Really ? nobody ?
You have a problem with local and global variables: https://www.mql5.com/en/articles/2744
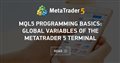
MQL5 Programming Basics: Global Variables of the MetaTrader 5 Terminal
- www.mql5.com
Global variables of the terminal provide an indispensable tool for developing sophisticated and reliable Expert Advisors. If you master the global variables, you will no more be able to imagine developing EAs on MQL5 without them.

You are missing trading opportunities:
- Free trading apps
- Over 8,000 signals for copying
- Economic news for exploring financial markets
Registration
Log in
You agree to website policy and terms of use
If you do not have an account, please register
Hello Everybody, I am still learning when it comes to mql5. I'm trying to code a script that will find support and resistance levels. I'm having problems populating arrays that will hold high values for resistance and low values for support as well as sorting them in ascending order to merge values which are close to each other.
I tried to print the vales of the arrays, It works when It's inside the for loop but doesn't when the print function is outside the for loop, the sort function doesn't seem to be doing it's job but when I create a static array with random values, the sort function works on that array.