You may find this topic useful: https://www.mql5.com/en/forum/473812
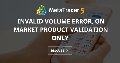
Invalid volume error, On Market product validation ONLY - I keep getting the same error when I submit my program to the market?
- 2024.09.27
- Omega J Msigwa
- www.mql5.com
Error however, this time when i submit it the program to the market, the validation fails due to invalid volume. Thanks, your suggestion and code works locally but, when i submit the program again i get the exact same error. I keep on getting the same error, still

You are missing trading opportunities:
- Free trading apps
- Over 8,000 signals for copying
- Economic news for exploring financial markets
Registration
Log in
You agree to website policy and terms of use
If you do not have an account, please register
Hi, i recently started coding using mql5, and i'm making my first steps. I was developing this expert advisor. It was nothing serious (i didn't even finish it), it was just an attempt to understend how simple "buy" methods work. Anyway, i keep getting stuck when i actually have to send the order, because if i try it on graphic ( i tried it on the COCACOLA one and on the EURUSD) it gives me back an error of "Invalid Volume", and i can't figure out why it does. Can someone please help me? This is the code. I wrote it mostly in italian, so if you need some translation ask me. Tnks.