Count you interval (x2-x1) in candels. But you will have errors in case of missing candels.
Another way quite complicated
Another way quite complicated
Thanks. But I think I need a more precise solution if eqist.
For example, you can do something like this (Ligth blue line is a MA )
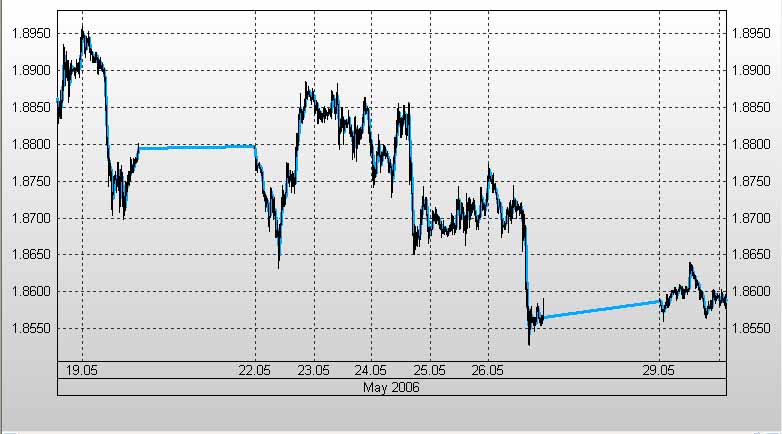
Put candels in new array leaving space for weekends and draw straight line.
If you want to do something vice versa you have to write function
bool WeekEnd( DateTime dt)
to count candels on interval [x1;x3] or to count weekends on it. then just make shorter intervals on WeekEndLength*WeekEndCount.
Quite simple :)
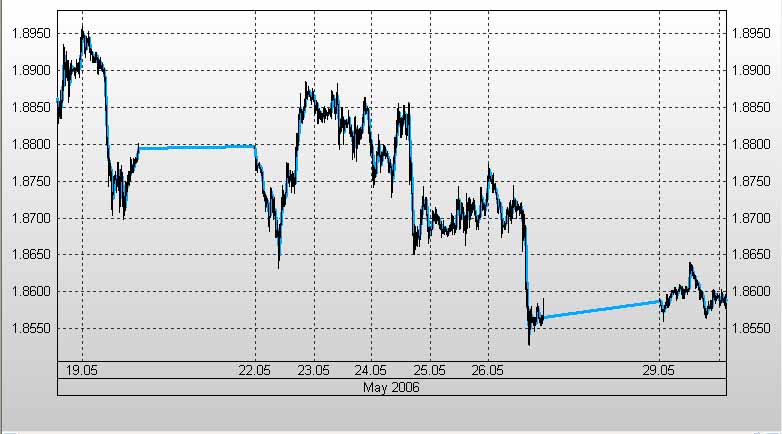
Put candels in new array leaving space for weekends and draw straight line.
If you want to do something vice versa you have to write function
bool WeekEnd( DateTime dt)
to count candels on interval [x1;x3] or to count weekends on it. then just make shorter intervals on WeekEndLength*WeekEndCount.
Quite simple :)
Enjoy if you can
version 1. errors possible.
version 1. errors possible.
//+------------------------------------------------------------------+ //| DateTime.mqh | //| Copyright © 2007, BabyBear. | //| babybear60@mail.ru | //+------------------------------------------------------------------+ #property copyright "Copyright © 2007, BabyBear." #property link "http://bearden.narod.ru/index.html" #define ONE_FRIDAY_END 1168038037 // 7*24*60*60 #define WEEK_LENGTH 604800 // (24 + 23)*60*60; #define WEEK_END_LENGTH 169200 datetime LastMondayStart( datetime dt) { datetime MondayStart = ONE_FRIDAY_END + WEEK_END_LENGTH; while ( MondayStart < dt) MondayStart += WEEK_LENGTH; return ( MondayStart - WEEK_LENGTH); } datetime NextFridayEnd( datetime dt) { datetime FridayEnd = ONE_FRIDAY_END; while ( FridayEnd < dt) FridayEnd += WEEK_LENGTH; return ( FridayEnd); } int SundayMidnightCount( datetime start, datetime end) { datetime s = LastMondayStart( start); datetime e = LastMondayStart( end); int result = 0; while( s < e) { s += WEEK_LENGTH; result++; } return (result); } int HoursCandleCount( datetime start, datetime end) { datetime s = start + SundayMidnightCount( start, end) * WEEK_END_LENGTH; int result = 0; while (s < end) { s += 3600; // one hour result++; } return (result); } datetime AddTradingSeconds( datetime start, int seconds) { int trading_week_length = 5*24*60*60; int s = seconds; datetime result = start; while ( s > trading_week_length) { s -= trading_week_length; result += WEEK_LENGTH; } if( NextFridayEnd( result) != NextFridayEnd( result + s)) result += 2*24*60*60; return ( result + s); }
Thank you very much BabyBear!
In the meantime I have solved the problem with a manual method.
But I show your program to my friend who programming for me. I hope he can use it and can make me a full automatic "indicator".
Thanks again!
In the meantime I have solved the problem with a manual method.
But I show your program to my friend who programming for me. I hope he can use it and can make me a full automatic "indicator".
Thanks again!

You are missing trading opportunities:
- Free trading apps
- Over 8,000 signals for copying
- Economic news for exploring financial markets
Registration
Log in
You agree to website policy and terms of use
If you do not have an account, please register
To be clear:
I have two time coordinate in the past (X1 & X2) and i want a third (X3) in the future.
So X3=X2+(X2-X1)
But my problem is that the program count in calendar time and on the charts the x3!=(not equal)X2+(X2-X1), because of the weekends.
How could I solve this problem? Or which commands have to use?
Thanks!