- number of pips on completed trades
- How to get high and low value from an custom indicator in code?
- Help with inserting indicator into EA
Please consider which section is most appropriate — https://www.mql5.com/en/forum/172166/page6#comment_49114893
There is no need to convert or incorporate an indicator's code into an EA. To use a custom indicator in an EA, simply use the iCustom() function to call on it.
If you are already doing that, then show your code in your EA that calls it so that we can help. Remember to show all relevant code.
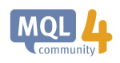
- docs.mql4.com
Don't try to do that. There are no buffers, no IndicatorCounted() or prev_calculated in EAs. No way to know if older bars have changed or been added (history update.)
Just get the value(s) of the indicator(s) into EA/indicator (using iCustom) and do what you want with it.
(MT4) Detailed explanation of iCustom - MQL4 programming forum (2017)
You should encapsulate your custom indicator iCustom calls to make your code self-documenting:
take candle color Hekin Ashi - MQL4 and MetaTrader 4 #8-10 or #1 (2018)
Not compiled, not tested, just typed.
enum CIbufers{clRL10, clRL30, clPsar} class CI{ public: void CI(int lengthRL10 = 10, int lengthRL30 = 30, double psarStep = 0.02, double psarMax = 0.2) : mRL10(lengthRL10), mRL30(lengthRL30), mStep(psarStep), mMax(psarMax){} double read(CLbuffers b, int shift){ return iCustom(_Symbol, _Period, "CIname", mRL10, mRL30, MStep, mMax, b, shift); } private: int mRL10, mRL30; double mStep, mMax; }Not compiled, not tested, just typed.

- Free trading apps
- Over 8,000 signals for copying
- Economic news for exploring financial markets
You agree to website policy and terms of use