Your topic has been moved to the section: Expert Advisors and Automated Trading — In the future, please consider which section is most appropriate for your query.
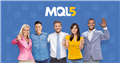
MQL5 forum: Expert Advisors and Automated Trading
- www.mql5.com
How to create an Expert Advisor (a trading robot) for Forex trading
Fernando Carreiro #:
Your topic has been moved to the section: Expert Advisors and Automated Trading — In the future, please consider which section is most appropriate for your query.
Your topic has been moved to the section: Expert Advisors and Automated Trading — In the future, please consider which section is most appropriate for your query.
Ok noted, its most appropriate so am waiting for your opinion on it.
I see so many EA'S Without a news filter, are they implying that their EA would win even if there is news?
Henry Asaba Achankeng #:
well if it was designed to work in the news then yes.
I noticed you are a pro developer.
No help for the needy :) ?
Your approach to solve this issue is very unintuitive.
You should hold an array of datetime with events.
This array should be created once and only updated if....
Then you check the array of datetime if TimeCurrent is approaching your events to avoid.
While you are in such a phase and all positions are closed/the EA is waiting, you could use that time to update your array of datetimes.
By splitting your functionality into smaller sub-pieces, it's much easier to manage your code.
The all in one function, you are using at the moment is a little messy and inefficient.
Dominik Christian Egert #:
Your approach to solve this issue is very unintuitive.
You should hold an array of datetime with events.
This array should be created once and only updated if....
Then you check the array of datetime if TimeCurrent is approaching your events to avoid.
While you are in such a phase and all positions are closed/the EA is waiting, you could use that time to update your array of datetimes.
By splitting your functionality into smaller sub-pieces, it's much easier to manage your code.
The all in one function, you are using at the moment is a little messy and inefficient.
okay i missed part of the code written by my partner. Actually the issue is already solved in the code but I did not notice.
Thanks for the advice
//// if(FilterHigh == true && UseNewsFilter == true) { DodgeMainHigh = HighEventDodge(MainCurrency,minutesBeforeHigh,minutesAfterHigh); DodgeSecHigh = HighEventDodge(SecondaryCurrency,minutesBeforeHigh,minutesAfterHigh); } if(FilterMed == true && UseNewsFilter == true) { DodgeMainMed = MedEventDodge(MainCurrency,minutesBeforeMed,minutesAfterMed); DodgeSecMed = MedEventDodge(SecondaryCurrency,minutesBeforeMed,minutesAfterMed); } if(UseNewsFilter == true && MinBeforeHighEvent > 0 && MinAfterHighEvent > 0 && FilterHigh == true) { EventStop = DodgeMainHigh || DodgeSecHigh; if(EventStop == true) { Print("--- Stoping for High news events ---"); sqCloseAllPositions("Any", MagicNumber, 1, ""); sqCloseAllPositions("Any", MagicNumber,-1, ""); } } if(UseNewsFilter == true && MinBeforeMedEvent > 0 && MinAfterMedEvent > 0 && FilterMed == true) { EventStop = DodgeMainMed || DodgeSecMed; if(EventStop == true) { Print("--- Stoping for Med news events ---"); sqCloseAllPositions("Any", MagicNumber, 1, ""); sqCloseAllPositions("Any", MagicNumber,-1, ""); } } ////

You are missing trading opportunities:
- Free trading apps
- Over 8,000 signals for copying
- Economic news for exploring financial markets
Registration
Log in
You agree to website policy and terms of use
If you do not have an account, please register
I am sharing this code with you in hope that you can help me to add the features for it to close all open trades x minutes before the news. Its MT5
If you can advice me on a feature to backtest news it would be a plus.
Code
Input:
Logic: