? I can only assume that adding the sortCandidate pointer to the method's (original) local "pivots" collection means that "pivots" goes out of scope at the end of the method and somehow, the pointers contained in it are affectedd? However, I thought that this was why pointers are used?
I've been trying to understand this issue for days and have run out of ideas. Any help would be very much appreciated.
---
I see that time passed since your problem was announced here, but I will put my view anyway... such an error do not concern the scope, but really access to pointer (I showed here)... the correct way to get the obj that is behind the pointer outside the scope where the pointer was given the real object is smth like this (use GetPointer())
for (int i=0 ; i<arr.Total(); i++) Print ("pop " , GetPointer (arr)[i].ToString()); // <<<<<<<<< MEMBER-METHOD CALL
though I do not see the whole your code - but I do not need it at all... -- next time you'd better to make short compilable example reflecting the problem - and you will probably get the answer quicker than this time...
good luck
p.s. also see links from here
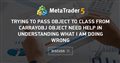
- 2010.11.20
- www.mql5.com
BTW, I also had "Invalid pointer access"- error -- when Copy-Constructor & Assignment operator= were not defined correctly ... and even GetPointer didn't matter...
(do not know the whole your code, but you can check)
p.s.
and besides, creating new object dynamically in class_methods is a BAD design-idea (it increases coupling of classes & possible proException-places in code)... use "new" ONLY in secondary Constructors (like in Java) or primary Constructors (and delete in Destructors)... imho

- Free trading apps
- Over 8,000 signals for copying
- Economic news for exploring financial markets
You agree to website policy and terms of use
This is where items are added:
There are obviously items in the _highPivots collection because it finds a Total() value.
Since I originally posted this message, I modified the following method:
Using _sortPivotCandidates (defined as CObjVector<CPivotCandidate> _sortPivotCandidates), the problem disappears? I can only assume that adding the sortCandidate pointer to the method's (original) local "pivots" collection means that "pivots" goes out of scope at the end of the method and somehow, the pointers contained in it are affectedd? However, I thought that this was why pointers are used?
I've been trying to understand this issue for days and have run out of ideas. Any help would be very much appreciated.
Regards and thanks.