int OpenOrders=0; MoneyProfit_Need = 150;//$$$ MoneyProfit_Summary =0; for(cnt=0;cnt<=OrdersTotal()-1;cnt++) { if (OrderSelect(cnt, SELECT_BY_POS, MODE_TRADES)) { if (OrderSymbol()==Symbol()) { if (OrderType()==OP_BUY || OrderType()==OP_SELL) { MoneyProfit_Summary = MoneyProfit_Summary+OrderProfit(); } OpenOrders++; } } } if (OpenOrders>0 && MoneyProfit_Need!=0 && MoneyProfit_Summary>= MoneyProfit_Need) { CloseOrders(............); }
I have an EA that may open and close several trades during a Trading session.
If the Equity increases by $150, then I want to close any open orders and stop/exit the EA from any further Trading.
Thank you.
Here you are:
extern double MyProfit=150.0; extern bool CanTrade=true; int init() { GlobalVariableSet("OldBalance",AccountBalance()); return(0); } int deinit() { return(0); } int start() { if (AccountEquity()>=GlobalVariableGet("OldBalance")+MyProfit) { CloseOrders(Magic); // see earlier post CanTrade=false; } GlobalVariableSet("OldBalance",AccountBalance()); if (CanTrade==true) { //... order opening ... }
You can change the CanTrade variable to TRUE manually, if you want new trades.
Cheers
&&
if(AccountEquity()-AccountBalance()>=150) CloseOrder(.....);
I would prefer an explanation on how to incorporate this into an EA i use being that i have one that trades with in a couple strategies.
Here is an example of an EA that i start off making mine out of. I understand this one more then other.
extern int MagicNumber = 0;
extern bool EachTickMode = False;
extern double Lots = 0.1;
extern int Slippage = 3;
extern bool StopLossMode = True;
extern int StopLoss = 100;
extern bool TakeProfitMode = True;
extern int TakeProfit = 100;
extern bool TrailingStopMode = True;
extern int TrailingStop = 30;
extern double MaximumRisk =0.15;
extern double DecreaseFactor =3;
extern int MaxOrders = 1;
#define SIGNAL_NONE 0
#define SIGNAL_BUY 1
#define SIGNAL_SELL 2
#define SIGNAL_CLOSEBUY 3
#define SIGNAL_CLOSESELL 4
int BarCount;
int Current;
bool TickCheck = False;
//+------------------------------------------------------------------+
//| expert initialization function |
//+------------------------------------------------------------------+
int init() {
BarCount = Bars;
if (EachTickMode) Current = 0; else Current = 1;
return(0);
}
//+------------------------------------------------------------------+
//| expert deinitialization function |
//+------------------------------------------------------------------+
int deinit() {
return(0);
}
//+------------------------------------------------------------------+
//| expert start function |
//+------------------------------------------------------------------+
int start() {
int Order = SIGNAL_NONE;
int Total, Ticket;
double StopLossLevel, TakeProfitLevel;
int digit = MarketInfo(Symbol(),MODE_DIGITS);
if (EachTickMode && Bars != BarCount) EachTickMode = False;
Total = OrdersTotal();
Order = SIGNAL_NONE;
//+------------------------------------------------------------------+
//| Signal Begin |
//+------------------------------------------------------------------+
double Buy1_1 = iMA(NULL, 0, 55, 0, MODE_EMA, PRICE_CLOSE, Current + 1);
double Buy1_2 = iMA(NULL, 0, 200, 0, MODE_EMA, PRICE_CLOSE, Current + 1);
double Buy2_1 = iMA(NULL, 0, 55, 0, MODE_EMA, PRICE_CLOSE, Current + 0);
double Buy2_2 = iMA(NULL, 0, 200, 0, MODE_EMA, PRICE_CLOSE, Current + 0);
double Buy3_1 = iSAR(NULL, 0, 0.005, 0.05, Current + 1);
double Buy3_2 = iSAR(NULL, 0, 0.005, 0.05, Current + 0);
double Buy4_1 = iMACD(NULL, 0, 12, 26, 9, PRICE_CLOSE, MODE_SIGNAL, Current + 1);
double Buy4_2 = iMACD(NULL, 0, 12, 26, 9, PRICE_CLOSE, MODE_SIGNAL, Current + 0);
double Sell1_1 = iMA(NULL, 0, 55, 0, MODE_EMA, PRICE_CLOSE, Current + 1);
double Sell1_2 = iMA(NULL, 0, 200, 0, MODE_EMA, PRICE_CLOSE, Current + 1);
double Sell2_1 = iMA(NULL, 0, 55, 0, MODE_EMA, PRICE_CLOSE, Current + 0);
double Sell2_2 = iMA(NULL, 0, 200, 0, MODE_EMA, PRICE_CLOSE, Current + 0);
double Sell3_1 = iSAR(NULL, 0, 0.005, 0.05, Current + 1);
double Sell3_2 = iSAR(NULL, 0, 0.005, 0.05, Current + 0);
double Sell4_1 = iMACD(NULL, 0, 12, 26, 9, PRICE_CLOSE, MODE_SIGNAL, Current + 1);
double Sell4_2 = iMACD(NULL, 0, 12, 26, 9, PRICE_CLOSE, MODE_SIGNAL, Current + 0);
if (Buy1_1 < Buy1_2 && Buy2_1 >= Buy2_2 && Buy3_1 < Buy3_2 && Buy4_1 < Buy4_2) Order = SIGNAL_BUY;
if (Sell1_1 > Sell1_2 && Sell2_1 <= Sell2_2 && Sell3_1 > Sell3_2 && Sell4_1 > Sell4_2) Order = SIGNAL_SELL;
//+------------------------------------------------------------------+
//| Signal End |
//+------------------------------------------------------------------+
//Buy
if (Order == SIGNAL_BUY && ((EachTickMode && !TickCheck) || (!EachTickMode && (Bars != BarCount)))) {
if(ScanTrades() < MaxOrders) {
//Check free margin
if (AccountFreeMargin() < (1000 * Lots)) {
Print("We have no money. Free Margin = ", AccountFreeMargin());
return(0);
}
StopLossLevel = Ask - StopLoss * Point;
TakeProfitLevel = Ask + TakeProfit * Point;
Ticket = OrderSend(Symbol(), OP_BUY, LotsOptimized(),
NormalizeDouble(Ask,digit),
Slippage,
NormalizeDouble(StopLossLevel,digit),
NormalizeDouble(TakeProfitLevel,digit),
"Buy(#" + MagicNumber + ")", MagicNumber, 0, DodgerBlue);
if(Ticket > 0) {
if (OrderSelect(Ticket, SELECT_BY_TICKET, MODE_TRADES)) Print("BUY order opened : ", OrderOpenPrice()); else Print("Error opening BUY order : ", GetLastError());
}
if (EachTickMode) TickCheck = True;
if (!EachTickMode) BarCount = Bars;
return(0);
}
}
//Sell
if (Order == SIGNAL_SELL && ((EachTickMode && !TickCheck) || (!EachTickMode && (Bars != BarCount)))) {
if(ScanTrades() < MaxOrders) {
//Check free margin
if (AccountFreeMargin() < (1000 * Lots)) {
Print("We have no money. Free Margin = ", AccountFreeMargin());
return(0);
}
StopLossLevel = Bid + StopLoss * Point;
TakeProfitLevel = Bid - TakeProfit * Point;
Ticket = OrderSend(Symbol(), OP_SELL, LotsOptimized(),
NormalizeDouble(Bid,digit),
Slippage,
NormalizeDouble(StopLossLevel,digit),
NormalizeDouble(TakeProfitLevel,digit),
"Sell(#" + MagicNumber + ")", MagicNumber, 0, DeepPink);
if(Ticket > 0) {
if (OrderSelect(Ticket, SELECT_BY_TICKET, MODE_TRADES)) Print("SELL order opened : ", OrderOpenPrice()); else Print("Error opening SELL order : ", GetLastError());
}
if (EachTickMode) TickCheck = True;
if (!EachTickMode) BarCount = Bars;
return(0);
}
}
//Check position
for (int i = 0; i < Total; i ++) {
OrderSelect(i, SELECT_BY_POS, MODE_TRADES);
if(OrderType() <= OP_SELL && OrderSymbol() == Symbol()) {
if(OrderType() == OP_BUY && OrderMagicNumber()==MagicNumber ) {
//Close
if (Order == SIGNAL_CLOSEBUY && ((EachTickMode && !TickCheck) || (!EachTickMode && (Bars != BarCount)))) {
OrderClose(OrderTicket(), OrderLots(), Bid, Slippage, MediumSeaGreen);
if (EachTickMode) TickCheck = True;
if (!EachTickMode) BarCount = Bars;
return(0);
}
//Trailing stop
if(TrailingStopMode && TrailingStop > 0) {
if(Bid - OrderOpenPrice() > Point * TrailingStop) {
if(OrderStopLoss() < Bid - Point * TrailingStop) {
OrderModify(OrderTicket(), OrderOpenPrice(), Bid - Point * TrailingStop, OrderTakeProfit(), 0, MediumSeaGreen);
if (!EachTickMode) BarCount = Bars;
return(0);
}
}
}
} else {
if(OrderType()==OP_SELL && OrderMagicNumber()==MagicNumber)
{
//Close
if (Order == SIGNAL_CLOSESELL && ((EachTickMode && !TickCheck) || (!EachTickMode && (Bars != BarCount))))
{
OrderClose(OrderTicket(), OrderLots(), Ask, Slippage, DarkOrange);
if (EachTickMode) TickCheck = True;
if (!EachTickMode) BarCount = Bars;
return(0);
}
//Trailing stop
if (TrailingStopMode && TrailingStop > 0)
{
if((OrderOpenPrice() - Ask) > (Point * TrailingStop)) {
if((OrderStopLoss() > (Ask + Point * TrailingStop)) || (OrderStopLoss() == 0)) {
OrderModify(OrderTicket(), OrderOpenPrice(), Ask + Point * TrailingStop, OrderTakeProfit(), 0, DarkOrange);
if (!EachTickMode) BarCount = Bars;
return(0);
}
}
}
}}
}
}
if (!EachTickMode) BarCount = Bars;
return(0);
}
int ScanTrades()
{
int total = OrdersTotal();
int numords = 0;
for(int cnt=0; cnt<total; cnt++)
{
OrderSelect(cnt, SELECT_BY_POS);
if(OrderSymbol() == Symbol() && OrderType()<=OP_SELLSTOP && OrderMagicNumber() == MagicNumber)
numords++;
}
return(numords);
}
//bool ExistPositions() {
// for (int i=0; i<OrdersTotal(); i++) {
// if (OrderSelect(i, SELECT_BY_POS, MODE_TRADES)) {
// if (OrderSymbol()==Symbol() && OrderMagicNumber()==MagicNumber) {
// return(True);
// }
// }
// }
// return(false);
//}
double LotsOptimized()
{
double lot=Lots;
int orders=HistoryTotal(); // history orders total
int losses=0; // number of losses orders without a break
//---- select lot size
if(MaximumRisk>0)lot=NormalizeDouble(Lots*AccountFreeMargin()*MaximumRisk/1000.0,1);
//---- calcuulate number of losses orders without a break
if(DecreaseFactor>0)
{
for(int i=orders-1;i>=0;i--)
{
if(OrderSelect(i,SELECT_BY_POS,MODE_HISTORY)==false) { Print("Error in history!"); break; }
if(OrderSymbol()!=Symbol() || OrderType()>OP_SELL) continue;
//----
if(OrderProfit()>0) break;
if(OrderProfit()<0) losses++;
}
if(losses>1) lot=NormalizeDouble(lot-lot*losses/DecreaseFactor,1);
}
//---- return lot size
if(lot<0.1) lot=0.1;
return(lot);
}
//+------------------------------------
How would i get it into this EA?
int start { ........... if(AccountEquity()-AccountBalance()>=150) ClosePosFirstProfit(); ........... } //+----------------------------------------------------------------------------+ //| Автор : Ким Игорь В. aka KimIV, http://www.kimiv.ru | //+----------------------------------------------------------------------------+ //| Версия : 19.02.2008 | //| Описание: Закрытие одной предварительно выбранной позиции | //+----------------------------------------------------------------------------+ void ClosePosBySelect() { bool fc; color clClose; double ll, pa, pb, pp; int err, it; if (OrderType()==OP_BUY || OrderType()==OP_SELL) { for (it=1; it<=NumberOfTry; it++) { if (!IsTesting() && (!IsExpertEnabled() || IsStopped())) break; while (!IsTradeAllowed()) Sleep(5000); RefreshRates(); pa=MarketInfo(OrderSymbol(), MODE_ASK); pb=MarketInfo(OrderSymbol(), MODE_BID); if (OrderType()==OP_BUY) { pp=pb; clClose=clCloseBuy; } else { pp=pa; clClose=clCloseSell; } ll=OrderLots(); fc=OrderClose(OrderTicket(), ll, pp, Slippage, clClose); if (fc) { if (UseSound) PlaySound(NameFileSound); break; } else { err=GetLastError(); if (err==146) while (IsTradeContextBusy()) Sleep(1000*11); Print("Error(",err,") Close ",GetNameOP(OrderType())," ", ErrorDescription(err),", try ",it); Print(OrderTicket()," Ask=",pa," Bid=",pb," pp=",pp); Print("sy=",OrderSymbol()," ll=",ll," sl=",OrderStopLoss(), " tp=",OrderTakeProfit()," mn=",OrderMagicNumber()); Sleep(1000*5); } } } else Print("Некорректная торговая операция. Close ",GetNameOP(OrderType())); } //+----------------------------------------------------------------------------+ //| Автор : Ким Игорь В. aka KimIV, http://www.kimiv.ru | //+----------------------------------------------------------------------------+ //| Версия : 19.02.2008 | //| Описание : Закрытие позиций по рыночной цене сначала прибыльных | //+----------------------------------------------------------------------------+ //| Параметры: | //| sy - наименование инструмента ("" - любой символ, | //| NULL - текущий символ) | //| op - операция (-1 - любая позиция) | //| mn - MagicNumber (-1 - любой магик) | //+----------------------------------------------------------------------------+ void ClosePosFirstProfit(string sy="", int op=-1, int mn=-1) { int i, k=OrdersTotal(); if (sy=="0") sy=Symbol(); // Сначала закрываем прибыльные позиции for (i=k-1; i>=0; i--) { if (OrderSelect(i, SELECT_BY_POS, MODE_TRADES)) { if ((OrderSymbol()==sy || sy=="") && (op<0 || OrderType()==op)) { if (OrderType()==OP_BUY || OrderType()==OP_SELL) { if (mn<0 || OrderMagicNumber()==mn) { if (OrderProfit()+OrderSwap()>0) ClosePosBySelect(); } } } } } // Потом все остальные k=OrdersTotal(); for (i=k-1; i>=0; i--) { if (OrderSelect(i, SELECT_BY_POS, MODE_TRADES)) { if ((OrderSymbol()==sy || sy=="") && (op<0 || OrderType()==op)) { if (OrderType()==OP_BUY || OrderType()==OP_SELL) { if (mn<0 || OrderMagicNumber()==mn) ClosePosBySelect(); } } } } }
I have an EA that may open and close several trades during a Trading session.
If the Equity increases by $150, then I want to close any open orders and stop/exit the EA from any further Trading.
Thank you.
This one has a graphical user interface (GUI) on chart window.
There are also trailing stop and break even, applied on equity.
Equity stops can be repeated or one-time.
http://xyzo-trade-robots.de/index.php/en/products/equity-win
They charge 200 EUR (ca. 260 USD) for it.
--------
Snyxol
I recently found and tested succesfully such an EA, "Xyzo Equity Win" from Xyzo Software, closing all trades on an account when equity reaches a threshold (equity stop loss, equity take profit).
This one has a graphical user interface (GUI) on chart window.
There are also trailing stop and break even, applied on equity.
Equity stops can be repeated or one-time.
http://xyzo-trade-robots.dum/index.php/en/products/equity-win
They charge 200 EUR (ca. 260 USD) for it.
--------
Snyxol
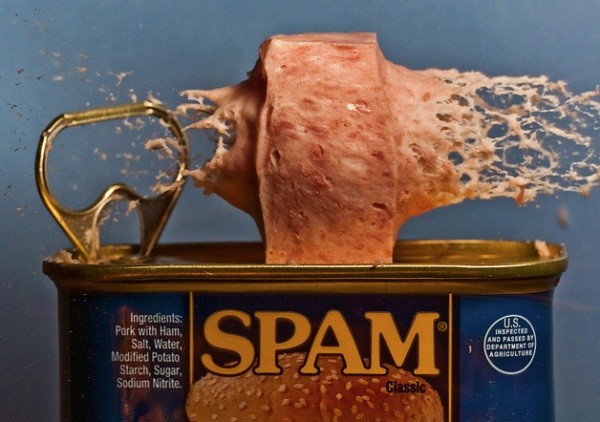

- Free trading apps
- Over 8,000 signals for copying
- Economic news for exploring financial markets
You agree to website policy and terms of use
I have an EA that may open and close several trades during a Trading session.
If the Equity increases by $150, then I want to close any open orders and stop/exit the EA from any further Trading.
Thank you.