This doesn't answer your question, but . . . had you considered using Terminal Globals?
https://www.mql5.com/en/docs/globals
If you need the vars sent to a file, then you need it; but if you don't, this may work out for you.
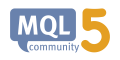
- www.mql5.com
Would you mind taking a look and making suggestions as to why it's not working?
Read the documentation again:
FileReadDouble
Reads a double-precision floating point number (double) from the current position of the binary file.
FileReadNumber
The function reads from the CSV file a string from the current position till a separator (or till the end of a text string) and converts the read string to a value of double type.
Read the documentation again:
Ahh thanks. That makes a lot more sense. It's working a little better now, but still behaving a bit strangely. Here is what I have now.
FileName = StringConcatenate(Symbol(),".txt"); int Vars[8]; int Count = 1; FileHandle = FileOpen(FileName,FILE_CSV|FILE_READ, ","); while (!FileIsEnding(FileHandle) && Count <= 8) { FileSeek(FileHandle,Count,SEEK_SET); Vars[Count] = FileReadNumber(FileHandle); Count++; } Print(Vars[1],Vars[2],Vars[3],Vars[4],Vars[5],Vars[6],Vars[7],Vars[8]);
Now when the program initialises it prints "000255000". Whereas it should print "002500001". I looked at the text file generated by the FileWrite function that I posted earlier, and the problem isn't with that, it's seems that something is wrong with the file pointer? Any ideas?
Oh ok, I didn't realise that it would remember the file position. It's fixed now. Thanks guys!!

- Free trading apps
- Over 8,000 signals for copying
- Economic news for exploring financial markets
You agree to website policy and terms of use
Hi guys,
I wrote this bit of code based on a discussion I found on a different thread. But I can't seem to get it to work. Basically I have an on deinit function that dumps 8 different varaibles as CSV to a file. I know that bit works because I can obviously read the file. Then I also have an on init function that reads those values into an array that I will then use to get them back into the variable slots that they came out of. Trouble is I can't get the file reading to work properly. I'm using FileReadDouble, but I think I'm doing something wrong. Here is the code.
Would you mind taking a look and making suggestions as to why it's not working?
Thanks