The static declaration is ok but I would put it near the start of the function and not within a for loop.
If you want to use OrderOpenPrice() you must select the CORRECT order first.
By correct I mean correct for the current symbol and the current EA (by Magic Number). If OrdersTotal is greater than 1 you need to get the correct Order!
The static declaration is ok but I would put it near the start of the function and not within a for loop.
If you want to use OrderOpenPrice() you must select the CORRECT order first.
By correct I mean correct for the current symbol and the current EA (by Magic Number). If OrdersTotal is greater than 1 you need to get the correct Order!
right, in fact there is only 1 open order by this EA at any time, so no need to flex the i number.
int start()
static double lastHedge;
if (OrderSelect(1, SELECT_BY_POS, MODE_TRADES) && OrderMagicNumber() == Magic.Number && OrderSymbol() == Current.Symbol) lastHedge = OrderOpenPrice();
correct?
right, in fact there is only 1 open order by this EA at any time, so no need to flex the i number.
int start()
static double lastHedge;
if (OrderSelect(1, SELECT_BY_POS, MODE_TRADES) && OrderMagicNumber() == Magic.Number && OrderSymbol() == Current.Symbol) lastHedge = OrderOpenPrice();
correct?
close ...
int Magic.Number= 1234; int start(){ static double lastHedge; if( OrderSelect(0, SELECT_BY_POS, MODE_TRADES) && OrderMagicNumber()== Magic.Number && OrderSymbol() == Symbol() ) lastHedge = OrderOpenPrice(); // any other stuff needed return( 0 ); }
Remember that in C and MQL4 arrays start from 0 not from 1.
The current symbol is held in Symbol()
>right, in fact there is only 1 open order by this EA at any time, so no need to flex the i number.
Actually I missed the subtlety here. If there any other open orders then you still need the for loop
>right, in fact there is only 1 open order by this EA at any time, so no need to flex the i number.
Actually I missed the subtlety here. If there any other open orders then you still need the for loop
hi Dabbler!
thanks a lot for your patience and support!! I will try it out and report back.
- just for my general info.. regarding adding back FOR (int i= etc. etc.), indeed there are other EA's running simultaneously on other charts, but all charts have unique Magic & Symbol combinations. Why will "For" operator still be required ?
Have a great weekend!
D.
Dannoo007:
right, in fact there is only 1 open order by this EA at any time, so no need to flex the i number.
int start()
static double lastHedge;
if (OrderSelect(1, SELECT_BY_POS, MODE_TRADES) && OrderMagicNumber() == Magic.Number && OrderSymbol() == Current.Symbol) lastHedge = OrderOpenPrice();
indeed there are other EA's running simultaneously on other charts, but all charts have unique Magic & Symbol combinations. Why will "For" operator still be required ?
- Only 1 open order until you put the EA on a second chart, or put another EA on a chart, or open a manual trade. When you have 2 open orders, position 1 may be yours or may be the other EAs. Do it right.
static double lastHedge; for(int iPos = OrdersTotal()-1; iPos >= 0 ; iPos--) if ( OrderSelect(iPos, SELECT_BY_POS) // Only my orders w/ && OrderMagicNumber() == Magic.Number // my magic number && OrderSymbol() == chart.symbol // and my pair. ){ lastHedge = OrderOpenPrice(); }
- Remember that a change of pair or timeframe will result in a deinit/init cycle, but global and static variables are NOT reset (as the EA is not reloaded.) When this is important, you must reset them. I use this pattern
int init(){ onInitFN1(); : } type wasStatic; void onInitFN1(){ wasStatic = ...; } type FN1(){ //static type wasStatic = ...; :
I put the variable and its initialization next to the function that uses it.
hi Dabbler!
- just for my general info.. regarding adding back FOR (int i= etc. etc.), indeed there are other EA's running simultaneously on other charts, but all charts have unique Magic & Symbol combinations. Why will "For" operator still be required ?
The thing is that there are multiple positions being held (orders) so you have to search through all of them to find which one belongs to this EA. Since you OrderSelecting by position in a list, and you don't know where the relevant order is in this list, you have to search the whole list. WHR has given the (correct) code for this in his post above.
Many thanks WHRoeder and Dabbler!!
yep, it makes sense!! I was moving in circles inside my head with the wrong logic, now I get it: at each tick the FOR loop will cycle through the ENTIRE LIST OF OPEN orders- not just those generated by the "home EA" of the FOR.
Good point about re-setting variables regularly - I will see how this plays out. I am actually now looking into having separate variables set for buy and sell signals, so that there is no interference (ie, adding OrderType()==OP_BUY/SELL to WHRoeder's script).
Best regards,
Dan.
yep, it makes sense!! I was moving in circles inside my head with the wrong logic, now I get it:
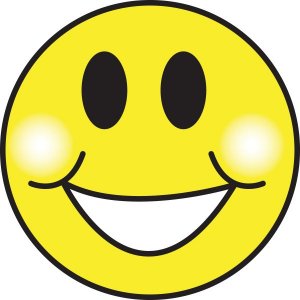

- Free trading apps
- Over 8,000 signals for copying
- Economic news for exploring financial markets
You agree to website policy and terms of use
Hello forum,
Market is closed for testing, but I am looking into creating this static variable, to capture the value of OrderOpenPrice for subsequent use (ie, once the current order is closed by SL, the previous level is kept as reference), for example:
BUY Order A : Open at 10, with SL at 9
Once SL=9 is hit, order is closed, however the static variable remebers opening level of 10
Once Bid < falls below the static variable (ie 10) ---> a new BUY Order B is opened, with the new open price recorded as the new value of the static variable..
etc. Note, maxorders=1 so I will always have only 1 open order.
Do I simply include at start():
int start()
{
static double lastHedge;
lastHedge = OrderOpenPrice();
}
or do I need to select the orders, to avoid interference from other Charts/magic numbers/etc? (I believe it shold be something like below..)
int start()
{
for (int i = OrdersTotal()-1; i >=0; i--) OrderSelect(i, SELECT_BY_POS, MODE_TRADES);
static double lastHedge;
lastHedge = OrderOpenPrice();
}
Cheers!
D.