I just need a little help to get off the ground. I'm new to MQL5, and I have copied code from here and there. I am missing something in my code.
The code compiles, but the indicator line does not show.
Thanks.
_MAHA, thanks for your reply.
I didn't understand this:
"Optimize and debug your code. Don't loop over precalculated bars unless needed. Prevent your your indicator from such errors as if there isn't enough bars for calculation."
I'm not sure how to give LineBuffer a default value.
(PRICE_OPEN + PRICE_CLOSE) / 2) is supposed to be the Midpoint between the Open and the Close price. I have developed an indicator which draws a line through the middle of each candlestick. Here is the code for that indicatorL
//+------------------------------------------------------------------+ //| Midline01.mq5 | //| Copyright 2011, MetaQuotes Software Corp. | //| https://www.mql5.com | //+------------------------------------------------------------------+ // 2022-07-01 1657 Removed random changing of color, width and style // of the line, and allowed for dynamic changes to // these properties. #property copyright "Copyright 2011, MetaQuotes Software Corp." #property link "https://www.mql5.com" #property version "1.00" #property description "An indicator to show the Midline, or the midpoint between the Open and Close" #property description "It draws a line of a specified color at the Midline" //#property description "Color, width and style of lines is changed randomly" //#property description "after every N ticks" #property indicator_chart_window #property indicator_buffers 1 #property indicator_plots 1 //--- Line properties are set using the compiler directives #property indicator_label1 "Line" // Name of a plot for the Data Window #property indicator_type1 DRAW_LINE // Type of plotting is line #property indicator_color1 clrRed // Line color #property indicator_style1 STYLE_SOLID // Line style #property indicator_width1 1 // Line Width //--- input parameter //input int N=5; // Number of ticks to change input //--- An indicator buffer for the plot double LineBuffer[]; //--- An array to store colors //color colors[]={clrRed,clrBlue,clrGreen}; //--- An array to store the line styles //ENUM_LINE_STYLE styles[]={STYLE_SOLID,STYLE_DASH,STYLE_DOT,STYLE_DASHDOT,STYLE_DASHDOTDOT}; //+------------------------------------------------------------------+ //| Custom indicator initialization function | //+------------------------------------------------------------------+ int OnInit() { //--- Binding an array and an indicator buffer SetIndexBuffer(0,LineBuffer,INDICATOR_DATA); //--- Initializing the generator of pseudo-random numbers // MathSrand(GetTickCount()); //--- return(INIT_SUCCEEDED); } //+------------------------------------------------------------------+ //| Custom indicator iteration function | //+------------------------------------------------------------------+ int OnCalculate(const int rates_total, const int prev_calculated, const datetime &time[], const double &open[], const double &high[], const double &low[], const double &close[], const long &tick_volume[], const long &volume[], const int &spread[]) { // static int ticks=0; //--- Calculate ticks to change the style, color and width of the line // ticks++; //--- If a critical number of ticks has been accumulated // if(ticks>=N) // { // //--- Change the line properties // ChangeLineAppearance(); // //--- Reset the counter of ticks to zero // ticks=0; // } //--- Block for calculating indicator values for(int i=0;i<rates_total;i++) { LineBuffer[i]=(open[i] + close[i]) / 2; // Midpoint is average of Open and Close } //--- Return the prev_calculated value for the next call of the function return(rates_total); } //+------------------------------------------------------------------+ //| Changes the appearance of the drawn line in the indicator | //+------------------------------------------------------------------+ //void ChangeLineAppearance() // { ////--- A string for the formation of information about the line properties // string comm=""; ////--- A block for changing the color of the line ////--- Get a random number // int number=MathRand(); ////--- The divisor is equal to the size of the colors[] array // int size=ArraySize(colors); ////--- Get the index to select a new color as the remainder of integer division // int color_index=number%size; ////--- Set the color as the PLOT_LINE_COLOR property // PlotIndexSetInteger(0,PLOT_LINE_COLOR,colors[color_index]); ////--- Write the line color // comm=comm+(string)colors[color_index]; ////--- A block for changing the width of the line // number=MathRand(); ////--- Get the width of the remainder of integer division // int width=number%5; // The width is set from 0 to 4 ////--- Set the color as the PLOT_LINE_WIDTH property // PlotIndexSetInteger(0,PLOT_LINE_WIDTH,width); ////--- Write the line width // comm=comm+", Width="+IntegerToString(width); ////--- A block for changing the style of the line // number=MathRand(); ////--- The divisor is equal to the size of the styles array // size=ArraySize(styles); ////--- Get the index to select a new style as the remainder of integer division // int style_index=number%size; ////--- Set the color as the PLOT_LINE_COLOR property // PlotIndexSetInteger(0,PLOT_LINE_STYLE,styles[style_index]); ////--- Write the line style // comm=EnumToString(styles[style_index])+", "+comm; ////--- Show the information on the chart using a comment // Comment(comm); // }
Here is the code for my new iindicator, after applying your suggestions. It still does not draw a line. I commented out the lines you said to remove.
//+------------------------------------------------------------------+ //| MAIndicator01.mq5 | //| Copyright 2011, MetaQuotes Software Corp. | //| https://www.mql5.com | //+------------------------------------------------------------------+ // 2022-07-01 1657 MAIndicator01 // Initial version. // Draws a line: // When MAFast is below Midline, -1. // When MAFast is above Midline, +1. #property copyright "Copyright 2011, MetaQuotes Software Corp." #property link "https://www.mql5.com" #property version "1.00" #property description "An indicator to show the Midline, or the midpoint between the Open and Close" #property description "It draws a line of a specified color at the Midline" #property indicator_chart_window #property indicator_buffers 1 #property indicator_plots 1 //--- Line properties are set using the compiler directives #property indicator_label1 "Line" // Name of a plot for the Data Window #property indicator_type1 DRAW_LINE // Type of plotting is line #property indicator_color1 clrRed // Line color #property indicator_style1 STYLE_SOLID // Line style #property indicator_width1 1 // Line Width //--- input parameters input int YellowLinePeriod =2; // Yellow MA trend period //--- indicator handles int m_handle_Midline; // midline indicator handle int m_handle_YellowMA; // moving average indicator handle //--- indicator buffers double m_buff_YellowMA[]; // YellowMA indicator buffer double m_buff_Midline[]; // Midline indicator buffer //--- indicator data for processing double m_Midline_1BarAgo; double m_YellowMA_1BarAgo; //--- An indicator buffer for the plot double LineBuffer[]; //+------------------------------------------------------------------+ //| Custom indicator initialization function | //+------------------------------------------------------------------+ int OnInit() { //--- Binding an array and an indicator buffer SetIndexBuffer(0,LineBuffer,INDICATOR_DATA); ////--- create Midline indicator (White Line) and add it to collection // if(m_handle_Midline==INVALID_HANDLE) // if((m_handle_Midline=(PRICE_OPEN + PRICE_CLOSE) / 2)==INVALID_HANDLE) // { // printf("Error creating Midline indicator"); // return(false); // } ////--- create Exponential Moving Average indicator (Yellow Line) and add it to collection // if(m_handle_YellowMA==INVALID_HANDLE) // if((m_handle_YellowMA=iMA(NULL,0,YellowLinePeriod,0,MODE_EMA,PRICE_CLOSE))==INVALID_HANDLE) // { // printf("Error creating YellowMA indicator"); // return(false); // } ArraySetAsSeries(m_buff_Midline,true); ArraySetAsSeries(m_buff_YellowMA,true); return(INIT_SUCCEEDED); } //+------------------------------------------------------------------+ //| Custom indicator iteration function | //+------------------------------------------------------------------+ int OnCalculate(const int rates_total, const int prev_calculated, const datetime &time[], const double &open[], const double &high[], const double &low[], const double &close[], const long &tick_volume[], const long &volume[], const int &spread[]) { //--- to simplify the coding and speed up access //--- data are put into internal variables CopyBuffer(m_handle_Midline,0,0,2,m_buff_Midline); CopyBuffer(m_handle_YellowMA,0,0,2,m_buff_YellowMA); m_YellowMA_1BarAgo =m_buff_YellowMA[1]; m_Midline_1BarAgo =m_buff_Midline[1]; //--- Block for calculating indicator values for(int i=0;i<rates_total;i++) { if(m_YellowMA_1BarAgo > m_Midline_1BarAgo) LineBuffer[i]=+1; else if(m_YellowMA_1BarAgo < m_Midline_1BarAgo) LineBuffer[i]=-1; else LineBuffer[i]=0; } //--- Return the prev_calculated value for the next call of the function return(rates_total); }
_MAHA, thanks for your reply.
I didn't understand this:
"Optimize and debug your code. Don't loop over precalculated bars unless needed. Prevent your your indicator from such errors as if there isn't enough bars for calculation."
I'm not sure how to give LineBuffer a default value.
(PRICE_OPEN + PRICE_CLOSE) / 2) is supposed to be the Midpoint between the Open and the Close price. I have developed an indicator which draws a line through the middle of each candlestick. Here is the code for that indicatorL
Here is the code for my new iindicator, after applying your suggestions. It still does not draw a line. I commented out the lines you said to remove.
Pick up an indicator source code from codebase or from mql5\indicator\examples folders. See how it is handled and organized. Then apply it in yours.
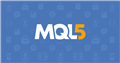
- www.mql5.com
Many thanks to those of you who have replied. I am working diligently to take in all your suggestions. I am reworking the code completely,
as there are certain things that I did not understand before. My goal is to get the program working, and then post the code back here so
that other newbies can benefit from what I have learned.
Thanks. I'll be posting the results here, hopefully in a few days, when I get it working.

- Free trading apps
- Over 8,000 signals for copying
- Economic news for exploring financial markets
You agree to website policy and terms of use
I just need a little help to get off the ground. I'm new to MQL5, and I have copied code from here and there. I am missing something in my code.
The code compiles, but the indicator line does not show.
Thanks.