double iStochMain() { double sumLow=0.0,sumHigh=0.0; for(int u=0;u<slowing;u++) { sumLow+=(iClose(Symbol(),5,u+1)-iLow(Symbol(),5,iLowest(Symbol(),5,MODE_LOW,perck,u+1))); sumHigh+=(iHigh(Symbol(),5,iHighest(Symbol(),5,MODE_HIGH,perck,u+1))-iLow(Symbol(),5,iLowest(Symbol(),5,MODE_LOW,perck,u+1))); } return(sumLow/sumHigh*100); }
Thank you so much! Appreciate the help of a fellow Saffer!
Ok so now I have the correct values for the %K line:
double SK1(int tf) { int starts=iTime(Symbol(),PERIOD_H4,0); int barstart=iBarShift(Symbol(),1,iTime(Symbol(),PERIOD_H4,0)); double rem=barstart%tf; int theshift=tf-rem-1; double sumLow=0.0,sumHigh=0.0; ArrayResize(K1,percd+1); int t; for(int i=0;i<(percd+1)*tf;i+=tf) { for(int u=0;u<slowing*tf;u+=tf) { sumLow+=(iClose(Symbol(),1,(u+tf-theshift)+i))-iLow(Symbol(),1,iLowest(Symbol(),1,MODE_LOW,perck*tf,(u+tf-theshift)+i)); sumHigh+=(iHigh(Symbol(),1,iHighest(Symbol(),1,MODE_HIGH,perck*tf,(u+tf-theshift)+i))-iLow(Symbol(),1,iLowest(Symbol(),1,MODE_LOW,perck*tf,(u+tf-theshift)+i))); } K1[t]=sumLow/sumHigh*100; sumLow=0.0; sumHigh=0.0; t++; } return(K1[0]); }
Adjust for a custom time frame value
How I want to go about calculating th %D - using an EMA.
From what I understand, the iMAOnArray() will only work for indicator buffers, for which this is not.
An SMA for %D would be simply:
double theema; theema=(K1[0]+K1[1]+K1[2]+K1[3]+K1[4])/5;
How would I go about calculating %D using EMA?
Regards
Richard
iMAOnArray() should work or you can use the function from MovingAverages.mqh header file.
MMm Thanks Ernst, but does not seem to be producing the correct value?
MMm Thanks Ernst, but does not seem to be producing the correct value
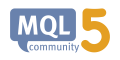
These array sizes get the correct values.
#include <MovingAverages.mqh> double Stoch[2]; //+------------------------------------------------------------------+ //| Custom indicator iteration function | //+------------------------------------------------------------------+ void OnTick() { //--- iStochastic(_Symbol,_Period,7,3,3,Stoch); printf("Stochastic = %.3f Signal = %.3f",Stoch[0],Stoch[1]); //--- return value of prev_calculated for next call return; } //+------------------------------------------------------------------+ int iStochastic(string symbol,int timeframe,int k_period,int d_period,int slowing,double &stoch[]) { double Kbuffer[],Dbuffer[]; ArrayResize(Kbuffer,k_period*3); ArrayResize(Dbuffer,k_period*3); //--- for(int i=0;i<k_period*3;i++) { double sumLow=0.0,sumHigh=0.0; for(int u=i;u<i+slowing;u++) { sumLow+=(iClose(symbol,timeframe,u)-iLow(symbol,timeframe,iLowest(Symbol(),timeframe,MODE_LOW,k_period,u))); sumHigh+=(iHigh(symbol,timeframe,iHighest(symbol,timeframe,MODE_HIGH,k_period,u))-iLow(symbol,timeframe,iLowest(symbol,timeframe,MODE_LOW,k_period,u))); } Kbuffer[i]=sumLow/sumHigh*100; } //--- for(int i=k_period*3-2;i>=0;i--) Dbuffer[i]=ExponentialMA(i,d_period,Dbuffer[i+1],Kbuffer); //--- stoch[0]=Kbuffer[0]; stoch[1]=Dbuffer[0]; //--- return(0); } //+------------------------------------------------------------------+
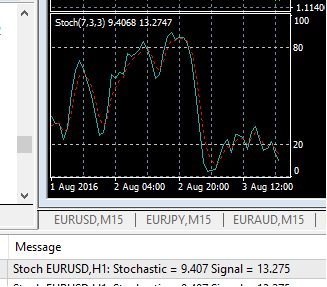
These array sizes get the correct values.
Awesome thanks Ernst! Will give it a try.
Thanks for the help man!
These array sizes get the correct values.
You rock Ernst! Works like a charm.
Thanks Buddy!

- Free trading apps
- Over 8,000 signals for copying
- Economic news for exploring financial markets
You agree to website policy and terms of use
Hi there,
I am trying to code an indicator to show Stochastic %K and %D for custom timeframes ( 2, 17, 26 etc).
For the first step, I am just trying to recode the normal stochastic, to give me the %K for the most recent closed bar. for this example I am using the M5 timeframe. My code is as follows:
Where "slowing" is the slowing period for the stochastic, and perck is the %K period.
The value is close to the standard MT4 stochastic indi, but out but a couple of decimal places.
Any idea where I am going wrong?
Thanks
Richard