason you aren't getting filled. This needs to be a relevant entry price.
double price=1000*point; // unnormalized open price price=NormalizeDouble(price,digits); // normalizing open price
Your pip calculations are also wrong and need to be adjusted for 3|5 digit brokers (all of them). Also, you need to be using volume that's within the parameters of the symbol and also you need to be rounding to the tick-size and not the point. CSymbolInfo will help make your code more readable and provide easier access to the API data.
#include <Trade\Trade.mqh> //+------------------------------------------------------------------+ //| Script program start function | //+------------------------------------------------------------------+ void OnStart() { const int num_pips_entry = 10; const string symbol_to_trade = "GBPUSD"; CSymbolInfo symbol; symbol.Name(symbol_to_trade); symbol.RefreshRates(); double volume = symbol.LotsMin(); double xpip = symbol.Point(); if(symbol.Digits() == 3 || symbol.Digits() == 5) xpip *= 10; double price_entry = round_tick( symbol.Ask() - num_pips_entry * xpip, symbol ); double sl = round_tick(price_entry - 300 * xpip, symbol); double tp = round_tick(price_entry + 500 * xpip, symbol); datetime expiration = TimeCurrent() + PeriodSeconds(PERIOD_D1); int digits = symbol.Digits(); string comment = StringFormat( "Buy Limit %s: %.2f lots @ %s, SL=%s TP=%s", symbol.Name(), volume, DoubleToString(price_entry, digits), DoubleToString(sl, digits), DoubleToString(tp, digits) ); CTrade trade; bool is_success = trade.BuyLimit( volume, price_entry, symbol.Name(), sl, tp, ORDER_TIME_GTC, expiration, comment ); printf("BuyLimit() method %s. Return code=%d. Code description: %s", is_success ? "Successful" : "Failed", trade.ResultRetcode(), trade.ResultRetcodeDescription() ); } //+------------------------------------------------------------------+ double round_tick(double price, CSymbolInfo &symbol) { return round(price / symbol.TickSize()) * symbol.TickSize(); }
This part doesn't make sense, and it's the reason you aren't getting filled. This needs to be a relevant entry price.
Your pip calculations are also wrong and need to be adjusted for 3|5 digit brokers (all of them). Also, you need to be using volume that's within the parameters of the symbol and also you need to be rounding to the tick-size and not the point. CSymbolInfo will help make your code more readable and provide easier access to the API data.
Hi
Just an update in order to eventually get round this problem, i used the following code
#include <Trade\Trade.mqh> CTrade trade; // at the top of the page double askNow =NormalizeDouble(SymbolInfoDouble(_Symbol,SYMBOL_ASK),_Digits); double bidNow =NormalizeDouble(SymbolInfoDouble(_Symbol,SYMBOL_BID),_Digits); if(OrdersTotal()==0 && PositionsTotal()==0) { trade.SellLimit(0.10,(askNow+(200*_Point)),_Symbol,0,(bidNow-(200*_Point)),ORDER_TIME_GTC,0,0); }
I then modified it for my purposes
error 10015 with my sell orders
Hello team members,
I have a grim problem; I have written the function below to send pending orders to broker. Currently I am looking at BTCUSD and I keep getting "error 10015" as a response. I am new to MQL5; I have just been at it at about a week; so getting to understanf=d the language must have compromised by the need to rush to have a useful script ready. Pardon me for that.Any help suggestions w=etc shall be appreciated. Thanks!
// called from inside batch_submit_pending orders()) void MqlTradeRequest_creator(string m_ticket,datetime m_datatstamp,string m_symbol,string m_direction,double m_entryprice,double m_takeprofit,double m_stoploss,double m_lotsize,string order_modifySLTP_or_cancel) { MqlTradeRequest request; MqlTradeResult result; int digits_ = SymbolInfoInteger(m_symbol,SYMBOL_DIGITS); Print("!",SymbolInfoInteger(pair_array[0],SYMBOL_DIGITS)); datetime request_expiry = m_datatstamp + PeriodSeconds(PERIOD_H4); if(order_modifySLTP_or_cancel == "order"){request.action = TRADE_ACTION_PENDING;} if(order_modifySLTP_or_cancel == "modifySLTP"){request.action = TRADE_ACTION_MODIFY;} if(order_modifySLTP_or_cancel == "cancel"){request.action = TRADE_ACTION_REMOVE;} if(m_direction =="LONG"){request.type = ORDER_TYPE_BUY_LIMIT;}else{request.type = ORDER_TYPE_SELL_LIMIT;} long ticket = StringToInteger(StringSubstr(m_ticket,2,15)); request.order = ticket; request.symbol = m_symbol; request.volume = NormalizeDouble(m_lotsize,digits_); request.price = NormalizeDouble(m_entryprice,digits_); request.sl = NormalizeDouble(m_stoploss,digits_); request.tp = NormalizeDouble(m_takeprofit,digits_); request.type_filling = ORDER_FILLING_FOK; request.type_time =ORDER_TIME_SPECIFIED; request.expiration = request_expiry; request.comment = "placed by xapiens_ea"; //Implementing trailing stop-loss double breakeven_trailing_stop_price = 0.0; // activated when price is +0.5% in profit and moves stop-loss to open-price. double above_1pc_trailing_stop_price = 0.0; // takes over from breakeven_trailing_stop_price once profitability hits 1% and trails at 60% of profitability. double dynamic_trailing_stop_price =0.0; // takes over from above_1pc_trailing_stop_price once profitability hits 1.5% and trails at 70% of profitability. double current_price = 0.0; double current_profit=0.0; double current_bid_price = 0.0; double current_ask_price = 0.0; double trailing_stop_price = 0.0; if(m_direction == "LONG"){ breakeven_trailing_stop_price = (1.0+(0.5/100))*m_entryprice; above_1pc_trailing_stop_price = (1.0+(1.0/100))*m_entryprice; dynamic_trailing_stop_price =(1.0 + (1.5/100))*m_entryprice; current_bid_price = SymbolInfoDouble(m_symbol,SYMBOL_BID); current_profit = NormalizeDouble(current_bid_price - m_entryprice,digits_); if( (m_entryprice+current_profit) < breakeven_trailing_stop_price){trailing_stop_price = m_stoploss;}else{ if( (current_profit >0) && ((m_entryprice + current_profit) >= breakeven_trailing_stop_price) && ((m_entryprice + current_profit) < above_1pc_trailing_stop_price) ){trailing_stop_price = breakeven_trailing_stop_price;} if( (m_entryprice + current_profit) > breakeven_trailing_stop_price && (m_entryprice + current_profit) >= above_1pc_trailing_stop_price && (m_entryprice + current_profit) < dynamic_trailing_stop_price ) { trailing_stop_price = above_1pc_trailing_stop_price;} if( (current_profit + m_entryprice) >= dynamic_trailing_stop_price ){trailing_stop_price = dynamic_trailing_stop_price;} }//ENDIF }//LONG if(m_direction == "SHORT"){ breakeven_trailing_stop_price = (1.0 - (0.5/100))*m_entryprice; above_1pc_trailing_stop_price = (1.0 - (1.0/100))*m_entryprice; dynamic_trailing_stop_price = (1.0 - (1.5/100))*m_entryprice; current_ask_price = SymbolInfoDouble(m_symbol,SYMBOL_ASK); current_profit = NormalizeDouble(m_entryprice - current_ask_price,2); if( (m_entryprice - current_profit) > breakeven_trailing_stop_price){trailing_stop_price = m_stoploss;}else{ if( (current_profit >0) && ((m_entryprice - current_profit) <= breakeven_trailing_stop_price) && ((m_entryprice + current_profit) > above_1pc_trailing_stop_price) ){trailing_stop_price = breakeven_trailing_stop_price;} if( (m_entryprice - current_profit) < breakeven_trailing_stop_price && (m_entryprice - current_profit) <= above_1pc_trailing_stop_price && (m_entryprice - current_profit) > dynamic_trailing_stop_price ) {trailing_stop_price = above_1pc_trailing_stop_price;} if( (m_entryprice - current_profit) <= dynamic_trailing_stop_price ){trailing_stop_price = dynamic_trailing_stop_price;} }//ENDIF } request.sl = NormalizeDouble(trailing_stop_price,digits_); Print("|ticket:",ticket,"|dxn:",m_direction,"|symbol:",m_symbol,"|volume:",m_lotsize,"|entryprice:",m_entryprice,"|deviation:",int((0.250/100)*m_entryprice),"|tstamp:",m_datatstamp,"|expiration:",request_expiry,"|trail_stop:",trailing_stop_price,"|current_profit:",current_profit); bool res = OrderSend(request,result); if( (res && result.retcode == TRADE_RETCODE_DONE) || (res && result.retcode == TRADE_RETCODE_PLACED)) { Print("Trade successfully placed"); } else { Print("Trade not placed. Error code ",result.retcode); } } //EOF
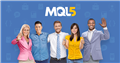

- Free trading apps
- Over 8,000 signals for copying
- Economic news for exploring financial markets
You agree to website policy and terms of use
Hi
I have been trying to modify this bit of sample code for use. I keep getting the Invalid Price error. Does anyone have any ideas
Thanks