I have found that placing one Object within OnInit() exponentially improves the loading of all other Objects created within a custom function or anywhere below OnInit().
Up top in the global scope:
Inside OnInit():
And after all other Objects have been created:
This is rather simple code. Obviously, you could just create a blank or minimal object with even less code.
My question is... Why does one Object in OnInit() have this positive effect? Is it "initializing" the list of Objects in the terminal in a special way that creation on-the-fly does not?
I'm unable to find the reason behind this in the doc's.
The objects works on two main functions
1. Creation
2. Move, Change of properties
OnInit function is only called once, so you can use ObjectCreate on OnInit for best performance
Object only need to be created once, and based on your logics you can move it using ObjectMove function
for example on the birth of a new bar, you can move object to new bar and also change its properties.
Creating Object everytime on new bar is a bad practice that is why it is recommended to use on OnInit and has positive effect on performance of program.
I have found that placing one Object within OnInit() exponentially improves the loading of all other Objects created within a custom function or anywhere below OnInit().
Up top in the global scope:
Inside OnInit():
And after all other Objects have been created:
This is rather simple code. Obviously, you could just create a blank or minimal object with even less code.
My question is... Why does one Object in OnInit() have this positive effect? Is it "initializing" the list of Objects in the terminal in a special way that creation on-the-fly does not?
I'm unable to find the reason behind this in the doc's.
The objects works on two main functions
1. Creation
2. Move, Change of properties
OnInit function is only called once, so you can use ObjectCreate on OnInit for best performance
Object only need to be created once, and based on your logics you can move it using ObjectMove function
for example on the birth of a new bar, you can move object to new bar and also change its properties.
Creating Object everytime on new bar is a bad practice that is why it is recommended to use on OnInit and has positive effect on performance of program.
Thanks, Rajesh. I will replace my temporary Object in OnInit() with zeroed out Trendline Objects, and then update those Objects from within my custom functions.
EDIT: I will also have a look at Polyline().
Polyline(int&,int&,const uint) - CCanvas - Custom Graphics - Standard Library - MQL5 Reference - Reference on algorithmic/automated trading language for MetaTrader 5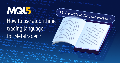
- www.mql5.com
I have found
it is faster . weird
#define SYSTEM_TAG "test_" #property version "1.00" input bool createInit=false;//create init object input int amount=1000;//amount of objects int OnInit() { ObjectsDeleteAll(0,SYSTEM_TAG); ObjectDelete(0,"Initial_Object"); if(createInit){ ObjectCreate(0, "Initial_Object", OBJ_LABEL, 0, 0, 0); ObjectSetInteger(0, "Initial_Object", OBJPROP_CORNER, CORNER_RIGHT_UPPER); ObjectSetInteger(0, "Initial_Object", OBJPROP_ANCHOR, ANCHOR_RIGHT_UPPER); ObjectSetInteger(0, "Initial_Object", OBJPROP_XDISTANCE, 70); ObjectSetInteger(0, "Initial_Object", OBJPROP_YDISTANCE, 120); ObjectSetString(0, "Initial_Object", OBJPROP_TEXT, "LOADING"); ObjectSetString(0, "Initial_Object", OBJPROP_FONT, "Arial"); ObjectSetInteger(0, "Initial_Object", OBJPROP_FONTSIZE, 12); ObjectSetInteger(0, "Initial_Object", OBJPROP_COLOR, clrYellow); } EventSetMillisecondTimer(66); return(INIT_SUCCEEDED); } void OnTimer() { EventKillTimer(); //create more objects and log time int scx=(int)ChartGetInteger(0,CHART_WIDTH_IN_PIXELS,0); int scy=(int)ChartGetInteger(0,CHART_HEIGHT_IN_PIXELS,0); int max_color=16777215; int max_random=32767*32767; color colors[]; int px[],py[],sx[],sy[]; ArrayResize(colors,amount,0); ArrayResize(px,amount,0); ArrayResize(py,amount,0); ArrayResize(sx,amount,0); ArrayResize(sy,amount,0); for(int r=0;r<amount;r++){ double pct=((double)MathRand()*MathRand())/((double)max_random); colors[r]=(color)MathFloor(((double)max_color)*pct); px[r]=(int)MathFloor(((double)scx)*(((double)MathRand())/32767.0)); py[r]=(int)MathFloor(((double)scy)*(((double)MathRand())/32767.0)); sx[r]=(int)MathFloor((200.0)*(((double)MathRand())/32767.0)); sy[r]=(int)MathFloor((200.0)*(((double)MathRand())/32767.0)); } long start_time=GetTickCount(); for(int x=0;x<amount;x++){ string name=SYSTEM_TAG+IntegerToString(x); ObjectCreate(0,name,OBJ_RECTANGLE_LABEL,0,0,0); ObjectSetInteger(0,name,OBJPROP_BGCOLOR,colors[x]); ObjectSetInteger(0,name,OBJPROP_XDISTANCE,px[x]); ObjectSetInteger(0,name,OBJPROP_YDISTANCE,py[x]); ObjectSetInteger(0,name,OBJPROP_XSIZE,sx[x]); ObjectSetInteger(0,name,OBJPROP_YSIZE,sy[x]); } ChartRedraw(true); while(!customObjectFind(SYSTEM_TAG+IntegerToString(amount-1))){ } long end_time=GetTickCount(); long ms=end_time-start_time; if(end_time<start_time){ ms=(end_time+UINT_MAX)-start_time; } Print("Draw time "+IntegerToString(ms)+"ms"); } void OnDeinit(const int reason) { ObjectsDeleteAll(0,SYSTEM_TAG); ObjectDelete(0,"Initial_Object"); } void OnTick() { } bool customObjectFind(string name){ int objs=ObjectsTotal(0,0,OBJ_RECTANGLE_LABEL); for(int i=0;i<objs;i++){ if(ObjectName(0,i,0,OBJ_RECTANGLE_LABEL)==name){return(true);} } return(false); }
Thank you for confirming this by way of your own code testing.
As Alain said, it's not clear what's going on. I rather agree. That's the point of my OP... a weird positive benefit.
Just a quick update here...
My Objects aren't created on every tick because they're created only on the blue box level changes in my Post #3.
In lieu of creating all Objects in OnInit() or using Polyline(), I adapted Thank-god Avwerosuoghene Odukudu's code which works flawlessly.
I'm guessing that other traders may need this:
void DrawLine2(string name, color Clr, datetime t1, double p1, datetime t2, double p2) { //--- Searching if the object (Line in this case) is already created in the chart, if not, create it if(ChartWindowFind(0, "MACD") == 1 && !IsStopped()) ObjectCreate(0, name, OBJ_TREND, 1, t1, p1, t2, p2); //--- Set the properties of the said object(line). ObjectSetInteger(0, name, OBJPROP_COLOR, Clr); ObjectSetInteger(0, name, OBJPROP_STYLE, STYLE_SOLID); ObjectSetInteger(0, name, OBJPROP_WIDTH, 1); }
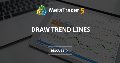
- 2021.04.30
- Rabia Yoruk
- www.mql5.com

- Free trading apps
- Over 8,000 signals for copying
- Economic news for exploring financial markets
You agree to website policy and terms of use
I have found that placing one Object within OnInit() exponentially improves the loading of all other Objects created within a custom function or anywhere below OnInit().
Up top in the global scope:
bool initialized;
Inside OnInit():
And after all other Objects have been created:
This is rather simple code. Obviously, you could just create a blank or minimal object with even less code.
My question is... Why does one Object in OnInit() have this positive effect? Is it "initializing" the list of Objects in the terminal in a special way that creation on-the-fly does not?
I'm unable to find the reason behind this in the doc's.