What's this?
myrequest.tp = myrequest.price + 1 ; myrequest.sl = myrequest.price - 0.5 ;
Why are you asking for ALL history since the creation of the dinosaurs?
if (! HistorySelect ( 0 , TimeCurrent ()))
Why are you looping through ALL trading history since the creation of the dinosaurs?
for ( uint i=total- 1 ; i>= 0 ; i--) //start from the last history
What's this?
Why are you asking for ALL history since the creation of the dinosaurs?
Why are you looping through ALL trading history since the creation of the dinosaurs?
it doesn't matter request.tp and .sl, that works, and for history I guess that's how it should be. it loops from last deal and takes last deal ticket. but last part of EA doesnt work
if(PositionsTotal()<1) { if(dtype==DEAL_TYPE_BUY) { if(reason==DEAL_REASON_TP) OrderSend(myrequest,myresult); if(reason==DEAL_REASON_SL) OrderSend(myrequest1,myresult1); } if(dtype==DEAL_TYPE_SELL) { if(reason==DEAL_REASON_TP) OrderSend(myrequest1,myresult1); if(reason==DEAL_REASON_SL) OrderSend(myrequest,myresult);
You should fix this:
myrequest.tp = myrequest.price + 1 ; myrequest.sl = myrequest.price - 0.5 ;
It is not right.
You don't have to download the ENTIRE story! You must either download a limited story.
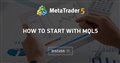
- 2022.04.07
- www.mql5.com
ok this is brilliant and just what i need. The only thing I don't understand is this:
if ( MathRand ()< 32767 / 2 )
But I will research and I will learn. What interests me is whether I can modify it somehow and how to modify it to know if the closed position was buy or sell. If you can still explain it to me, I would be very grateful. Besides, this is brilliant. I want to learn to code like You.

- Free trading apps
- Over 8,000 signals for copying
- Economic news for exploring financial markets
You agree to website policy and terms of use
so the problem is that my EA doesn't work even though there are no errors, if anyone knows what I did wrong and instructs or corrects me, I would be grateful.