I have a simple price action EA. I think the "business logic" works fine, from what I've seen in the tester, but I think there is a problem with the creation and modification of orders. This is for example the Buy function:
void Buy(string comm)
{
double op=0,sl=0,tp=0;
op = NormalizeDouble(Ask,Digits);
//CalcLot();
if (AccountFreeMarginCheck(Symbol(),OP_BUY,Lots)<=0 || GetLastError()==134)
{
Print("Νε δξρςΰςξχνξ ρβξαξδνϋυ ρπεδρςβ δλ ξςκπϋςθ ξπδεπΰ BUY ξαϊεμξμ: " + DoubleToStr(Lots,2));
return;
}
Print("Βϋρςΰβλεμ ξπδεπ BUY (v=" + DoubleToStr(Lots, 2) +"), ρ οΰπΰμεςπΰμθ: OP=" + DoubleToStr(op,Digits) +"; SL=" + DoubleToStr(sl,Digits) + "; TP=" + DoubleToStr(tp,Digits));
int Ticket = OrderSend(Symbol(), OP_BUY, Lots, op, Slippage, 0, 0, comm, Magic, 0 ,Blue);
if (PrintError(357)==0)
{
if (OrderSelect( Ticket, SELECT_BY_TICKET)==false) return;
op = NormalizeDouble(OrderOpenPrice(),Digits);
tp = NormalizeDouble(op + (TakeProfit)*Point,Digits);
if (TakeProfit==0) tp=0;
sl = NormalizeDouble(op - (StopLoss)*Point,Digits);
if (StopLoss==0) sl=0;
if (sl!=0 || tp!=0)
{
Print("Μξδθτθφθπσεμ βϋρςΰβλεννϋι ξπδεπ BUY #" + IntegerToString(Ticket));
if (OrderModify( Ticket, op, sl, tp, 0, Yellow)==false)
PrintError(369);
}
}
}
This is with the following default tp and sl:
extern int TakeProfit=30;
extern int StopLoss=15;
A little information, I am using this EA with Pepperstone, who say they are an ECN broker, with 5 digits. I think the way this code is written it caters for the ECN part, meaning I think that everything up to the Ordersend call which sets stop loss and take profit to 0, works. So the EA is able to initiate orders. My problem is that, from what it seems, the OrderModify doesn't work. This means that any order that is made, there is no stop loss or take profit (I think OrderModify is responsible for this). Unfortunately I don't remember whether I saw the error 369 on the Experts tab, so something else might be the problem too. I am setting stop loss to 8 pips and take profit to 2.5, but it doesn't work. By the way, Pepperstone has stop_level 0 so I think that I can set this stop loss and tp with them. Anyone have any idea what might be wrong?
Update: It seems I am getting the Error 130: Invalid stops. Can someone please help with this?
The problem lay on the following line of code:
int Ticket = OrderSend(Symbol(), OP_BUY, Lots, op, Slippage, 0, 0, comm, Magic, 0 ,Blue);
it should be:
int Ticket = OrderSend(Symbol(), OP_BUY, Lots, op, Slippage, NULL, NULL, comm, Magic, 0 ,Blue);
problem solved!
The problem lay on the following line of code:
int Ticket = OrderSend(Symbol(), OP_BUY, Lots, op, Slippage, 0, 0, comm, Magic, 0 ,Blue);
it should be:
int Ticket = OrderSend(Symbol(), OP_BUY, Lots, op, Slippage, NULL, NULL, comm, Magic, 0 ,Blue);
problem solved!
No, you should not be using "NULL", instead of 0 for a "double". The OP's usage of "0" is correct.
Update: It seems I am getting the Error 130: Invalid stops. Can someone please help with this?
When you get an error you should be specific about where the error is occurring. In this case, it is most probably with the OrderModify() line, since that is where you are setting the stops.
However, your code does not show any signs of you checking for the Brokers Stops Level conditions. Also, please do not use NormalizeDouble, but instead use proper price alignment to the Tick Size.
Here is quote from another user which clarifies things:
Forum on trading, automated trading systems and testing trading strategies
MT4 Stop Loss and Take Profit formula
whroeder1, 2017.09.11 02:40
Risk depends on your initial stop loss, lot size, and the value of the pair.- You place the stop where it needs to be - where the reason for the trade is no longer valid. E.g. trading a support bounce the stop goes below the support.
- Account Balance * percent/100 = RISK = OrderLots * (|OrderOpenPrice - OrderStopLoss| * DeltaPerLot + CommissionPerLot) (Note OOP-OSL includes the SPREAD, and DeltaPerLot is usually around $10/pip but it takes account of the exchange rates of the pair vs. your account currency.)
- Do NOT use TickValue by itself - DeltaPerLot
- You must normalize lots properly and check against min and max.
- You must also check FreeMargin to avoid stop out
Forum on trading, automated trading systems and testing trading strategies
Start time lag when using TimeCurrent or market watch feed
whroeder1, 2017.07.19 16:48
|
|
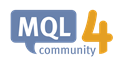
- book.mql4.com
Already answered! The use of "0" or "0.0" is the correct way to define an order without a S/L or T/P! Also:
Void Type and NULL Constant
Syntactically the void type is a fundamental type along with types of char, uchar, bool, short, ushort, int, uint, color, long, ulong, datetime, float, double and string. This type is used either to indicate that the function does not return any value, or as a function parameter it denotes the absence of parameters.
The predefined constant variable NULL is of the void type. It can be assigned to variables of any other fundamental types without conversion. The comparison of fundamental type variables with the NULL value is allowed.
Chris Lazarius: ... could you please type it for us, how to calculate ST or TP?Let say TP = 60 and SL = 75
When you get an error you should be specific about where the error is occurring. In this case, it is most probably with the OrderModify() line, since that is where you are setting the stops.
However, your code does not show any signs of you checking for the Brokers Stops Level conditions. Also, please do not use NormalizeDouble, but instead use proper price alignment to the Tick Size.
Here is quote form another user which clarifies things:
Thanks much, it make sense

- Free trading apps
- Over 8,000 signals for copying
- Economic news for exploring financial markets
You agree to website policy and terms of use
I have a simple price action EA. I think the "business logic" works fine, from what I've seen in the tester, but I think there is a problem with the creation and modification of orders. This is for example the Buy function:
void Buy(string comm)
{
double op=0,sl=0,tp=0;
op = NormalizeDouble(Ask,Digits);
//CalcLot();
if (AccountFreeMarginCheck(Symbol(),OP_BUY,Lots)<=0 || GetLastError()==134)
{
Print("Νε δξρςΰςξχνξ ρβξαξδνϋυ ρπεδρςβ δλ ξςκπϋςθ ξπδεπΰ BUY ξαϊεμξμ: " + DoubleToStr(Lots,2));
return;
}
Print("Βϋρςΰβλεμ ξπδεπ BUY (v=" + DoubleToStr(Lots, 2) +"), ρ οΰπΰμεςπΰμθ: OP=" + DoubleToStr(op,Digits) +"; SL=" + DoubleToStr(sl,Digits) + "; TP=" + DoubleToStr(tp,Digits));
int Ticket = OrderSend(Symbol(), OP_BUY, Lots, op, Slippage, 0, 0, comm, Magic, 0 ,Blue);
if (PrintError(357)==0)
{
if (OrderSelect( Ticket, SELECT_BY_TICKET)==false) return;
op = NormalizeDouble(OrderOpenPrice(),Digits);
tp = NormalizeDouble(op + (TakeProfit)*Point,Digits);
if (TakeProfit==0) tp=0;
sl = NormalizeDouble(op - (StopLoss)*Point,Digits);
if (StopLoss==0) sl=0;
if (sl!=0 || tp!=0)
{
Print("Μξδθτθφθπσεμ βϋρςΰβλεννϋι ξπδεπ BUY #" + IntegerToString(Ticket));
if (OrderModify( Ticket, op, sl, tp, 0, Yellow)==false)
PrintError(369);
}
}
}
This is with the following default tp and sl:
extern int TakeProfit=30;
extern int StopLoss=15;
A little information, I am using this EA with Pepperstone, who say they are an ECN broker, with 5 digits. I think the way this code is written it caters for the ECN part, meaning I think that everything up to the Ordersend call which sets stop loss and take profit to 0, works. So the EA is able to initiate orders. My problem is that, from what it seems, the OrderModify doesn't work. This means that any order that is made, there is no stop loss or take profit (I think OrderModify is responsible for this). Unfortunately I don't remember whether I saw the error 369 on the Experts tab, so something else might be the problem too. I am setting stop loss to 8 pips and take profit to 2.5, but it doesn't work. By the way, Pepperstone has stop_level 0 so I think that I can set this stop loss and tp with them. Anyone have any idea what might be wrong?
Update: It seems I am getting the Error 130: Invalid stops. Can someone please help with this?