Greetings,
I'm studying MQL4 and MQL5 for a few years now (I'm not a professional programmer, I'm self educated), but I find hard to understand topics still. Recently, I wanted to store chart object data from a sqlite database to a multi-dimensional array in a project, to minimize disk I/O. As far as I know, I need to declare the array somewhat like this:
But when I try to work with the array, so like to search in it with ArrayBsearch, I need to sort it first:
This way I get an error:
'ArraySort' - constant cannot be modified
At this point, there were no data transaction between the database and the array, the error showed up when I added the array to the code. I tried to comment out each and every data types, same error. I tried ArraySort with a one-dimensional integer type array, to test, all went well. So it is quite sure, the struct declaration, or the struct-based array causes the error.
It is also veird, that I cannot add a string to the struct with that syntax, I get a
'MarkerArray' - structures or classes containing objects are not allowed
built-in: bool ArraySort(void&)
I'm quite new to arrays (used a few here and there, but mostly one-dimensional), and never used structs before. What do I do wrong? The documentation is not clear, and I did not find relevant topics neither on the web, nor on youtube. Please help.
EDIT: the code was written in MQL5, not sure if there are any differences in the relevant sections between the 2 language variants.
The documentation reads:
"Sorts the values in the first dimension of a multidimensional numeric array in the ascending order."
So I thought it should work like:
MarkerArray[0]: Period_M1 ... 2023.08.15. 14:01 ... BUY
MarkerArray[1]: Period_M30 ... 2023.08.12. 12:00 ... SELL
MarkerArray[2]: Period_M5 ... 2023.08.17. 19:10 ... BUY
MarkerArray[3]: Period_M15 ... 2023.06.25. 19:45 ... BUY
MarkerArray[4]: Period_H1 ... 2023.05.15. 11:00 ... SELL
will go like:
MarkerArray[0]: Period_H1 ... 2023.05.15. 11:00 ... SELL
MarkerArray[1]: Period_M1 ... 2023.08.15. 14:01 ... BUY
MarkerArray[2]: Period_M15 ... 2023.06.25. 19:45 ... BUY
MarkerArray[3]: Period_M30 ... 2023.08.12. 12:00 ... SELL
MarkerArray[4]: Period_M5 ... 2023.08.17. 19:10 ... BUY
When I tried the array.Sort() I got some different errors, but I didn't pay close attention, since I was thinking about the limitations you mentioned.
I don't really care about the order, I just wanted to verify existence of a certain datetime in the array, and ArrayBsearch requires the array to be sorted. But in that case, if the array with a struct format has such limitations I should approach this task from a for loop perspective? Something like:
for(int i = 0; i == ArraySize(MarkerData); i++) { if(MarkerData[i].timestamp == <DATETIME TO MATCH>) { some operations } }
?
Maybe, I sorted it out for myself, but will need to try it out (haven't done yet).
The documentation reads:
"Sorts the values in the first dimension of a multidimensional numeric array in the ascending order."
So I thought it should work like:
MarkerArray[0]: Period_M1 ... 2023.08.15. 14:01 ... BUY
MarkerArray[1]: Period_M30 ... 2023.08.12. 12:00 ... SELL
MarkerArray[2]: Period_M5 ... 2023.08.17. 19:10 ... BUY
MarkerArray[3]: Period_M15 ... 2023.06.25. 19:45 ... BUY
MarkerArray[4]: Period_H1 ... 2023.05.15. 11:00 ... SELL
will go like:
MarkerArray[0]: Period_H1 ... 2023.05.15. 11:00 ... SELL
MarkerArray[1]: Period_M1 ... 2023.08.15. 14:01 ... BUY
MarkerArray[2]: Period_M15 ... 2023.06.25. 19:45 ... BUY
MarkerArray[3]: Period_M30 ... 2023.08.12. 12:00 ... SELL
MarkerArray[4]: Period_M5 ... 2023.08.17. 19:10 ... BUY
When I tried the array.Sort() I got some different errors, but I didn't pay close attention, since I was thinking about the limitations you mentioned.
I don't really care about the order, I just wanted to verify existence of a certain datetime in the array, and ArrayBsearch requires the array to be sorted. But in that case, if the array with a struct format has such limitations I should approach this task from a for loop perspective? Something like:
?
Maybe, I sorted it out for myself, but will need to try it out (haven't done yet).
const int compare(const my_struct& left, const my_struct& right)
{
return(left.timestamp - right.timestamp); // or whatever your comparison might be...
}
array.Sort(compare);
The goal is to create a buttonpress function, which should, if an object exist with the matching ENUM_TIMEFRAME and timestamp values, modify the object properties, otherwise create an object. My main problem is, I have no idea how could I express a proper pattern in a separate sort function, since I'm not a professional programmer. The first dimension in the array is an ENUM_TIMEFRAMES value, but I also tried to rearrange the fields, in hope of the ArraySort will work, but it didn't. Time complexity is an important aspect, since there might be over 1000 elements in the array after some time, so I might simply just drop the array approach, and will operate with database queries instead (the idea was to minimize disk I/O when adding elements, but it is worth to change back to the original plan due to the fact, that for me it seems to be esaier to express db queries instead of the sort on a struct array).
The goal is to create a buttonpress function, which should, if an object exist with the matching ENUM_TIMEFRAME and timestamp values, modify the object properties, otherwise create an object. My main problem is, I have no idea how could I express a proper pattern in a separate sort function, since I'm not a professional programmer. The first dimension in the array is an ENUM_TIMEFRAMES value, but I also tried to rearrange the fields, in hope of the ArraySort will work, but it didn't. Time complexity is an important aspect, since there might be over 1000 elements in the array after some time, so I might simply just drop the array approach, and will operate with database queries instead (the idea was to minimize disk I/O when adding elements, but it is worth to change back to the original plan due to the fact, that for me it seems to be esaier to express db queries instead of the sort on a struct array).
A database for 1000 objects ? Not a good idea.
Just use a hashmap with the timeframe/timestamp as a key. You will find a library in Include/Generic.
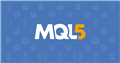
- www.mql5.com
A database for 1000 objects ? Not a good idea.
Just use a hashmap with the timeframe/timestamp as a key. You will find a library in Include/Generic.
Come on, people want things working and the hashmap from MQ is working fine. If he wants to use a tree, that's fine for me too. He could also use an array by the way, he just doesn't know how.
Don't go off-topic ;-)

- Free trading apps
- Over 8,000 signals for copying
- Economic news for exploring financial markets
You agree to website policy and terms of use
Greetings,
I'm studying MQL4 and MQL5 for a few years now (I'm not a professional programmer, I'm self educated), but I find hard to understand topics still. Recently, I wanted to store chart object data from a sqlite database to a multi-dimensional array in a project, to minimize disk I/O. As far as I know, I need to declare the array somewhat like this:
But when I try to work with the array, so like to search in it with ArrayBsearch, I need to sort it first:
ArraySort(MarkerArray);
This way I get an error:
'ArraySort' - constant cannot be modified
At this point, there were no data transaction between the database and the array, the error showed up when I added the array to the code. I tried to comment out each and every data types, same error. I tried ArraySort with a one-dimensional integer type array, to test, all went well. So it is quite sure, the struct declaration, or the struct-based array causes the error.
It is also veird, that I cannot add a string to the struct with that syntax, I get a
'MarkerArray' - structures or classes containing objects are not allowed
built-in: bool ArraySort(void&)
I'm quite new to arrays (used a few here and there, but mostly one-dimensional), and never used structs before. What do I do wrong? The documentation is not clear, and I did not find relevant topics neither on the web, nor on youtube. Please help.
EDIT: the code was written in MQL5, not sure if there are any differences in the relevant sections between the 2 language variants.