Hi I'm kinda new to mql5. i just wanna ask. how to modify stop loss. for example i have 3 open trades w/ same entry prices and same stop loss at first but different tp's, can you suggest how to modify the remaining 2 open trades if tp 1 is closed. thank you.
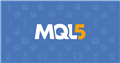
Documentation on MQL5: Constants, Enumerations and Structures / Trade Constants / Trade Operation Types
- www.mql5.com
Trade Operation Types - Trade Constants - Constants, Enumerations and Structures - MQL5 Reference - Reference on algorithmic/automated trading language for MetaTrader 5
- TP modify
- How to close all of your open positions at the same trailing stop price?
- Stop Loss error
Oloy1432:
Hi I'm kinda new to mql5. i just wanna ask. how to modify stop loss. for example i have 3 open trades w/ same entry prices and same stop loss at first but different tp's, can you suggest how to modify the remaining 2 tps if tp 1 is closed. thank you.
Hi I'm kinda new to mql5. i just wanna ask. how to modify stop loss. for example i have 3 open trades w/ same entry prices and same stop loss at first but different tp's, can you suggest how to modify the remaining 2 tps if tp 1 is closed. thank you.
each order should be recognized then you will be able to close/modify, write a program
step 1 - checks pending order and find ticket id of order
step 2 - modify tp from ticket id obtained on step 1
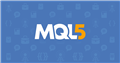
Documentation on MQL5: Trade Functions / OrderGetTicket
- www.mql5.com
OrderGetTicket - Trade Functions - MQL5 Reference - Reference on algorithmic/automated trading language for MetaTrader 5
Oloy1432: same entry prices and same stop loss at first but different tp's, can you suggest how to modify the remaining 2 tps if tp 1 is closed.
Why do you want to modify the TPs? You already set them when you opened.
Oloy1432:
Hi I'm kinda new to mql5. i just wanna ask. how to modify stop loss. for example i have 3 open trades w/ same entry prices and same stop loss at first but different tp's, can you suggest how to modify the remaining 2 tps if tp 1 is closed. thank you.
Hi I'm kinda new to mql5. i just wanna ask. how to modify stop loss. for example i have 3 open trades w/ same entry prices and same stop loss at first but different tp's, can you suggest how to modify the remaining 2 tps if tp 1 is closed. thank you.
Hello, Please see this code, it is perfect to study for what you are trying to do.
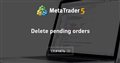
Delete pending orders
- www.mql5.com
Советник-утилита: при появлении позиции удаляет отложенные ордера
Your answers are off-topic. The OP is asking how to modify SL/TP of open positions when one position is closed, not orders (pending).
Alain Verleyen #:
Your answers are off-topic. The OP is asking how to modify SL/TP of open positions when one position is closed, not orders (pending).
Your answers are off-topic. The OP is asking how to modify SL/TP of open positions when one position is closed, not orders (pending).
Ok, here Im posting code example for modification of SL / TP taken from codebase. It will give the Op understanding to modify it further based on requirements.
//+------------------------------------------------------------------+ //| Modify SL TP.mq5 | //| Copyright 2017, MetaQuotes Software Corp. | //| https://www.mql5.com | //+------------------------------------------------------------------+ #property copyright "Copyright 2017, fxMeter" #property link "https://www.mql5.com/en/users/fxmeter" #property version "1.00" #property script_show_inputs #include <Trade\PositionInfo.mqh> #include <Trade\Trade.mqh> #include <Trade\SymbolInfo.mqh> CPositionInfo posi; CTrade trade; CSymbolInfo symb; input double InpStoploss=0.0; //StopLoss Pips input double InpTakeProfit=0.0;//TakeProfit Pips //+------------------------------------------------------------------+ //| Script program start function | //+------------------------------------------------------------------+ void OnStart() { //--- double stoploss=0.0; double takeprofit=0.0; ulong slippage=3; int pt=1; symb.Name(Symbol()); if(!symb.RefreshRates())return; if(symb.Digits()==5 || symb.Digits()==3) pt=10; stoploss=InpStoploss*pt; slippage = slippage * pt; takeprofit = InpTakeProfit * pt; trade.SetDeviationInPoints(slippage); //--- double curBid = symb.Bid(); double slbuy=0.0,slsell=0.0,tpbuy=0.0,tpsell=0.0; if(stoploss>0) { slbuy = curBid - stoploss*symb.Point(); slsell = curBid + stoploss*symb.Point(); } if(takeprofit>0) { tpbuy = curBid + takeprofit*symb.Point(); tpsell = curBid - takeprofit*symb.Point(); } ModifySLTP(slbuy,tpbuy,slsell,tpsell); } //+------------------------------------------------------------------+ void ModifySLTP(double slPriceBuy,double tpPriceBuy,double slPriceSell,double tpPriceSell) { //--- double sl=0.0 ,tp=0.0; bool bslbuy = false,btpbuy = false, bslsell = false, btpsell = false; if(slPriceBuy>0)bslbuy=true; if(tpPriceBuy>0)btpbuy=true; if(slPriceSell>0)bslsell=true; if(tpPriceSell>0)btpsell=true; if(!bslbuy && !btpbuy && !bslsell && !btpsell ) { Print(__FUNCTION__,",No SL/TP need to be modified"); return; } for(int i=PositionsTotal()-1;i>=0;i--) { if(posi.SelectByIndex(i)) { if(posi.Symbol()==Symbol() ) { if(posi.PositionType()==POSITION_TYPE_BUY) { if(bslbuy)sl=slPriceBuy;else sl = posi.StopLoss(); if(btpbuy)tp=tpPriceBuy;else tp = posi.TakeProfit(); trade.PositionModify(posi.Ticket(),NormalizeDouble(sl,Digits()),NormalizeDouble(tp,Digits())); } if(posi.PositionType()==POSITION_TYPE_SELL) { if(bslsell)sl=slPriceSell;else sl = posi.StopLoss(); if(btpsell)tp=tpPriceSell;else tp = posi.TakeProfit(); trade.PositionModify(posi.Ticket(),NormalizeDouble(sl,Digits()),NormalizeDouble(tp,Digits())); } } } } //--- }
Oloy1432 #:
now that's a better word how to call it, im so sorry. im new to this .
now that's a better word how to call it, im so sorry. im new to this .
below codes from codebase as a sample , and you can search yourself for more from codebase as 'breakeven'..
//+------------------------------------------------------------------+ //| Breakeven | //+------------------------------------------------------------------+ void Breakeven() { for(int i=PositionsTotal()-1;i>=0;i--) // returns the number of open positions if(m_position.SelectByIndex(i)) if(m_position.Symbol()==m_symbol.Name() && m_position.Magic()==m_magic) { if(m_position.PositionType()==POSITION_TYPE_BUY) { double level=m_position.PriceOpen()+ m_symbol.NormalizePrice((m_position.TakeProfit()-m_position.PriceOpen())*FiboTral); if(m_position.PriceOpen()>m_position.StopLoss() && level<m_position.PriceCurrent()) { if(!m_trade.PositionModify(m_position.Ticket(), m_position.PriceOpen(), m_position.TakeProfit())) Print("Breakeven ",m_position.Ticket(), " Position -> false. Result Retcode: ",m_trade.ResultRetcode(), ", description of result: ",m_trade.ResultRetcodeDescription()); } } else { double level=m_position.PriceOpen()- m_symbol.NormalizePrice((m_position.PriceOpen()-m_position.TakeProfit())*FiboTral); if(m_position.PriceOpen()<m_position.StopLoss() && level>m_position.PriceCurrent()) { if(!m_trade.PositionModify(m_position.Ticket(), m_position.PriceOpen(), m_position.TakeProfit())) Print("Modify ",m_position.Ticket(), " Breakeven -> false. Result Retcode: ",m_trade.ResultRetcode(), ", description of result: ",m_trade.ResultRetcodeDescription()); } } } }

You are missing trading opportunities:
- Free trading apps
- Over 8,000 signals for copying
- Economic news for exploring financial markets
Registration
Log in
You agree to website policy and terms of use
If you do not have an account, please register