Here is an example of putting function pointers to good use. Those who know javascript or python will likely recognize this pattern immediately.
This is a generic object vector that I frequently use, and I've added a filter method which takes a function as an argument and returns a pointer to a new vector. The new vector is added to a "garbage collector" so there is no need for memory management, however, if the scope of the original (non-filtered) collection runs out before the scope of the filtered vector then there will be an error due to the object getting deleted.
objvector
Use example.
This is rather old, but still I'd like to thank for the good template and reference! :)
nicholish en: Here is an example of putting function pointers to good use. Those who know javascript or python will likely recognize this pattern immediately.
in C++ it (function pointer) is a functor or callback... it is easy way to use a function as a parameter for another function... passing pointer to it (the first) as an argument...
general good explanation was on youtube about typedef Function Pointers in MQL4_ and also here example was and here ...
& thank you for the generalized version to deal with pointers in function (with last being used as function pointer)... though it is still a little bit complicated for me
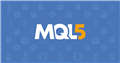
- www.mql5.com
new_vector.FreeMode(false);
but why did you switch-off the CArrayObject's responsibility for freeing the memory ??
I suppose the reason is Lifetime in the scope & the goal is to return new_vector (or dest_arr) out of scope... taking into consideration :
In MQL4 functions can't return objects, but they can return the object pointer.
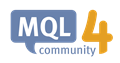
- docs.mql4.com
I finally found out why to use FreeMode(false); else -
The element will not change if an invalid pointer (for example, NULL) is passed as a parameter. If the memory management is enabled, the memory of a replaced element is deallocated.
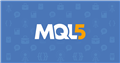
- www.mql5.com

- Free trading apps
- Over 8,000 signals for copying
- Economic news for exploring financial markets
You agree to website policy and terms of use
Here is an example of putting function pointers to good use. Those who know javascript or python will likely recognize this pattern immediately.
This is a generic object vector that I frequently use, and I've added a filter method which takes a function as an argument and returns a pointer to a new vector. The new vector is added to a "garbage collector" so there is no need for memory management, however, if the scope of the original (non-filtered) collection runs out before the scope of the filtered vector then there will be an error due to the object getting deleted.
objvector
Use example.