You could use ObjectDeleteAll function to delete all objects from the chart. Like -
// string PREFIX = "Object"; string objName1 = PREFIX + "Yesterday"; string objName2 = PREFIX + "Tomorrow"; string objName3 = PREFIX + "Today"; void OnDeinit(const int reason) { //---- // Delete all objects where PREFIX is used ObjectsDeleteAll(0, PREFIX, 0, -1); //---- }
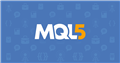
Documentation on MQL5: Object Functions / ObjectsDeleteAll
- www.mql5.com
ObjectsDeleteAll - Object Functions - MQL5 Reference - Reference on algorithmic/automated trading language for MetaTrader 5
Md Atikur Rahman #:
You could use ObjectDeleteAll function to delete all objects from the chart. Like -
Thank you. Will try this.

You are missing trading opportunities:
- Free trading apps
- Over 8,000 signals for copying
- Economic news for exploring financial markets
Registration
Log in
You agree to website policy and terms of use
If you do not have an account, please register
I not able to delete the 3rd object in the OnDeinit function
No matter how I arrange the 3 lines of code, only the last object doesn't get deleted
Any idea why?