Hi guys, I don't know much about coding and I have been asked to do an expert advisor as a graduation project. I have almost finished everything but I need to generate random numbers to test the expert advisor and get the required results so I need to keep generating numbers every test. I really need help regarding this problem, I have found a random number genreator online but I want to know what was the number that has been generated.
- How do I open a trade position
- Need help with drawing arrows, I think I'm having a problem getting the name to re-write?
- Generate 10digits random number without repeating number
senseo:
Hi guys, I don't know much about coding and I have been asked to do an expert advisor as a graduation project. I have almost finished everything but I need to generate random numbers to test the expert advisor and get the required results so I need to keep generating numbers every test. I really need help regarding this problem, I have found a random number genreator online but I want to know what was the number that has been generated.
MathRand
Hi guys, I don't know much about coding and I have been asked to do an expert advisor as a graduation project. I have almost finished everything but I need to generate random numbers to test the expert advisor and get the required results so I need to keep generating numbers every test. I really need help regarding this problem, I have found a random number genreator online but I want to know what was the number that has been generated.
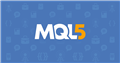
Documentation on MQL5: Math Functions / MathRand
- www.mql5.com
MathRand - Math Functions - MQL5 Reference - Reference on algorithmic/automated trading language for MetaTrader 5
- senseo #: I have already got the random number but i cant know what is it here is the random function
“You have it but can't know what it is,” makes no sense. If you have it you know it.
Your code int RandomNumber; for(int i = 0; i< 1; i++) { RandomNumber = MathRand(); if(RandomNumber > 2000 || RandomNumber < 1000) { i--; }
Simplified int RandomNumber=0; while(RandomNumber > 2000 || RandomNumber < 1000) RandomNumber = MathRand();
William Roeder #:
I meant I can't know what is the random number, for example I start the test the app will generate a random number but that number won't show up in the code obviously so I want to know what is that random number (the output). (I would like to remind you I am not a coder and I don't have much knowledge about coding).
-
“You have it but can't know what it is,” makes no sense. If you have it you know it.
Your code Simplified
This could also be done without a loop.
I think this is more efficient
double RandomNumber = MathRand() / 32767.0;
int result = (int)(RandomNumber * 1000) + 1000;
Dominik Christian Egert #: I think this is more efficient
double RandomNumber = MathRand() / 32767.0;
int result = (int)(RandomNumber * 1000) + 1000;
It is also wrong. MathRand returns an integer in the range [0 … 32767].
- Your code tries to generate equal probably of [0.0 … 0.99999] but also creates a 1/32K chance of a one. The divisor is one short.
- If you want efficient, modulo arithmetic operations rather than int to floating point back to int would be.
Random number generator within range in mql5 - MQL5 programming forum #8 (2019)
Ok, I see the issue with the 1.0.
Because it was asked for a range of 1001 to 1999, right? Well, then I would adjust for the range like this:
int result = (int)(RandomNumber * 998) + 1001;
But I "disagree" to modulo operation, since it gives you a non complete range. What I mean is, the available range of 32767 is not dividable by a pow2 number without a leftover. So you would not get an uniform distribution.
Although the cast between float and int has also a roundoff, the given "granularity" of 32767 should be enough to still hava a fairly uniform distribution for a range of 999 numbers.
Or am I getting this wrong?
The intend was to have a range from 0.0 to 1.0
Edit:
I just checked, the asked range was 1000 to 2000, inclusive. So the first proposed solution is in fact fulfilling the job as intended.
While thinking about it, the chances of "hitting" 0.0 or 1.0 are smaller than the other numbers within the range.
So this needs to be corrected.
int result = (int)(RandomNumber * 1000) + 1000;
Dominik Christian Egert #: Ok, I see the issue with the 1.0. Because it was asked for a range of 1001 to 1999, right? Well, then I would adjust for the range like this:
But I "disagree" to modulo operation, since it gives you a non complete range. What I mean is, the available range of 32767 is not dividable by a pow2 number without a leftover. So you would not get an uniform distribution.
Although the cast between float and int has also a roundoff, the given "granularity" of 32767 should be enough to still hava a fairly uniform distribution for a range of 999 numbers. Or am I getting this wrong?
The intend was to have a range from 0.0 to 1.0
Edit: I just checked, the asked range was 1000 to 2000, inclusive. So the first proposed solution is in fact fulfilling the job as intended.
- You are truncating your value instead of rounding it, which causes a shift towards the floor value, hence why William mentioned 0 ... 0.999, instead of 0 ... 1.000. This will cause the probability of the last value in the range to have a lower probability of coming up than the rest of the values in the range. However, rounding is also not ideal, as the probability of the first and last elements of the range, also have a lower probability of coming up compared to the other values.
- Assuming a uniformly distributed random number generator, then the modulus alternative will also be uniformly distributed.
Yes, I agree to point 1. And see that issue as well, which I haven't taken into consideration.
But I disagree about modulo operator.
Short, theoretical example: random numbers between 1 and 10, modulo by 8.
You will see more of 1s and 2s..
Your range must be a multiple of the modulo operator. Which is not possible with 32767 being the biggest number in the range.
I need a computer to verify this.... Ha.
But, MathRand() % 5 will not uniformly distribute.

You are missing trading opportunities:
- Free trading apps
- Over 8,000 signals for copying
- Economic news for exploring financial markets
Registration
Log in
You agree to website policy and terms of use
If you do not have an account, please register