Please consider which section is most appropriate — https://www.mql5.com/en/forum/172166/page6#comment_49114893
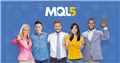
- www.mql5.com
double last_bid = last_tick.bid; double last_ask = last_tick.ask; int openpositions = PositionsTotal(); double bsl = last_ask - 150*_Point; double btp = last_bid + 300*_Point; double ssl = last_bid + 150*_Point; double stp = last_ask - 300*_Point;
Those are not assignments; they are initialization of a common (globally declared), or static variable(s) with a constant. They work exactly the same way in MT4/MT5/C/C++.
-
They are initialized once on program load.
-
They don't update unless you assign to them.
-
In C/C++ you can only initialize them with constants, and they default to zero. In MTx you should only initialize them with constants. There is no default in MT5, or MT4 with strict (which you should always use).
MT4/MT5 actually compiles with non-constants, but the order that they are initialized is unspecified and Don't try to use any price (or indicator) or server related functions in OnInit (or on load or in OnTimer before you've received a tick), as there may be no connection/chart yet:
- Terminal starts.
- Indicators/EAs are loaded. Static and globally declared variables are initialized. (Do not depend on a specific order.)
- OnInit is called.
- For indicators OnCalculate is called with any existing history.
- Human may have to enter password, connection to server begins.
- New history is received, OnCalculate called again.
- A new tick is received, OnCalculate/OnTick is called. Now TickValue, TimeCurrent, account information and prices are valid.
-
Unlike indicators, EAs are not reloaded on chart change, so you must reinitialize them, if necessary.
external static variable - MQL4 programming forum #2 (2013)
Those are not assignments; they are initialization of a common (globally declared), or static variable(s) with a constant. They work exactly the same way in MT4/MT5/C/C++.
-
They are initialized once on program load.
-
They don't update unless you assign to them.
-
In C/C++ you can only initialize them with constants, and they default to zero. In MTx you should only initialize them with constants. There is no default in MT5, or MT4 with strict (which you should always use).
MT4/MT5 actually compiles with non-constants, but the order that they are initialized is unspecified and Don't try to use any price (or indicator) or server related functions in OnInit (or on load or in OnTimer before you've received a tick), as there may be no connection/chart yet:
- Terminal starts.
- Indicators/EAs are loaded. Static and globally declared variables are initialized. (Do not depend on a specific order.)
- OnInit is called.
- For indicators OnCalculate is called with any existing history.
- Human may have to enter password, connection to server begins.
- New history is received, OnCalculate called again.
- A new tick is received, OnCalculate/OnTick is called. Now TickValue, TimeCurrent, account information and prices are valid.
-
Unlike indicators, EAs are not reloaded on chart change, so you must reinitialize them, if necessary.
external static variable - MQL4 programming forum #2 (2013)

- Free trading apps
- Over 8,000 signals for copying
- Economic news for exploring financial markets
You agree to website policy and terms of use
Hi All, I am attempting to create an EA that places two opposing trades at every 0.01 of price, and only places another after these two have been closed, this my first attempt at building an EA and Im not quite sure why the code attached does not work, could somebody please point me in the right direction?