MQL5. Помогите разобраться, как выделить сигнал на вход в сделку по первой появившейся синей гистограмме (для лонга) и красной(для шорта).
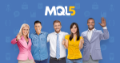
Открой новые возможности в MetaTrader 5 с сообществом и сервисами MQL5
- 599.00 USD
- 2024.04.29
- www.mql5.com
MQL5: язык торговых стратегий для MetaTrader 5, позволяет писать собственные торговые роботы, технические индикаторы, скрипты и библиотеки функций

Вы упускаете торговые возможности:
- Бесплатные приложения для трейдинга
- 8 000+ сигналов для копирования
- Экономические новости для анализа финансовых рынков
Регистрация
Вход
Вы принимаете политику сайта и условия использования
Если у вас нет учетной записи, зарегистрируйтесь