Please consider which section is most appropriate — https://www.mql5.com/en/forum/172166/page6#comment_49114893
Thanks and sorry about that.
Since it's more of a price action indicator, I thought the term "technical" didn't fit here. My mistake.
Anyways...I'm not sure what's the problem with my code, it looks like it's pretty straightforward.
Maybe something wrong with the main loop?
Still waiting for help :)
- You have not explained what you consider as "not working".
- You have set the Buy and Sell buffers as a "series", but you have not done the same for the OHLC buffers.
Since this MQL5 and the default is "non-series", don't set the buffers as "series". - You are starting the loop at "i = 1", but you are referencing the index "i - 4" which would be an invalid index of "-3".
- Also you are assigning values to the Buy and Sell buffers when one of the conditions are met, but don't assign any value when the conditions are NOT met.
In other words, you should be assigning the value EMPTY_VALUE in those cases.
BuyBuffer[ i] = tipo1Compra || tipo2Compra || tipo3Compra ? close[i-1] : EMPTY_VALUE; SellBuffer[i] = tipo1Venda || tipo2Venda || tipo3Venda ? close[i-1] : EMPTY_VALUE;
for(int i=1; i<rates_total; i++) { tipo1Compra = (open[i-4]<close[i-4
-
When i equals one, you try to access element minus three. Indicator crashes.
How to do your lookbacks correctly #9 — #14 & #19 (2016) -
You haven't specified whether you are accessing your arrays as-series or non-series. Your buffers are set as-series.
To define the indexing direction in the time[], open[], high[], low[], close[], tick_volume[], volume[] and spread[] arrays, call the ArrayGetAsSeries() function. In order not to depend on defaults, call the ArraySetAsSeries() function for the arrays to work with.
Event Handling / OnCalculate - Reference on algorithmic/automated trading language for MetaTrader 5
William Roeder #:
-
When i equals one, you try to access element minus three. Indicator crashes.
How to do your lookbacks correctly #9 — #14 & #19 (2016) -
You haven't specified whether you are accessing your arrays as-series or non-series. Your buffers are set as-series.
Many thanks for both of your responses!
I've commented out the "buffer set as series" function and changed the main loop to prevent from crashing when there's not enough bars in the chart. And now it is working (it displays the Buy and Sell buffers at the right places on the chart).
But i suspect that what i did was not the best solution though. This is how I wrote the loop:
if(rates_total<5) { return(0); } for(int i=5; i<rates_total; i++)
Again,it does work but i think it's far from optimal..
Could you please help me with this loop to make it more efficient?
thanks in advance
Make use of the parameter variable "prev_calculated" so that you don't recalculate everything on each call.
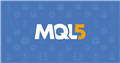
I see some potential issues in your code that might be causing it not to work as expected. Here are a few suggestions:
-
Array Indexing: Ensure that your array indexing is within bounds to avoid accessing elements outside the array.
-
Initialization: Initialize your boolean variables ( tipo1Compra , tipo1Venda , etc.) at the beginning of the OnCalculate function. This helps in preventing unexpected behavior.
-
Plotting Arrows: Make sure that the conditions for plotting arrows are met. You are currently plotting arrows at every bar, but you might want to plot them only when a buy or sell condition occurs.
-
Buffer Assignments: The assignment of values to BuyBuffer and SellBuffer should be conditional on whether a buy or sell condition is met. Ensure that you are assigning values only when the conditions are true.
Here's an updated version of your OnCalculate function with these considerations:
int OnCalculate(const int rates_total, const int prev_calculated, const datetime &time[], const double &open[], const double &high[], const double &low[], const double &close[], const long &tick_volume[], const long &volume[], const int &spread[]) { // Initialize boolean variables bool tipo1Compra, tipo1Venda, tipo2Compra, tipo2Venda, tipo3Compra, tipo3Venda; for (int i = 1; i < rates_total; i++) { // Your existing conditions for pattern detection if (tipo1Compra || tipo2Compra || tipo3Compra) { BuyBuffer[i] = close[i - 1]; SellBuffer[i] = 0; // Reset SellBuffer to avoid conflicting signals } if (tipo1Venda || tipo2Venda || tipo3Venda) { SellBuffer[i] = close[i - 1]; BuyBuffer[i] = 0; // Reset BuyBuffer to avoid conflicting signals } } return (rates_total); }
This modification resets the opposite buffer whenever a buy or sell condition is met to avoid conflicting signals. Adjust this according to your strategy logic. Also, make sure to test this thoroughly in a safe environment before using it with real funds.
Awesome! Thanks a lot for all the help
For anyone that might use this topic for educational purposes:
Since I fixed the buffer array ordering and the main loop to avoid crashing when it returned [-3] It's now totally functional.
Now I'm just tweaking the main loop to make it more efficient computation-wise.

- Free trading apps
- Over 8,000 signals for copying
- Economic news for exploring financial markets
You agree to website policy and terms of use
Hi guys! I'm trying to code a simple indicator that identifies a pattern of 4 bullish/bearish candles in a particular order and whenever it finds one of the 3 patterns, it should set a buffer for buying/Selling at the Close price of the last candle, drawing arrows. But I can't make it work and I don't know why.
Can anyone help me with that, please?
Here's the code: