You already know the drill. Show us your code so that so that we can help resolve your issue. Show also the output in the log.
You already know the drill. Show us your code so that so that we can help resolve your issue. Show also the output in the log.
#define TOTAL 5 double vol[TOTAL]= {5,2,4,3,1}; string symbol[TOTAL]= {"A","B","C","D","E"}; //+------------------------------------------------------------------+ //| Script program start function | //+------------------------------------------------------------------+ void OnStart() { //--- ArraySort(vol); for(int i=0; i<TOTAL; i++) { Print(symbol[i]+" "+vol[i]); } }
i want to sort in descending order so 5,4,3,2,1 and also want to make sure symbol names are sorted with vol in output
ArraySort only does ascending order and sorting the "vol" array does not affect the "symbol" array.
If you want them linked, then create a array of structure with both values and then create a sorting method or function for it.
You can also use the Data Collections from the Standard Library, for example CList.
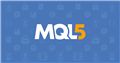
- www.mql5.com
i want to sort in descending order so 5,4,3,2,1 and also want to make sure symbol names are sorted with vol in output
You can use a 2d array and it will sort based on the first index of the 2nd dimension which allows you to keep the index of the symbol on the on the second index .
Then reverse the array and you get the descending order .
#define TOTAL 5 double vol[][2]; double vol_values[]={5,2,4,3,1}; string symbol[]= {"A","B","C","D","E"}; void OnStart() { //fill ArrayResize(vol,TOTAL,0); for(int i=0;i<TOTAL;i++){ vol[i][0]=vol_values[i];//the 0 index will be used in sorting which leaves the [1] index free vol[i][1]=i;//which becomes the index reference } ArraySort(vol); ArrayReverse(vol,0,TOTAL);//reverse for descending order for(int i=0; i<TOTAL; i++) { //get the reference ix to the symbol int ix=(int)vol[i][1]; Print(symbol[ix]+" "+vol[i][0]); } }
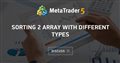
- 2019.09.05
- www.mql5.com

- Free trading apps
- Over 8,000 signals for copying
- Economic news for exploring financial markets
You agree to website policy and terms of use
I want to sort a dynamic array which has value in "double" in either ascending or descending order
When i use ArraySort function it returns invalid values so looking forward for any other method