i am guessing that this is created by ChatGPT or other AI? These codes are often removed by moderators because theses generators create crap code. I only read first quarter of the code and i see 5 lines that are wrong or missing stuff. There is NO fixing this code. Too many issues.
It is not Generated by AI.
There is no warning or issues with code.
it just doesn't take any positions while backtesting.
you don't have to say it to be deleted if you don't know to how ot fix it
Сoders are working for free:
- if it is interesting for them personally, or
- if it is interesting for many members on this forum.
Freelance section of the forum should be used in most of the cases.
NOTE: some parts in your code are common for AI-generated, so it can be hardly helped (would need considerable human review/corrections before being usable in a live trading environment).
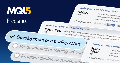
- 2025.02.18
- www.mql5.com
-
If there is no issue with the code, why did you post?
-
Use the debugger or print out your variables, including _LastError and prices and find out why. Do you really expect us to debug your code for you?
Code debugging - Developing programs - MetaEditor Help
Error Handling and Logging in MQL5 - MQL5 Articles (2015)
Tracing, Debugging and Structural Analysis of Source Code - MQL5 Articles (2011)
Introduction to MQL5: How to write simple Expert Advisor and Custom Indicator - MQL5 Articles (2010)
Please consider which section is most appropriate — https://www.mql5.com/en/forum/172166/page6#comment_49114893

- Free trading apps
- Over 8,000 signals for copying
- Economic news for exploring financial markets
You agree to website policy and terms of use