Berk Cetiner: Hello, if i backtest my ea with visual mode. it trades and works perfect but if i close visual mode during backtesting or starting optimization, i can not see any trades? Can someone help me why this occurs? Thanks
Without seeing the code, it will be difficult! Are there any entries in the log that might identify the issue?
Also, Graphical Objects are not fully supported during "non visual mode", and definitely not during optimisations. Maybe that is the cause.
Fernando Carreiro #: Without seeing the code, it will be difficult! Are there any entries in the log that might identify the issue? Also, Graphical Objects are not fully supported during "non visual mode", and definitely not during optimisations. Maybe that is the cause.
Hello, i found the issue why it doesn't trade when visual mode is on.
if visual mode is off, backtest can't find the value of indicator's buffer but with visual mode on, it can see the buffer value...
I am using "0" and "1" buffers which are BufferArrowDn and BufferArrowUp.
I will be happy if you can help me to fix why this occurs because i am confused, thanks.
#property copyright "Copyright 2018, MetaQuotes Software Corp." #property link "https://mql5.com" #property version "1.00" #property indicator_separate_window #property indicator_buffers 6 #property indicator_plots 5 //--- plot Arrow to up #property indicator_label1 "Long signal" #property indicator_type1 DRAW_ARROW #property indicator_color1 clrBlue #property indicator_style1 STYLE_SOLID #property indicator_width1 1 //--- plot Arrow to down #property indicator_label2 "Short signal" #property indicator_type2 DRAW_ARROW #property indicator_color2 clrRed #property indicator_style2 STYLE_SOLID #property indicator_width2 1 //--- plot divergence line to up #property indicator_label3 "Line to up" #property indicator_type3 DRAW_LINE #property indicator_color3 clrGreen #property indicator_style3 STYLE_SOLID #property indicator_width3 1 //--- plot divergence line to down #property indicator_label4 "Line to down" #property indicator_type4 DRAW_LINE #property indicator_color4 clrRed #property indicator_style4 STYLE_SOLID #property indicator_width4 1 //--- plot AO #property indicator_label5 "OSMA" #property indicator_type5 DRAW_LINE #property indicator_color5 clrGreen #property indicator_style5 STYLE_SOLID #property indicator_width5 1 //--- input parameters input uint InpFastEma = 12; input uint InpSlowEma = 26; input uint InpSignalEma = 9; // Period input ENUM_APPLIED_PRICE InpAppliedPrice = PRICE_CLOSE; // Applied price input color InpColorBullish = clrBlue; // Bullish color input color InpColorBearish = clrRed; // Bearish color //--- indicator buffers double BufferArrowToUP[]; double BufferLineToUP[]; double BufferArrowToDN[]; double BufferLineToDN[]; double BufferOSMA[]; double BufferATR[]; //--- global variables string prefix; int periodfast; int periodslow; int periodsignal; int handle_OSMA; int handle_atr; //+------------------------------------------------------------------+ //| Custom indicator initialization function | //+------------------------------------------------------------------+ int OnInit() { //--- setting global variables periodfast=int(InpFastEma<4 ? 3 : InpFastEma); periodslow=int(InpSlowEma<4 ? 3 : InpSlowEma); periodsignal=int(InpSignalEma<4 ? 3 : InpSignalEma); prefix="OSMAdiv"; //--- indicator buffers mapping SetIndexBuffer(0,BufferArrowToUP,INDICATOR_DATA); SetIndexBuffer(1,BufferArrowToDN,INDICATOR_DATA); SetIndexBuffer(2,BufferLineToUP,INDICATOR_DATA); SetIndexBuffer(3,BufferLineToDN,INDICATOR_DATA); SetIndexBuffer(4,BufferOSMA,INDICATOR_DATA); SetIndexBuffer(5,BufferATR,INDICATOR_CALCULATIONS); //--- settings indicators parameters IndicatorSetInteger(INDICATOR_DIGITS,Digits()); IndicatorSetString(INDICATOR_SHORTNAME,"Osma Divergence"); //--- setting buffer arrays as timeseries ArraySetAsSeries(BufferArrowToUP,true); ArraySetAsSeries(BufferArrowToDN,true); ArraySetAsSeries(BufferLineToUP,true); ArraySetAsSeries(BufferLineToDN,true); ArraySetAsSeries(BufferOSMA,true); ArraySetAsSeries(BufferATR,true); //--- setting plot buffer colors PlotIndexSetInteger(0,PLOT_ARROW,241); PlotIndexSetInteger(0,PLOT_LINE_COLOR,InpColorBullish); PlotIndexSetInteger(1,PLOT_ARROW,242); PlotIndexSetInteger(1,PLOT_LINE_COLOR,InpColorBearish); PlotIndexSetInteger(2,PLOT_LINE_COLOR,InpColorBullish); PlotIndexSetInteger(3,PLOT_LINE_COLOR,InpColorBearish); //--- create MA and ATR handles ResetLastError(); handle_OSMA=iOsMA(Symbol(),PERIOD_CURRENT,periodfast,periodslow,periodsignal,InpAppliedPrice); if(handle_OSMA==INVALID_HANDLE) { Print("The iOSMA(",(string)periodfast,") object was not created: Error ",GetLastError()); return INIT_FAILED; } ResetLastError(); handle_atr=iATR(Symbol(),PERIOD_CURRENT,14); if(handle_atr==INVALID_HANDLE) { Print("The iATR object was not created: Error ",GetLastError()); return INIT_FAILED; } return(INIT_SUCCEEDED); } //+------------------------------------------------------------------+ //| Custom indicator deinitialization function | //+------------------------------------------------------------------+ void OnDeinit(const int reason) { ObjectsDeleteAll(0,prefix); ChartRedraw(); } //+------------------------------------------------------------------+ //| Custom indicator iteration function | //+------------------------------------------------------------------+ int OnCalculate(const int rates_total, const int prev_calculated, const datetime &time[], const double &open[], const double &high[], const double &low[], const double &close[], const long &tick_volume[], const long &volume[], const int &spread[]) { //--- Проверка на минимальное количество баров для расчёта if(rates_total<periodfast+6 || Point()==0) return 0; //--- Установка индексации массивов как таймсерий ArraySetAsSeries(high,true); ArraySetAsSeries(low,true); ArraySetAsSeries(time,true); //--- Проверка и расчёт количества просчитываемых баров int limit=rates_total-prev_calculated; if(limit>1) { limit=rates_total-10; ArrayInitialize(BufferArrowToUP,EMPTY_VALUE); ArrayInitialize(BufferArrowToDN,EMPTY_VALUE); ArrayInitialize(BufferLineToUP,EMPTY_VALUE); ArrayInitialize(BufferLineToDN,EMPTY_VALUE); ArrayInitialize(BufferOSMA,EMPTY_VALUE); } //--- Подготовка данных int copied=0,count=(limit==0 ? 1 : rates_total); copied=CopyBuffer(handle_OSMA,0,0,count,BufferOSMA); if(copied!=count) return 0; copied=CopyBuffer(handle_atr,0,0,count,BufferATR); if(copied!=count) return 0; //--- Расчёт индикатора for(int i=limit; i>=0 && !IsStopped(); i--) { BufferLineToUP[i]=BufferLineToDN[i]=BufferArrowToUP[i]=BufferArrowToDN[i]=EMPTY_VALUE; ProcessBullish(rates_total,high,low,time,i+1); ProcessBearish(rates_total,high,low,time,i+1); } //--- return value of prev_calculated for next call return(rates_total); } bool IsTrough(const int &rates_total,const int index) { if(BufferOSMA[index]<0 && BufferOSMA[index]<BufferOSMA[index+1] && BufferOSMA[index]<BufferOSMA[index-1]) { for(int n=index+1 ;n<rates_total-2; n++) { if(BufferOSMA[n]<0 ) return true; else if(BufferOSMA[index]>BufferOSMA[n]) return false; } } return false; } int PrevTrough(const int &rates_total,const int index) { for(int n=index+1; n<rates_total-3; n++) { if(BufferOSMA[n]<=BufferOSMA[n+1] && BufferOSMA[n]<BufferOSMA[n+2] && BufferOSMA[n]<=BufferOSMA[n-1] && BufferOSMA[n]<BufferOSMA[n-2] && BufferOSMA[n-1]<0 && BufferOSMA[n-2]<0) return n; } return WRONG_VALUE; } //+------------------------------------------------------------------+ //| Поиск бычьих дивергенций | //+------------------------------------------------------------------+ void ProcessBullish(const int &rates_total,const double &high[],const double &low[],const datetime &time[],const int index) { if(IsTrough(rates_total,index)) { int curr=index; int prev=PrevTrough(rates_total,index); if(prev!=WRONG_VALUE) { if(BufferOSMA[curr]>BufferOSMA[prev] && low[curr]<low[prev]) DrawDivergence(high,low,time,prev,curr,true); else if(BufferOSMA[curr]<BufferOSMA[prev] && low[curr]>low[prev]) DrawDivergence(high,low,time,prev,curr,true); } } } bool IsPeak(const int &rates_total,const int index) { if(BufferOSMA[index]>0 && BufferOSMA[index]>BufferOSMA[index+1] && BufferOSMA[index]>BufferOSMA[index-1]) { for(int n=index+1; n<rates_total-2; n++) { if(BufferOSMA[n]>0) return true; else if(BufferOSMA[index]<BufferOSMA[n]) return false; } } return false; } int PrevPeak(const int &rates_total,const int index) { for(int n=index+1; n<rates_total-3; n++) { if(BufferOSMA[n]>=BufferOSMA[n+1] && BufferOSMA[n]>BufferOSMA[n+2] && BufferOSMA[n]>=BufferOSMA[n-1] && BufferOSMA[n]>BufferOSMA[n-2]&& BufferOSMA[n-1]>0 && BufferOSMA[n-2]>0) return n; } return WRONG_VALUE; } void ProcessBearish(const int &rates_total,const double &high[],const double &low[],const datetime &time[],const int index) { if(IsPeak(rates_total,index)) { int curr=index; int prev=PrevPeak(rates_total,index); if(prev!=WRONG_VALUE) { if(BufferOSMA[curr]<BufferOSMA[prev] && high[curr]>high[prev]) DrawDivergence(high,low,time,prev,curr,false); else if(BufferOSMA[curr]>BufferOSMA[prev] && high[curr]<high[prev]) DrawDivergence(high,low,time,prev,curr,false); } } } void DrawDivergence(const double &high[],const double &low[],const datetime &time[],const int first,const int second,const bool bull_flag) { string obj_name=prefix+(string)(long)time[first]+"_"+(string)(long)time[second]; int wnd; if(ObjectFind(0,obj_name)<0) { wnd=ChartWindowFind(); if(wnd!=WRONG_VALUE) { if(bull_flag) { BufferArrowToUP[second]=BufferOSMA[second]; for(int j=first; j>=second; j--) BufferLineToUP[j]=EquationDirect(first,BufferOSMA[first],second,BufferOSMA[second],j); //--- } else { BufferArrowToDN[second]=BufferOSMA[second]; for(int j=first; j>=second; j--) BufferLineToDN[j]=EquationDirect(first,BufferOSMA[first],second,BufferOSMA[second],j); //--- } } } } double EquationDirect(const int left_bar,const double left_price,const int right_bar,const double right_price,const int bar_to_search) { return(right_bar==left_bar ? left_price : (right_price-left_price)/(right_bar-left_bar)*(bar_to_search-left_bar)+left_price); } //+------------------------------------------------------------------+
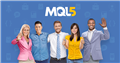
Discover new MetaTrader 5 opportunities with MQL5 community and services
- 2023.06.06
- www.mql5.com
MQL5: language of trade strategies built-in the MetaTrader 5 Trading Platform, allows writing your own trading robots, technical indicators, scripts and libraries of functions

You are missing trading opportunities:
- Free trading apps
- Over 8,000 signals for copying
- Economic news for exploring financial markets
Registration
Log in
You agree to website policy and terms of use
If you do not have an account, please register
Can someone help me why this occurs? Thanks