What do you mean by ...
MarketInfo(Symbol(), 14)
???
You must use NormalizeDouble(price level for SL, _Digits) for SL's and TP's.
Also, if you open a Sell order, you must be sure SL is higher than Ask + Minimum Stoplevel. If you open a Buy order, SL must be lower than Bid - Minimum Stoplevel.
Regards.
It's a simple EA which should do nothing but open a trade and set the SL to the stoplevel + 2 pips
Sorry if the code is ugly, it's my first day coding in MQL
It's opening the trades successfully but then not modifying the SL
It's always throwing error 130. Stoplevel is 40 points with my broker
Hi Vandi,
I thought you should not always use "OrderModify" to place your SL at first time opening position.
You can do "OrderModify" later, after your position in surplus to lock current position with SL breakeven before hit t/p.
Because we can put SL at at the same time when opening position.
I write a simple code for you :
//--- input parameters extern int MagicNo=0;//Magic number extern double Lot=0.01;//Lot extern int TP=50;//Target (how many pips range between currrent price to next Support as 1st target) extern int SL=10;//Stop loss (how many pips above current Resistance) extern int Slip=3;//Slippage extern string Tradelog="<your strategy>";//Trade log int ticket=0, error, Dgt; //+------------------------------------------------------------------+ //| Expert initialization function | //+------------------------------------------------------------------+ int OnInit() { if (Digits==4) Dgt=1; else if (Digits==5||Digits==2||Digits==3) Dgt=10; return(INIT_SUCCEEDED); } //+------------------------------------------------------------------+ //| Expert tick function | //+------------------------------------------------------------------+ void OnTick() { //--- //--- <A> variables and criteria of your strategy //--- To increase CPU performance and to prevent repainting: //--- I suggest you are better only once at time last candle ended //--- for iBands and iStochastic, not at every tick :) if( ...<A>... ) { Print("--- opening Sell "); while (true) { if(SL>0) ticket=OrderSend(Symbol(),OP_SELL,Lot,Bid,Slip,NormalizeDouble(Bid+SL*Dgt*Point,Digits), NormalizeDouble(Bid-TP*Dgt*Point,Digits),Tradelog,MagicNo,0,clrRed); else if(SL==0) ticket=OrderSend(Symbol(),OP_SELL,Lot,Bid,Slip,0, NormalizeDouble(Bid-TP*Dgt*Point,Digits),Tradelog,MagicNo,0,clrRed); if(ticket<=0) { error=GetLastError(); if(error>0) {Print("--- Please wait until Sell opened."); RefreshRates();} // prices changed break; } else { Print("+++ Position Sell is now opened."); break; } //---- 3 seconds wait Sleep(3000); } // --> while true } }
Good luck,
Yohana
Hi Vandi,
I thought you should not always use "OrderModify" to place your SL at first time opening position.
You can do "OrderModify" later, after your position in surplus to lock current position with SL breakeven before hit t/p.
Because we can put SL at at the same time when opening position.
I write a simple code for you :
Good luck,
Yohana
Yes but this does not work for ECN broker so you have to use OrderSend() to open order with 0 tp and 0 sl, and then after it opened used OrderModify() to set tp and sl values.
Problem is clearly in the MarketInfo()
Print("Open " + OrderOpenPrice() + " SL " + (Bid+20*Point+Point*MarketInfo(Symbol(), 14))); int changed=OrderModify(ticket,0,Bid+20*Point+Point*MarketInfo(Symbol(), 14),0,0,Green);
Like Osama pointed out above.
It's a simple EA which should do nothing but open a trade and set the SL to the stoplevel + 2 pips
Sorry if the code is ugly, it's my first day coding in MQL
It's opening the trades successfully but then not modifying the SL
It's always throwing error 130. Stoplevel is 40 points with my broker
I think you should use also the price of your order like below :
int changed=OrderModify(ticket,OrderOpenPrice(),Bid+20*Point+Point*MarketInfo(Symbol(), 14),0,0,Green);
For whom that asked the MarketInfo function it is for getting stoplevel number.
Stop level may be 'floating'.
extern double StopLoss=20; int OnInit() { double minStop=MarketInfo(NULL,MODE_STOPLEVEL); StopLoss+=minStop; StopLoss*=_Point; return(INIT_SUCCEEDED); } void OnTick(void) { double bbUp=iBands(NULL,0,20,0,2,PRICE_CLOSE,MODE_UPPER,1); double bbLow = iBands(NULL,0,20,0,2,PRICE_CLOSE,MODE_LOWER, 1); double stoch = iStochastic(NULL,0,5,3,3,MODE_SMA,0,1,0); int ticket; if(Bid>bbUp && stoch>80) { ticket=OrderSend(Symbol(),OP_SELL,0.01,Bid,30,0,0,"test",1337,0,Green); if(ticket>0) { if(OrderSelect(ticket,SELECT_BY_TICKET,MODE_TRADES)) { Print("SELL order opened : ",OrderOpenPrice()); Print("Open " + (string)OrderOpenPrice() + " SL " + string(Bid+StopLoss)); bool changed=OrderModify(ticket,0,Bid+StopLoss,0,0,Green); if(!changed) Print("Error"); } } else { Print("Error opening SELL order : ",GetLastError()); } } }
MODE_STOPLEVEL | 14 | Stop level in points
A zero value of MODE_STOPLEVEL means either absence of any restrictions on the minimal distance for Stop Loss/Take Profit or the fact that a trade server utilizes some external mechanisms for dynamic level control, which cannot be translated in the client terminal. In the second case, GetLastError() can return error 130, because MODE_STOPLEVEL is actually "floating" here. |
It's clearly in the description too.
https://docs.mql4.com/constants/environment_state/marketinfoconstants
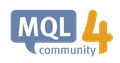
- docs.mql4.com

- Free trading apps
- Over 8,000 signals for copying
- Economic news for exploring financial markets
You agree to website policy and terms of use
It's a simple EA which should do nothing but open a trade and set the SL to the stoplevel + 2 pips
Sorry if the code is ugly, it's my first day coding in MQL
It's opening the trades successfully but then not modifying the SL
It's always throwing error 130. Stoplevel is 40 points with my broker