does anyone know why i see so many failed trades???
<Improperly formatted code removed by moderator>
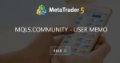
- www.mql5.com
AI generated code is not reliable at all and if you want to learn to code you should stay away from it...
so you need to use CopyBuffer in MQL5 code
something to help you:
input int distance = 50; input int ma_period = 18; // Period for the Moving Average input int shift = 0; // MA Shift input ENUM_MA_METHOD ma_method = MODE_SMA; // MA Smoothing type input ENUM_APPLIED_PRICE applied_price = PRICE_CLOSE; // Price mode for the MA int bars = 1000; int ma_handle = INVALID_HANDLE; double smaBuffer[]; int OnInit(){ ma_handle = iMA(Symbol(), Period(), ma_period, shift, ma_method, applied_price); ArraySetAsSeries(smaBuffer, true); return(INIT_SUCCEEDED); } void OnTick(){ CopyBuffer(ma_handle, 0, 0, bars, smaBuffer); double latest_bar_MA_value = smaBuffer[0]; double candleClosePrice = iClose(Symbol(), Period(), 1); bool MaCloseToPrice = (MathAbs(candleClosePrice - latest_bar_MA_value)/_Point < distance); if(MaCloseToPrice){ //make the trade because the MA is near the market price } }
#include <Trade\Trade.mqh> // Include the necessary header file input int EMA_Period = 20; // Period for the Exponential Moving Average input int TP_Pips = 50; // Take Profit in pips input int SL_Pips = 30; // Stop Loss in pips input int BreakEven_Pips = 20; // Pips to move stop loss to breakeven input double LotSize = 0.1; // Lot size datetime lastTradeTime = 0; // Last time a trade was opened //+------------------------------------------------------------------+ //| Expert initialization function | //+------------------------------------------------------------------+ int OnInit() { // Initialize your EA return INIT_SUCCEEDED; } //+------------------------------------------------------------------+ //| Expert deinitialization function | //+------------------------------------------------------------------+ void OnDeinit(const int reason) { // Perform any necessary cleanup before the EA is removed } //+------------------------------------------------------------------+ //| Expert tick function | //+------------------------------------------------------------------+ void OnTick() { double ema = iMA(NULL, 0, EMA_Period, 0, MODE_EMA, PRICE_CLOSE); // Calculate EMA if (TimeCurrent() - lastTradeTime < 86400) // Check if a trade has been opened within the last day (86400 seconds) return; double close[]; ArrayResize(close, 2); // Fetch historical prices if (CopyClose(_Symbol, Period(), 1, 2, close) == 2) { double prevClose = close[1]; // Previous close price double currentClose = close[0]; // Current close price double bid = SymbolInfoDouble(_Symbol, SYMBOL_BID); // Current bid price double ask = SymbolInfoDouble(_Symbol, SYMBOL_ASK); // Current ask price MqlTradeRequest request; // Declare the trade request structure // Check if price crosses above EMA if (prevClose < ema && currentClose > ema) { request.action = ENUM_TRADE_REQUEST_ACTIONS::TRADE_ACTION_DEAL; // Set the trade action request.type = ORDER_TYPE_BUY; request.symbol = _Symbol; request.volume = LotSize; request.price = ask; request.sl = ask - SL_Pips * Point; // Normalize stop loss request.tp = ask + TP_Pips * Point; // Normalize take profit request.comment = "Buy Order"; MqlTradeResult result; if(OrderSend(request, result)) lastTradeTime = TimeCurrent(); // Update last trade time } // Check if price crosses below EMA else if (prevClose > ema && currentClose < ema) { request.action = ENUM_TRADE_REQUEST_ACTIONS::TRADE_ACTION_DEAL; // Set the trade action request.type = ORDER_TYPE_SELL; request.symbol = _Symbol; request.volume = LotSize; request.price = bid; request.sl = bid + SL_Pips * Point; // Normalize stop loss request.tp = bid - TP_Pips * Point; // Normalize take profit request.comment = "Sell Order"; MqlTradeResult result; if(OrderSend(request, result)) lastTradeTime = TimeCurrent(); // Update last trade time } } } //+------------------------------------------------------------------+
#include <Trade\Trade.mqh> // Include the necessary header file here input int EMA_Period = 20; // this is the period for the Exponential Moving Average input int TP_Pips = 50; // Your take Profit in pips input int SL_Pips = 30; // Your stop Loss in pips input int BreakEven_Pips = 20; // Pips to move stop loss to breakeven input double LotSize = 0.1; // Lot size datetime lastTradeTime = 0; // Last time a trade was opened // Function to get the point value double GetPoint() { return SymbolInfoDouble(_Symbol, SYMBOL_POINT); } //+------------------------------------------------------------------+ //| Expert initialization function | //+------------------------------------------------------------------+ int OnInit() { // Initialize your EA return INIT_SUCCEEDED; } //+------------------------------------------------------------------+ //| Expert deinitialization function | //+------------------------------------------------------------------+ void OnDeinit(const int reason) { // Perform any necessary cleanup before the EA is removed } //+------------------------------------------------------------------+ //| Expert tick function | //+------------------------------------------------------------------+ void OnTick() { double ema = iMA(_Symbol, Period(), EMA_Period, 0, MODE_EMA, PRICE_CLOSE); // Calculate EMA if (TimeCurrent() - lastTradeTime < 86400) // Check if a trade has been opened within the last day (86400 seconds) return; double close[]; ArrayResize(close, 2); // Fetch historical prices if (CopyClose(_Symbol, Period(), 1, 2, close) == 2) { double prevClose = close[1]; // Previous close price double currentClose = close[0]; // Current close price double bid = SymbolInfoDouble(_Symbol, SYMBOL_BID); // Current bid price double ask = SymbolInfoDouble(_Symbol, SYMBOL_ASK); // Current ask price MqlTradeRequest request; // Declare the trade request structure // Check if price crosses above EMA if (prevClose < ema && currentClose > ema) { request.action = TRADE_ACTION_DEAL; // Set the trade action request.type = ORDER_TYPE_BUY; request.symbol = _Symbol; request.volume = LotSize; request.price = ask; request.sl = ask - SL_Pips * GetPoint(); // Normalize stop loss request.tp = ask + TP_Pips * GetPoint(); // Normalize take profit request.comment = "Buy Order"; MqlTradeResult result; if(OrderSend(request, result)) lastTradeTime = TimeCurrent(); // Update last trade time } // Check if price crosses below EMA else if (prevClose > ema && currentClose < ema) { request.action = TRADE_ACTION_DEAL; // Set the trade action request.type = ORDER_TYPE_SELL; request.symbol = _Symbol; request.volume = LotSize; request.price = bid; request.sl = bid + SL_Pips * GetPoint(); // Normalize stop loss request.tp = bid - TP_Pips * GetPoint(); // Normalize take profit request.comment = "Sell Order"; MqlTradeResult result; if(OrderSend(request, result)) lastTradeTime = TimeCurrent(); // Update last trade time } } }GetPoint() ;-)
Thanks for the tips! I got rid of the idea that I will learn it from Chat gpt, instead I watched some tutorials on youtube and start addingo to initial concept.
scope:
1. open only one position per calendar day
2. lot size dependant on %risk
3. look for short position only if price is below fast ema , which is below slow ema . so price < fast ema < slow ema
4. open short position when price touches the fast ema
5 trailing stop possible to set.
6. SL and TP based on ATR
The problem is, that when I backtest it, it only opens some positions where previous bar high intersects with fast ema;/ I can't figure out why it doesn't work if whole previous candle is below fast ema, and then current price touches the fast ema.
Could you have a look on these two from the sourcecode ? I thought it's because of the executeSell function, then I checked this one time per day settings, but not.... now i don't know what to do to improve it;/ I also tried this MQLtick to have a tick price, but also failed;/
if ( open < previousEMAfastVal && EMA1[0] < EMA2[0] && ( high >= previousEMAfastVal || tempriceBID >=currentEMAfastVal) ){ // Print("Sell signal świeczka najnowsza"); ExecuteSell(); } else if ( high <= previousEMAfastVal && EMA1[0] < EMA2[0] && (highcurrent >=previousEMAfastVal || tempriceBID >=currentEMAfastVal)) { ExecuteSell(); }
sourcecode
//+------------------------------------------------------------------+ //| kaj_tutorial.mq5 | //| Kajtek Kędior | //| https://www.mql5.com | //+------------------------------------------------------------------+ #property copyright "Kajtek Kędior" #property link "https://www.mql5.com" #property version "1.00" #include <trade/trade.mqh> int handleEMAfast; int handleEMAslow; int barsTotal; int handleATR; CTrade trade; input double Lots = 1; input ENUM_TIMEFRAMES Timeframe = PERIOD_M5; input int EMAfast = 288; input int EMAslow = 1440; input string Comentary = "EMA touch"; input double Tppoints = 3000; input double Slpoints = 3000; input double TslTrigger = 0; input double TlsPoints = 2000; input int Magic = 1; input double SELLATRMultiplierTP = 2; input double SELLATRMultiplierSL = 2; //+------------------------------------------------------------------+ //| Include | //+------------------------------------------------------------------+ #include <Expert\Expert.mqh> //--- available signals #include <Expert\Signal\SignalMA.mqh> //--- available trailing #include <Expert\Trailing\TrailingNone.mqh> //--- available money management #include <Expert\Money\MoneyFixedLot.mqh> //+------------------------------------------------------------------+ //| Inputs | //+------------------------------------------------------------------+ //+------------------------------------------------------------------+ //| Initialization function of the expert | //+------------------------------------------------------------------+ int OnInit() { trade.SetExpertMagicNumber(Magic); handleEMAfast = iMA(_Symbol,Timeframe,EMAfast,0,MODE_EMA,PRICE_CLOSE); handleEMAslow = iMA(_Symbol,Timeframe,EMAslow,0,MODE_EMA,PRICE_CLOSE); barsTotal = iBars(NULL,Timeframe); return(INIT_SUCCEEDED); } //+------------------------------------------------------------------+ //| Deinitialization function of the expert | //+------------------------------------------------------------------+ void OnDeinit(const int reason) { } //+------------------------------------------------------------------+ //| "Tick" event handler function | //+------------------------------------------------------------------+ void OnTick() { // tutaj cały opis jak się robi Trailing stop for(int i = 0; i < PositionsTotal(); i = i+1 ){ //i++ == i+1 double bid = SymbolInfoDouble(_Symbol, SYMBOL_BID); double ask = SymbolInfoDouble(_Symbol, SYMBOL_ASK); ulong PosTicket = PositionGetTicket(i); if (PositionGetInteger(POSITION_MAGIC) != Magic) continue; if (PositionGetSymbol(POSITION_SYMBOL) != _Symbol) continue; double PosPriceOpen = PositionGetDouble(POSITION_PRICE_OPEN); //Print(PosTicket, " > ", PosPriceOpen); //printing position number with position price double PosSL = PositionGetDouble(POSITION_SL); double PosTP = PositionGetDouble(POSITION_TP); if (PositionGetInteger(POSITION_TYPE) == POSITION_TYPE_BUY){ if (bid > PosPriceOpen +TslTrigger * _Point){ double sl = bid - TlsPoints * _Point; sl = NormalizeDouble(sl,_Digits); if (sl > PosSL){ trade.PositionModify(PosTicket,sl,PosTP); } } } else if(PositionGetInteger(POSITION_TYPE) == POSITION_TYPE_SELL){ if(ask < PosPriceOpen - TslTrigger * _Point){ double sl = ask + TlsPoints *_Point; sl = NormalizeDouble(sl,_Digits); if (sl < PosSL || sl == 0){ trade.PositionModify(PosTicket,sl,PosTP); } } } } //========================================================= //ceny bid ask tick //MqlTick last_tick; //double Bid = last_tick.bid; //double Ask = last_tick.ask; //Bid = NormalizeDouble(Bid,_Digits); //Ask = NormalizeDouble(Ask,_Digits); //Print( "last tick bid...", Bid,"last tick ask...",Ask); //przypisyanie koleych wartości wskaźnikowi double EMA1[]; //EMAfast array double EMA2[]; //EMAslow array //array[1] = 2141; //Print(array[1]); CopyBuffer(handleEMAfast,0,1,2,EMA1); CopyBuffer(handleEMAslow,0,1,1,EMA2); double currentEMAfastVal = EMA1[1]; double previousEMAfastVal = EMA1[0]; currentEMAfastVal = NormalizeDouble(currentEMAfastVal,_Digits); previousEMAfastVal = NormalizeDouble(previousEMAfastVal, _Digits); Print("ostatniaszybka...",currentEMAfastVal); Print("przedostatniaszybka...",previousEMAfastVal); //Print("emafast najswiezsza...", EMA1[0], "emafast wczesniejsza...", EMA1[1]); //Print("emaslow najswiezsza...", EMA2[0], "emaslow wczesniejsza...", EMA2[1]); double open = iOpen(NULL,Timeframe,1); double high = iHigh(NULL,Timeframe,1); double highcurrent = iHigh(NULL,Timeframe,0); highcurrent = NormalizeDouble(high,_Digits); high = NormalizeDouble(high,_Digits); Print("previous open is ",open, " previous high is...",high); //double close = iClose(NULL,Timeframe,2); int bars = iBars(NULL,Timeframe); double tempriceASK = SymbolInfoDouble(_Symbol,SYMBOL_ASK); tempriceASK = NormalizeDouble(tempriceASK,_Digits); double tempriceBID = SymbolInfoDouble(_Symbol,SYMBOL_BID); tempriceBID=NormalizeDouble(tempriceBID,_Digits); Print("current tempricebid...",tempriceBID); if(barsTotal != bars){ barsTotal = bars; if(DoWeHaveAlreadyOneTradeToday() == 0){ //function checking trade //buy signal // if (EMA1[0] > EMA2[0] && tempriceASK < EMA2[0] && open > EMA2[0]){ // Print("Buy sigbal"); // ExecuteBuy(); // } //else if ( open < previousEMAfastVal && EMA1[0] < EMA2[0] && ( high >= previousEMAfastVal || tempriceBID >=currentEMAfastVal) ){ // Print("Sell signal świeczka najnowsza"); ExecuteSell(); } else if ( high <= previousEMAfastVal && EMA1[0] < EMA2[0] && (highcurrent >=previousEMAfastVal || tempriceBID >=currentEMAfastVal)) { ExecuteSell(); } /*double handleATR1 = iATR(_Symbol,PERIOD_CURRENT,14); double ATR1[]; // ATR array CopyBuffer(handleATR1,0,1,1,ATR1); double TP = tempriceBID - ATR1[0]*SELLATRMultiplierTP; double SL = tempriceBID + ATR1[0]*SELLATRMultiplierSL; double SLpips = ATR1[0]*SELLATRMultiplierSL; //SL w pipsach SLpips = NormalizeDouble(SLpips, _Digits); TP = NormalizeDouble(TP,_Digits); SL = NormalizeDouble(SL,_Digits); double lots = dblLotsRisk(SLpips, 0.1); Print("wielkosc pozycja to....", lots); trade.Sell(lots,NULL,tempriceBID,SL,TP,Comentary); */ } } } void ExecuteSell(){ double entry = SymbolInfoDouble(_Symbol,SYMBOL_BID); entry = NormalizeDouble(entry,_Digits); //dostosowuje ile po przecinku ma symbol, inaczej z palca trzeba wpisać, a tak wystarczyz digitis Print(entry); double handleATR1 = iATR(_Symbol,PERIOD_CURRENT,14); double ATR1[]; // ATR array CopyBuffer(handleATR1,0,1,1,ATR1); double TP = entry - ATR1[0]*SELLATRMultiplierTP; double SL = entry + ATR1[0]*SELLATRMultiplierSL; double SLpips = ATR1[0]*SELLATRMultiplierSL; //SL w pipsach SLpips = NormalizeDouble(SLpips, _Digits); TP = NormalizeDouble(TP,_Digits); SL = NormalizeDouble(SL,_Digits); double lots = dblLotsRisk(SLpips, 0.1); Print("wielkosc pozycja to....", lots); trade.Sell(lots,NULL,entry,SL,TP,Comentary); } bool DoWeHaveAlreadyOneTradeToday(){ MqlDateTime today; datetime NullUhr; datetime now= TimeCurrent(today); int year = today.year; int month = today.mon; int day = today.day; NullUhr = StringToTime(string(year)+"."+string(month)+"."+string(day)+" 00:00"); HistorySelect(NullUhr, now); uint total= HistoryDealsTotal(); for(uint i=0;i<total;i++){ ulong ticket=HistoryDealGetTicket(i); string symbol=HistoryDealGetString(ticket,DEAL_SYMBOL); datetime time =(datetime)HistoryDealGetInteger(ticket,DEAL_TIME); if(time>NullUhr){ return true; } } return false; } // Calculate Max Lot Size based on Maximum Risk double dblLotsRisk( double dbStopLoss, double dbRiskRatio ) { double dbLotsMinimum = SymbolInfoDouble( _Symbol, SYMBOL_VOLUME_MIN ), dbLotsMaximum = SymbolInfoDouble( _Symbol, SYMBOL_VOLUME_MAX ), dbLotsStep = SymbolInfoDouble( _Symbol, SYMBOL_VOLUME_STEP ), dbTickSize = SymbolInfoDouble( _Symbol, SYMBOL_TRADE_TICK_SIZE ), dbTickValue = SymbolInfoDouble( _Symbol, SYMBOL_TRADE_TICK_VALUE ), dbValueAccount = fmin( fmin( AccountInfoDouble( ACCOUNT_EQUITY ) , AccountInfoDouble( ACCOUNT_BALANCE ) ), AccountInfoDouble( ACCOUNT_MARGIN_FREE ) ), dbValueRisk = dbValueAccount * dbRiskRatio, dbLossOrder = dbStopLoss * dbTickValue / dbTickSize, dbCalcLot = fmin( dbLotsMaximum, // Prevent too greater volume fmax( dbLotsMinimum, // Prevent too smaller volume round( dbValueRisk / dbLossOrder // Calculate stop risk / dbLotsStep ) * dbLotsStep ) ); // Align to step value return ( dbCalcLot ); }
You need to be checking if a position exists first before you modify any positions, so I added posTicket > 0 check in the conditions:
for(int i = 0; i < PositionsTotal(); i = i+1 ){ //i++ == i+1 double bid = SymbolInfoDouble(_Symbol, SYMBOL_BID); double ask = SymbolInfoDouble(_Symbol, SYMBOL_ASK); ulong PosTicket = PositionGetTicket(i); if (PositionGetInteger(POSITION_MAGIC) != Magic) continue; if (PositionGetSymbol(POSITION_SYMBOL) != _Symbol) continue; double PosPriceOpen = PositionGetDouble(POSITION_PRICE_OPEN); //Print(PosTicket, " > ", PosPriceOpen); //printing position number with position price double PosSL = PositionGetDouble(POSITION_SL); double PosTP = PositionGetDouble(POSITION_TP); if (posTicket > 0 && PositionGetInteger(POSITION_TYPE) == POSITION_TYPE_BUY){ if (bid > PosPriceOpen +TslTrigger * _Point){ double sl = bid - TlsPoints * _Point; sl = NormalizeDouble(sl,_Digits); if (sl > PosSL){ trade.PositionModify(PosTicket,sl,PosTP); } } } else if(posTicket > 0 && PositionGetInteger(POSITION_TYPE) == POSITION_TYPE_SELL){ if(ask < PosPriceOpen - TslTrigger * _Point){ double sl = ask + TlsPoints *_Point; sl = NormalizeDouble(sl,_Digits); if (sl < PosSL || sl == 0){ trade.PositionModify(PosTicket,sl,PosTP); } } } }
And you use copybuffer with a shift of 1 (start position) which might not give you valid signals. That means that it's waiting for 1 bar to pass when it already processed the data 1 bar before
CopyBuffer(handleEMAfast,0,1,2,EMA1); CopyBuffer(handleEMAslow,0,1,1,EMA2);
maybe try to use it this way:
CopyBuffer(handleEMAfast,0,0,2,EMA1); CopyBuffer(handleEMAslow,0,0,1,EMA2);
Thanks @Conor Mcnamara! I tried this,but still there is an issue with these if conditions where Execute sell is.
Let me show you on couple of screens. I thought it's because i used tempricebid when the price barely touch EMA but it is not that;/ I tired different settings and conditions and can't figure out why it is not working;/

- Free trading apps
- Over 8,000 signals for copying
- Economic news for exploring financial markets
You agree to website policy and terms of use