Example:
EURUSD price: 1.22375
it40cash price: 23638
these numbers are both double, but the first has a decimal part, the second not.
Is there a way to verify if a number have a decimal part?
Thank's
Piero
You could for example convert it into a string and search for the "."
EDIT : not with strings cause it adds a .0 to an integer. Much more simply actually :
if (MathRound(price)==price) { printf("**** yeeepeeee no digits!"); }
Example:
EURUSD price: 1.22375
it40cash price: 23638
these numbers are both double, but the first has a decimal part, the second not.
Is there a way to verify if a number have a decimal part?
Thank's
Piero
The CDouble library has a function to get the number of Digits.
#include <Double.mqh> void OnStart() { //--- double number = 0.1234567; printf("The number of digits in %.10f is %d", number, CDouble::GetDigits(number)); } //The number of digits in 0.1234567000 is 7
converting with the string I tried yesterday but it does not work as you said.
I was looking for a library like Double.mqh, very good!
Thanks
Piero
Hi
I have a problem with the Double library.
----------------------------------------
bool Verifica(double prezzo, int decimali, int button)
{
//verifica decimali
int lungh2 = CDouble::GetDigits(prezzo);
--------------------------------------
if "prezzo" came from the function "SymbolInfoDouble(Symbol, SYMBOL_BID)" where Symbol is EUSUSD, I have that lungh2 = 16 as result.
But the result would be 5..
Hi
I have a problem with the Double library.
----------------------------------------
bool Verifica(double prezzo, int decimali, int button)
{
//verifica decimali
int lungh2 = CDouble::GetDigits(prezzo);
--------------------------------------
if "prezzo" came from the function "SymbolInfoDouble(Symbol, SYMBOL_BID)" where Symbol is EUSUSD, I have that lungh2 = 16 as result.
But the result would be 5..
You should only be using the tick size for prices.
CDouble eurusd_prices(PRECISION_TICK_SIZE,"EURUSD"); eurusd_prices = SymbolInfoDouble("EURUSD",SYMBOL_BID); double step = eurusd_prices.Step(); int digits = eurusd_prices.Digits();
Great work!!
Thank's
Piero
Example:
EURUSD price: 1.22375
it40cash price: 23638
these numbers are both double, but the first has a decimal part, the second not.
Is there a way to verify if a number have a decimal part?
Thank's
Piero
This is possible to do entirely in MQL too, using the MathMod() function with a divisor of 1:
This is the MathMod() function spec:
double MathMod(
double value, // dividend value
double value2 // divisor value
);
--
Here's a quick little function to test the values you've mentioned:
bool hasDecimal(double num) { if(MathMod(num, 1) != 0) { Print(num + " -> has decimal places."); return(true); } Print(num + " -> does NOT have decimal places."); return(false); }
Results:
hasDecimal(1.22375);
2018.01.25 12:14:48.679 TestBench EURUSD,H1: 1.22375 -> has decimal places.
hasDecimal(23638.0); // With ".0" for sake of completeness.
2018.01.25 12:14:48.679 TestBench EURUSD,H1: 23638 -> does NOT have decimal places.
hasDecimal(23638); // Without any decimal point.
2018.01.25 12:14:48.679 TestBench EURUSD,H1: 23638 -> does NOT have decimal places.
--
Hope this proves useful.
The == operand. - MQL4 and MetaTrader 4 - MQL4 programming forum
bool isInt(double d){ return MathAbs(d - int(d) ) < DBL_EPSILON; } bool hasFraction(double d){ return !isInt(d); }
whroeder1:
I wouldn't use MathMod. There are known problems with it due to floating point rounding.
...
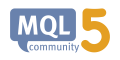
- 2013.02.20
- www.mql5.com
For reference about MathMod issue https://www.mql5.com/en/forum/143605#comment_3627665
Interesting.. the example in the earlier post was a trivial on-the-fly demo, but thank you very much for pointing this issue out with MathMod() - noted!

- Free trading apps
- Over 8,000 signals for copying
- Economic news for exploring financial markets
You agree to website policy and terms of use
Example:
EURUSD price: 1.22375
it40cash price: 23638
these numbers are both double, but the first has a decimal part, the second not.
Is there a way to verify if a number have a decimal part?
Thank's
Piero