Leverage is irrelavant.
Forum on trading, automated trading systems and testing trading strategies
My understanding of leverage so far, please correct me if I am wrong !!
William Roeder, 2017.09.11 15:30
- You don't pay 360 to the broker, he impounds it and releases it when you close the trade.
- As you said, leverage can be dangerous. You shouldn't be even thinking about it. You should be thinking about your risk on a trade. The leverage just allows it. Never risk more than a small percentage of your balance on any single trade.
- In code: Risk depends on your initial stop loss, lot size, and the value of the pair.
- You place the stop where it needs to be - where the reason for the trade is no longer valid. E.g. trading a support bounce the stop goes below the support.
- Account Balance * percent/100 = RISK = OrderLots * (|OrderOpenPrice - OrderStopLoss| * DeltaPerLot + CommissionPerLot) (Note OOP-OSL includes the SPREAD, and DeltaPerLot is usually around $10/pip but it takes account of the exchange rates of the pair vs. your account currency.)
- Do NOT use TickValue by itself - DeltaPerLot
- You must normalize lots properly and check against min and max.
- You must also check FreeMargin to avoid stop out
I have found a way but it seems diferent instruments have diferent leverage so the new question is what in symbol info tell me the leverage
Thank you for the link but i solved the quantity calculation problem. The only problem remaining is how I find the symbol leverage from what is provided? Because in symbol_info there is no such information
https://www.mql5.com/en/docs/constants/environment_state/marketinfoconstants#enum_symbol_calc_mode
Dragos Drimbe #:
Thank you for the link but i solved the quantity calculation problem. The only problem remaining is how I find the symbol leverage from what is provided? Because in symbol_info there is no such information
Thank you for the link but i solved the quantity calculation problem. The only problem remaining is how I find the symbol leverage from what is provided? Because in symbol_info there is no such information
Strange enough, There is no leverage property at symbol level.
Thx but that is for MT5 language. Could be this for Python? https://www.mql5.com/en/docs/integration/python_metatrader5/mt5ordercalcmargin_py
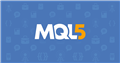
Documentation on MQL5: Integration / MetaTrader for Python / order_calc_margin
- www.mql5.com
order_calc_margin - MetaTrader for Python - Integration - MQL5 Reference - Reference on algorithmic/automated trading language for MetaTrader 5
Dragos Drimbe #:Thx but that is for MT5 language. Could be this for Python? https://www.mql5.com/en/docs/integration/python_metatrader5/mt5ordercalcmargin_py
It is the same thing in python by using the "symbol_info" and I assume it is the "trade_calc_mode" property according to the example code in the documentation. The constants will be the same as the MQL5 documentation.
Thats because leverage is the result of a calculation given by margin values given by the symbol.
Your account has a leverage value, but every symbol can define their own margin parameters, overwriting your accounts leverage.
If you understand the formulas, and you work with some symbols and brokers, you will find out how this works.
It took me quite some time to figure out. But once understood, it will be quite intuitive.
It dosent seems to find a way to get the leverage for the symbol, I have tryied maket_book_get which return none and margin_calc which give something else and it seems no one else knows

You are missing trading opportunities:
- Free trading apps
- Over 8,000 signals for copying
- Economic news for exploring financial markets
Registration
Log in
You agree to website policy and terms of use
If you do not have an account, please register
Hi, I am using python to build an expert advisor. Right now I have a function which calculate the quantity by the investment but dosent take into account the leverage so how I calculate the leverage?
Here is my function:
I am taking an investment of 10% of the account_info.margin_free for testings. I need to add the leverage which is 1:30 with my broker so how do I do this? whats the formula?