[...] all this things suggest that THERE IS a multiple close! But how i can do this in programatically (in MQL4 language) by a EA?
You do use OrderCloseBy() in order to do a multiple-close. However, this is less than simple. You can only pair off two orders at a time (which creates a new order for any difference between them in lot size). To do a multiple close, you have to make repeated calls to OrderCloseBy(). But the speed shouldn't matter enormously; once your buys and sells net out to zero, your cash position should only be affected by movements in the spread, not movements in the price. Therefore, it generally won't matter (significantly) whether the closure is near-instantaneous as it is in the user interface, or somewhat slower in the automated version using repeated calls to OrderCloseBy().
However, the "close by" is only supported by some brokers. On the others, the user interface option is not available, and the OrderCloseBy() function does not work.
Is there any way to close all buy/sell trades at once?
There is a "Multiple Close By" feature in N2Trader which can close all buys/sells alternatively until all trades be closed or just one trade (which there are no pair trade for closing it) remains:
At first, i thought N2Trader uses the "Close By" method iteratively to close all trades. but it seems it's not the case, because:
- N2Trader do this significantly fast
- as shown below, the log distinguish between "close by" and multiple close".
all this things suggest that THERE IS a multiple close! But how i can do this in programatically (in MQL4 language) by a EA?
The log of a "Close by" order for two trades (a buy is closed by a sell):
The log of a "Multiple close" order:
for (int cnt = OrdersTotal()-1 ; cnt >= 0; cnt--)
{
OrderSelect(cnt,SELECT_BY_POS,MODE_TRADES);
if (OrderType()==OP_SELL&&OrderSymbol() == Symbol())
{
if(OrderMagicNumber() == MagicN)
{
OrderClose(OrderTicket(),OrderLots(),OrderClosePrice(),Digits),10,CLR_NONE);
Sleep(100);
}
}
}
Example for Close All Sell from an EA [...]
isaacarsenal is specifically asking about closing orders by pairing them off against each other, rather than simply closing each individual order separately - i.e. using OrderCloseBy() rather than OrderClose(). On brokers who support it, this usually saves money (spread) and executes faster.
I know the OrderCloseBy() function which close pairs of orders. But it cannot close all orders at once.
Is there any function which closes all trades at once?
I know the OrderCloseBy() function which close pairs of orders. But it cannot close all orders at once.
Is there any function which closes all trades at once?
No. As explained in my earlier response.
No. As explained in my earlier response.
Can someone please help me with / point me to a pre-written piece of code that I can use to achieve multiple close by ?
sat
Can someone please help me with / point me to a pre-written piece of code that I can use to achieve multiple close by ?
I know this is an old thread but I'm posting this here.
I re-wrote the code slightly
// MQL4&5-code // #include <MT4Orders.mqh> // https://www.mql5.com/en/code/16006 #property strict string CorrectSymbol( string &Symb ) { return(Symb = ((Symb == NULL) || (Symb == "")) ? _Symbol: Symb); } bool PlaceHedge( const string Symb ) { double Lots = 0; for (int i = OrdersTotal() - 1; i >= 0; i--) if (OrderSelect(i, SELECT_BY_POS) && (OrderType() <= OP_SELL) && (OrderSymbol() == Symb)) Lots += OrderType() ? OrderLots() : -OrderLots(); Lots = NormalizeDouble(Lots, 2); return(((Lots > 0) && (OrderSend(Symb, OP_BUY, Lots, SymbolInfoDouble(Symb, SYMBOL_ASK), 100, 0, 0, "HEDGE") > 0)) || ((Lots < 0) && (OrderSend(Symb, OP_SELL, -Lots, SymbolInfoDouble(Symb, SYMBOL_BID), 100, 0, 0, "HEDGE") > 0)) || !Lots); } bool CloseAll( string Symb = NULL ) { int Type = 0; long Ticket = 0; bool Res = PlaceHedge(CorrectSymbol(Symb)); for (int i = OrdersTotal() - 1; !IsStopped() && Res && (i >= 0); i--) if (OrderSelect(i, SELECT_BY_POS) && (OrderType() <= OP_SELL) && (OrderSymbol() == Symb)) { if (!Ticket) { Ticket = OrderTicket(); Type = OrderType(); } else if ((OrderType() != Type) && (Res = OrderCloseBy(Ticket, OrderTicket()) && PlaceHedge(Symb))) { Ticket = 0; i = OrdersTotal(); } } return(Res); } void OnStart() { CloseAll(); }
I re-wrote the code slightly
Hi fxsaber,
Thanks for your feedback, I'm a big fan of your work... I like the simplicity of your alterations. I know that my example looks to be over complicated on the surface, however, I designed it that way so that it could close all open orders/positions in the following way for maximum risk mitigation.
Operation Summary:
- Net positions are calculated per symbol (sum of all open trades).
- Symbols are prioritized to manage net-positions from larger (more risk) to smaller.
- Hedge orders are sent in the order of priority to close all exposure at once regardless of the number of trades.
- Once all open-exposure is hedged the program then prioritizes pending orders
- Pending orders are deleted in order of highest risk of being executed
- Remaining hedged positions (closed by hedge) are then reconciled on the broker server using CloseBy()

- Free trading apps
- Over 8,000 signals for copying
- Economic news for exploring financial markets
You agree to website policy and terms of use
Is there any way to close all buy/sell trades at once?
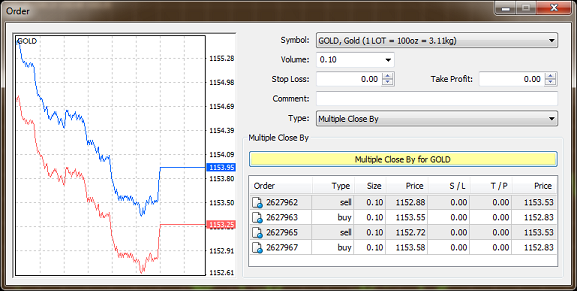
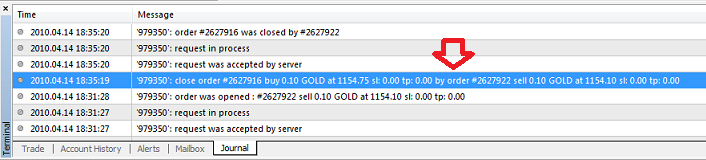
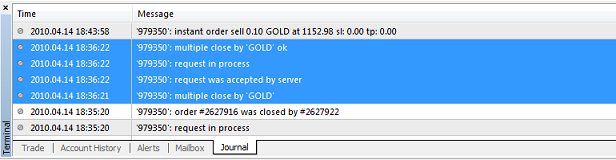
There is a "Multiple Close By" feature in N2Trader which can close all buys/sells alternatively until all trades be closed or just one trade (which there are no pair trade for closing it) remains:
At first, i thought N2Trader uses the "Close By" method iteratively to close all trades. but it seems it's not the case, because:
- N2Trader do this significantly fast
- as shown below, the log distinguish between "close by" and multiple close".
all this things suggest that THERE IS a multiple close! But how i can do this in programatically (in MQL4 language) by a EA?
The log of a "Close by" order for two trades (a buy is closed by a sell):
The log of a "Multiple close" order: