Hi
There are a few mistakes in your code.
First, why do you activate trailing stop only when there is buy or sell signal? You should call “trailing stop” functions all the time when you have any opened positions from your EA (here you could add a counter for trades only from your EA instead PositlonsTotal()).
And within CheckTrailingStop… - you should compare
(PositionGetInteger(POSITION_TYPE)==POSITION_TYPE_SELL)
instead of
(PositionGetInteger(POSITION_TYPE)==ORDER_TYPE_SELL)
(same for BUY direction).
And in the modify function you cannot use direct TPprofit value as take profit level. You need to calculate new TP if you want to modify this also – or use current tp of the trade: PositionGetDouble(POSITION_TP).
Besides that – all prices should be normalized to tick size and you would have to check if the stop levels are not higher than new SL distance – to allow modification (https://book.mql4.com/appendix/limits) etc. But those are just good coding management.
Best Regards
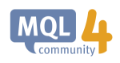
- book.mql4.com
Hi
There are a few mistakes in your code.
First, why do you activate trailing stop only when there is buy or sell signal? You should call “trailing stop” functions all the time when you have any opened positions from your EA (here you could add a counter for trades only from your EA instead PositlonsTotal()).
And within CheckTrailingStop… - you should compare
(PositionGetInteger(POSITION_TYPE)==POSITION_TYPE_SELL)
instead of
(PositionGetInteger(POSITION_TYPE)==ORDER_TYPE_SELL)
(same for BUY direction).
And in the modify function you cannot use direct TPprofit value as take profit level. You need to calculate new TP if you want to modify this also – or use current tp of the trade: PositionGetDouble(POSITION_TP).
Besides that – all prices should be normalized to tick size and you would have to check if the stop levels are not higher than new SL distance – to allow modification (https://book.mql4.com/appendix/limits) etc. But those are just good coding management.
Best Regards
Ok thank you for your help

- Free trading apps
- Over 8,000 signals for copying
- Economic news for exploring financial markets
You agree to website policy and terms of use
Good Morning to all members, I have coded a Cross Moving Average EA. And I want to activate a trailing stop when trade is in profit the trailing stop must be set at a certain level after open price for buy or below open price for sell.the problem is that the trailing stop does not activate when the conditions are met here is the mql5 code