I have attached the code below for your reference.
I am trying to remove the second row.
But I only got the following output:
As shown in the documentation linked https://docs.mql4.com/array/arrayresize, it should return the size if it is resized, but in this case, i checked ArraySize again and it isn't resized (4x3=12) and when printed, it still shows 3 rows.
How do I remove the 3rd row? Basically I want create a function that removes the rows with all zero's in a 2D array and size it down.
What output ? nothing posted.
Didn't check your code closely, but for multi-dimension array, you need to use ArrayRange(). Please check it.
Thank you for this, I will use ArrayRange() from now on, but perhaps I am not doing it correctly. I have adjusted the above code below.
int arr[][4] = {{1,2,3,4},{5,6,7,8},{9,10,11,12}}; void OnInit() { int arrRowsSize = ArrayRange(arr,0); // will return 3 int arrColSize = ArraySize(arr)/3; Print("arrColSize: " + arrColSize); Print("arrRowsSize: " + arrRowsSize); Print("Row remove: " + ArrayRemove(arr, 1, 1)); Print("Arr Size is: " + ArraySize(arr)); for(int i=0;i<arrColSize;i++){ arr[2][i] = 0; } Print("Arr Resize is: " + ArrayResize(arr, arrRowsSize-1)); Print("Arr Size is: " + ArraySize(arr)); ArrayPrint(arr); }
Output is attached. It still did not remove the last row, despite using ArrayResize().
int arrColSize = ArraySize(arr)/3;
ArraySize is only for single dimensioned arrays. You need to use ArrayRange - Array Functions - MQL5 Reference - Reference on algorithmic/automated trading language for MetaTrader 5 to get the size of the first dimension (3).
Your array is ArraySize(arr)/4. The second dimension is four.
Seems like an mql5 bug. I will check later with a fresh mind.
ArrayResize: cannot be used for static allocated array.
If ArrayResize() is applied to a static array, a timeseries or an indicator buffer, the array size remains the same – these arrays will not be reallocated. In this case, if new_size<=ArraySize(array), the function will only return new_size; otherwise a value of -1 will be returned.
If ArrayRemove() is used for a fixed-size array, the array size does not change: the remaining "tail" is physically copied to the start position.
It is clear in the documentation. https://www.mql5.com/en/docs/array/arrayresize and https://www.mql5.com/en/docs/array/arrayremove
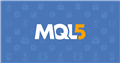
- www.mql5.com
ArrayResize: cannot be used for static allocated array.
If ArrayResize() is applied to a static array, a timeseries or an indicator buffer, the array size remains the same – these arrays will not be reallocated. In this case, if new_size<=ArraySize(array), the function will only return new_size; otherwise a value of -1 will be returned.
It is clear in the documentation. https://www.mql5.com/en/docs/array/arrayresize
Yes you are right, I missed the point that the array is static because it's initialized (and that is not clear in the documentation).
So if you want a dynamic array and initialize it, you need 2 arrays and copy the values.
int INIT[][4]= {{1,2,3,4},{5,6,7,8},{9,10,11,12}}; int arr[][4]; void OnInit() { ArrayCopy(arr,INIT); int arrRowsSize = ArrayRange(arr,0); // will return 3 int arrColSize = ArraySize(arr)/3; Print("arrColSize: " + arrColSize); Print("arrRowsSize: " + arrRowsSize); Print("Row remove: " + ArrayRemove(arr, 1, 1)); Print("Arr Size is: " + ArraySize(arr)); //for(int i=0;i<arrColSize;i++){ // arr[2][i] = 0; //} Print("Arr Resize is: " + ArrayResize(arr, arrRowsSize-1)); Print("Arr Size is: " + ArraySize(arr)); ArrayPrint(arr); }
Output:

- Free trading apps
- Over 8,000 signals for copying
- Economic news for exploring financial markets
You agree to website policy and terms of use
I have attached the code below for your reference.
I am trying to remove the second row.
But I only got the following output:
As shown in the documentation linked https://www.mql5.com/en/docs/array/arrayresize, it should return the size if it is resized, but in this case, i checked ArraySize again and it isn't resized (4x3=12) and when printed, it still shows 3 rows.
How do I remove the 3rd row? Basically I want create a function that removes the rows with all zero's in a 2D array and size it down.