ZeroMemory(request);
before modifying the request!
No, that is not the solution. The correct solution is for you to fill in every field correctly.
You have left many fields unfilled that are vital for a correct order placement. For example, you have not a filling type, nor a magic number, nor an opening price.
You were just lucky that that it worked with the missing fields set to zero, but that will not always be the case.
All the fields are necessary and need to be properly set according to a specific need.
No, that is not the solution. The correct solution is for you to fill in every field correctly.
You have left many fields unfilled that are vital for a correct order placement. For example, you have not a filling type, nor a magic number, nor an opening price.
You were just lucky that that it worked with the missing fields set to zero, but that will not always be the case.
All the fields are necessary and need to be properly set according to a specific need.
not all fields are mandatory look at the documentation, filling type is mandatory
https://www.mql5.com/en/docs/constants/structures/mqltraderequest
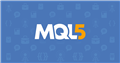
- www.mql5.com
not all fields are mandatory look at the documentation, filling type is mandatory
https://www.mql5.com/en/docs/constants/structures/mqltraderequest
Don't you find it odd that not one of the example orders include request.type_filling ?
It is no wonder that so many people have this problem.
Even when certain fields are not mandatory, they still should be set to prevent unexpected actions, given that their initial values are not known.
For example, imagine a normal "TRADE_ACTION_DEAL" operation where the uninitialised "position ticket" value just so happens to be "correct" and be a valid position in the opposite direction, causing a partial close of that position when in fact you wanted to place a new hedged order instead.
So, even if the documentation does not define it as mandatory, it is a better practice to set all the fields explicitly to prevent any unpredictable mishaps.

- Free trading apps
- Over 8,000 signals for copying
- Economic news for exploring financial markets
You agree to website policy and terms of use
Hello,
I want to make a simple sell but I always receive error. This is my code:
Every time Error have a value 4756!
Every time retcode in the result structure is 10013.
What is my mistake??