Hi!
I have some problem with this indicator (https://www.mql5.com/en/code/442) and I try to remake it in a simple way.
All work ok, but the signal value is always 1.
Some help?
Are you sure that it is correct? I compiled above code and nothing is drawn in the indicator windows - neither Lime ot Yellow line.
The main line is correct (lime line).
If you comment the signal ( put // in front of the code and compile),
ExponentialMAOnBuffer(rates_total,prev_calculated,begin,SmoothPeriod,Ext1Buffer,Ext2Buffer);
You can see the lime line.
The Signal line always give value of 1, it is the cause of error, that I do not know how to solve yet.
Signal is just a EMA of the main line.
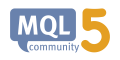
- www.mql5.com
The main line is correct (lime line).
If you comment the signal ( put // in front of the code and compile),
You can see the lime line.
The Signal line always give value of 1, it is the cause of error, that I do not know how to solve yet.
Signal is just a EMA of the main line.
I don't see any of the lines, just blank indicator window.
o.0
Try this code (with this you can see ONLY the main line):
//+------------------------------------------------------------------+ //| Center of Gravity2.mq5 | //| Bruno Pio | //| http://www.mql5.com | //+------------------------------------------------------------------+ #property copyright "Bruno Pio" #property link "http://www.mql5.com" #property version "1.00" #property description "Center of Gravity2" #include <MovingAverages.mqh> //--- indicator settings #property indicator_separate_window #property indicator_buffers 4 #property indicator_plots 2 #property indicator_type1 DRAW_LINE #property indicator_color1 Lime #property indicator_label1 "Center of Gravity" #property indicator_width1 1 #property indicator_style1 STYLE_SOLID #property indicator_type2 DRAW_LINE #property indicator_color2 Yellow #property indicator_label2 "Signal Line" #property indicator_width2 1 #property indicator_style2 STYLE_DASHDOTDOT //--- input parameters input int MA_Period=10; // Indicator averaging period input int SmoothPeriod=3; // Signal line smoothing period //input ENUM_MA_METHOD MA_Method =MODE_EMA; // Signal line averaging method input ENUM_APPLIED_PRICE MA_Price = PRICE_CLOSE; // Price constant //--- indicator buffers double Ext1Buffer[]; double Ext2Buffer[]; double ima_buffer1[]; double ima_buffer2[]; int ima_handle1; int ima_handle2; //+------------------------------------------------------------------+ //| Custom indicator initialization function | //+------------------------------------------------------------------+ int OnInit() { //--- indicator buffers mapping //--- indicator buffers mapping SetIndexBuffer(0,Ext1Buffer,INDICATOR_DATA); PlotIndexSetString(0,PLOT_LABEL,"Center of Gravity"); PlotIndexSetInteger(0,PLOT_DRAW_BEGIN,MA_Period); SetIndexBuffer(1,Ext2Buffer,INDICATOR_DATA); PlotIndexSetString(1,PLOT_LABEL,"Signal Line"); PlotIndexSetInteger(1,PLOT_DRAW_BEGIN,MA_Period); SetIndexBuffer(2,ima_buffer1,INDICATOR_CALCULATIONS); SetIndexBuffer(3,ima_buffer2,INDICATOR_CALCULATIONS); //---- initializations of variable for indicator short name string shortname; StringConcatenate(shortname,"Center of Gravity(",MA_Period,")"); //---- creating name for displaying in a separate sub-window and in a tooltip IndicatorSetString(INDICATOR_SHORTNAME,shortname); //---- set accuracy of displaying of the indicator values IndicatorSetInteger(INDICATOR_DIGITS,0); //---- ima_handle1 = iMA(NULL, 0, MA_Period, 0, MODE_EMA, MA_Price); ima_handle2 = iMA(NULL, 0, MA_Period, 0, MODE_LWMA, MA_Price); return(0); } //+------------------------------------------------------------------+ //| Custom indicator iteration function | //+------------------------------------------------------------------+ int OnCalculate(const int rates_total, const int prev_calculated, const int begin, const double &price[]) { //--- int i,limit; if(prev_calculated==0) limit=0; else limit=prev_calculated-1; //--- check for bars count if(rates_total<=MA_Period) return(0); // not enough bars for calculation ResetLastError(); if (CopyBuffer(ima_handle1,0,0,rates_total,ima_buffer1) <= 0) { Print (GetLastError()); return(0); } ResetLastError(); if (CopyBuffer(ima_handle2,0,0,rates_total,ima_buffer2) <= 0) { Print (GetLastError()); return(0); } for(i = limit; i >= 0; i--) { Ext1Buffer[i] = (ima_buffer1[i]*ima_buffer2[i])/Point(); } //ExponentialMAOnBuffer(rates_total,prev_calculated,begin,SmoothPeriod,Ext1Buffer,Ext2Buffer); //CalculateEMA(rates_total,prev_calculated,begin,Ext1Buffer); //--- return value of prev_calculated for next call return(rates_total); } //+------------------------------------------------------------------+ /* //+------------------------------------------------------------------+ //| exponential moving average | //+------------------------------------------------------------------+ void CalculateEMA(int rates_total,int prev_calculated,int begin,const double &price[]) { int i,limit; double SmoothFactor=2.0/(1.0+SmoothPeriod); //--- first calculation or number of bars was changed if(prev_calculated==0) { limit=SmoothPeriod+begin; Ext2Buffer[begin]=price[begin]; for(i=begin+1;i<limit;i++) Ext2Buffer[i]=price[i]*SmoothFactor+Ext2Buffer[i-1]*(1.0-SmoothFactor); } else limit=prev_calculated-1; //--- main loop for(i=limit;i<rates_total && !IsStopped();i++) Ext2Buffer[i]=price[i]*SmoothFactor+Ext2Buffer[i-1]*(1.0-SmoothFactor); //--- } */
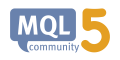
- www.mql5.com
Just an update (but has an error at last bar?):
//+------------------------------------------------------------------+ //| Center of Gravity2.mq5 | //| Bruno Pio | //| http://www.mql5.com | //+------------------------------------------------------------------+ #property copyright "Bruno Pio" #property link "http://www.mql5.com" #property version "1.00" #property description "Center of Gravity2" #include <MovingAverages.mqh> //--- indicator settings #property indicator_separate_window #property indicator_buffers 4 #property indicator_plots 2 #property indicator_type1 DRAW_LINE #property indicator_color1 Yellow #property indicator_label1 "Center of Gravity" #property indicator_width1 1 #property indicator_style1 STYLE_SOLID #property indicator_type2 DRAW_LINE #property indicator_color2 Red #property indicator_label2 "Signal Line" #property indicator_width2 1 #property indicator_style2 STYLE_SOLID //--- input parameters input int MA_Period=10; // Indicator averaging period input int SmoothPeriod=3; // Signal line smoothing period //input ENUM_MA_METHOD MA_Method =MODE_EMA; // Signal line averaging method input ENUM_APPLIED_PRICE MA_Price = PRICE_CLOSE; // Price constant //--- indicator buffers double Ext1Buffer[]; double Ext2Buffer[]; double ima_buffer1[]; double ima_buffer2[]; int ima_handle1; int ima_handle2; //+------------------------------------------------------------------+ //| Custom indicator initialization function | //+------------------------------------------------------------------+ int OnInit() { //--- indicator buffers mapping //--- indicator buffers mapping SetIndexBuffer(0,Ext1Buffer,INDICATOR_DATA); PlotIndexSetString(0,PLOT_LABEL,"Center of Gravity"); PlotIndexSetInteger(0,PLOT_DRAW_BEGIN,MA_Period); SetIndexBuffer(1,Ext2Buffer,INDICATOR_DATA); PlotIndexSetString(1,PLOT_LABEL,"Signal Line"); PlotIndexSetInteger(1,PLOT_DRAW_BEGIN,MA_Period); SetIndexBuffer(2,ima_buffer1,INDICATOR_CALCULATIONS); SetIndexBuffer(3,ima_buffer2,INDICATOR_CALCULATIONS); //---- initializations of variable for indicator short name string shortname; StringConcatenate(shortname,"Center of Gravity(",MA_Period,")"); //---- creating name for displaying in a separate sub-window and in a tooltip IndicatorSetString(INDICATOR_SHORTNAME,shortname); //---- set accuracy of displaying of the indicator values IndicatorSetInteger(INDICATOR_DIGITS,0); //---- ima_handle1 = iMA(NULL, 0, MA_Period, 0, MODE_EMA, MA_Price); ima_handle2 = iMA(NULL, 0, MA_Period, 0, MODE_LWMA, MA_Price); return(0); } //+------------------------------------------------------------------+ //| Custom indicator iteration function | //+------------------------------------------------------------------+ int OnCalculate(const int rates_total, const int prev_calculated, const int begin, const double &price[]) { //--- int i,limit; if(prev_calculated==0) limit=0; else limit=prev_calculated-1; //--- check for bars count if(rates_total<=MA_Period) return(0); // not enough bars for calculation ResetLastError(); if (CopyBuffer(ima_handle1,0,0,rates_total,ima_buffer1) <= 0) { Print (GetLastError()); return(0); } ResetLastError(); if (CopyBuffer(ima_handle2,0,0,rates_total,ima_buffer2) <= 0) { Print (GetLastError()); return(0); } for(i = limit; i >= 0; i--) { Ext1Buffer[i] = (ima_buffer1[i]*ima_buffer2[i])/Point(); } double weight=2.0/(1.0+SmoothPeriod); double ema1; for(i = limit; i >= 0; i--) { ema1 = weight*Ext1Buffer[i] + (1.0-weight)*ema1; Ext2Buffer[i] = ema1;//Ext1Buffer[i]*weight+Ext2Buffer[i+1]*(1.0-weight); } //ExponentialMAOnBuffer(rates_total,prev_calculated,0,SmoothPeriod,Ext1Buffer,Ext2Buffer); //CalculateEMA(rates_total,prev_calculated,0,Ext1Buffer); //--- return value of prev_calculated for next call return(rates_total); } //+------------------------------------------------------------------+ /* //+------------------------------------------------------------------+ //| exponential moving average | //+------------------------------------------------------------------+ void CalculateEMA(int rates_total,int prev_calculated,int begin,const double &price[]) { int i,limit; double SmoothFactor=2.0/(1.0+SmoothPeriod); //--- first calculation or number of bars was changed if(prev_calculated==0) { limit=SmoothPeriod+begin; Ext2Buffer[begin]=price[begin]; for(i=begin+1;i<limit;i++) Ext2Buffer[i]=price[i]*SmoothFactor+Ext2Buffer[i-1]*(1.0-SmoothFactor); } else limit=prev_calculated-1; //--- main loop for(i=limit;i<rates_total && !IsStopped();i++) Ext2Buffer[i]=price[i]*SmoothFactor+Ext2Buffer[i-1]*(1.0-SmoothFactor); //--- } */

- Free trading apps
- Over 8,000 signals for copying
- Economic news for exploring financial markets
You agree to website policy and terms of use
Hi!
I have some problem with this indicator (https://www.mql5.com/en/code/442) and I try to remake it in a simple way.
All work ok, but the signal value is always 1.
Some help?