Quick question. I am trying to write an EA that will record datetime and bid price data at each tick tick, and store this data only for the most recent x number of seconds. (IE the ea uses the tick data from the previous 20 seconds to make decisions on trading.) Since I do not know how many ticks there will be in this amount of time, I thought I would use in the global variables - dynamic arrays CArrayDouble object for the bid prices and CArrayInt object for the datetimes. These dynamic arrays would store data at each new tick and delete tick data that is older than x number of seconds. Is this a good idea? Or will it cause memory leak or something else bad? Here is my code so far:
In my opinion, there is no technical issue with your approach but maybe a logical one. What you need as dynamic are the arrays not the object itself. So you can use :
//---global variables
CArrayDouble bid_array;
CArrayInt seconds_array;
However if you keep pointers, I suggest you the following modification :
int OnInit() { if(CheckPointer(bid_array)==POINTER_INVALID) { Print("Object create error"); return(INIT_FAILED); } //--- if(CheckPointer(seconds_array)==POINTER_INVALID) { Print("Object create error"); return(INIT_FAILED); } ...
Quick question. I am trying to write an EA that will record datetime and bid price data at each tick tick, and store this data only for the most recent x number of seconds. (IE the ea uses the tick data from the previous 20 seconds to make decisions on trading.) Since I do not know how many ticks there will be in this amount of time, I thought I would use in the global variables - dynamic arrays CArrayDouble object for the bid prices and CArrayInt object for the datetimes. These dynamic arrays would store data at each new tick and delete tick data that is older than x number of seconds. Is this a good idea? Or will it cause memory leak or something else bad? Here is my code so far:
Using dynamic arrays will not cause memory leaks, unless MT5 has a bug. It should be handling the dynamic part of the array and controlling the memory allocation.
One slightly disconnected point, you might want to consider how and if you want to record missed ticks . . . you will miss some ticks and you can determine that you have missed ticks, the question is do you want to keep track of that fact in your array ?
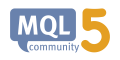
- www.mql5.com
Thanks for the advice guys! Angevogageur - I will use those global variables you recommended. RaptorUK, I am not worried about missed ticks for now. I have another question though, what type of dynamic array should I use to store the datetime of each tick? I tried CArrayInt, and also tried CArrayObj to store MqlDatetime Objects. Neither of theses approaches worked. What would you recommend? Should I convert the datetime from TimeCurrent() to a string, store at in CArrayString, then reconvert each element back to a datetime using StringToTime() when I need to work with each element?
Thanks for the advice guys! Angevogageur - I will use those global variables you recommended. RaptorUK, I am not worried about missed ticks for now. I have another question though, what type of dynamic array should I use to store the datetime of each tick? I tried CArrayInt, and also tried CArrayObj to store MqlDatetime Objects. Neither of theses approaches worked. What would you recommend? Should I convert the datetime from TimeCurrent() to a string, store at in CArrayString, then reconvert each element back to a datetime using StringToTime() when I need to work with each element?
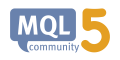
- www.mql5.com
There is no class for datetime array, as datetime is actually an int. So you can normally use CArrayInt. Or follow RaptorUk advice, don't use a class, use datetime array and resize it yourself.
Hi, are you sure CArrayInt is conviniet, if Int has 4 bytes and datetime is of 8 bytes? Or is it necessary first to structure datetime some how?
Hi, are you sure CArrayInt is conviniet, if Int has 4 bytes and datetime is of 8 bytes? Or is it necessary first to structure datetime some how?
You are answering to a more than 6 years post. In the meantime a lot has changed (datetime was 4 bytes at that time).
With 4 bytes a datetime can manage date up to 2038, up to you to see if you can live with that or not. If not use CArrayLong.
You are answering to a more than 6 years post. In the meantime a lot has changed (datetime was 4 bytes at that time).
With 4 bytes a datetime can manage date up to 2038, up to you to see if you can live with that or not. If not use CArrayLong.

- Free trading apps
- Over 8,000 signals for copying
- Economic news for exploring financial markets
You agree to website policy and terms of use
Quick question. I am trying to write an EA that will record datetime and bid price data at each tick tick, and store this data only for the most recent x number of seconds. (IE the ea uses the tick data from the previous 20 seconds to make decisions on trading.) Since I do not know how many ticks there will be in this amount of time, I thought I would use in the global variables - dynamic arrays CArrayDouble object for the bid prices and CArrayInt object for the datetimes. These dynamic arrays would store data at each new tick and delete tick data that is older than x number of seconds. Is this a good idea? Or will it cause memory leak or something else bad? Here is my code so far: