Hei MQL5 Community,
I've been working on two entry conditions for my EA. I am still new to coding and would appreciate your assistance in providing your expertise where my code lacks the necessary conditions.
The Strategy:
1. The MA filter check is a Boolean that can be either true or false.
2. As with point 1, the CCI filter check is also a Boolean condition.
When both are set to true, I want to use both at the same time to confirm entry.
Your assistance with this task is greatly appreciated.
if((remain==1 && Bid<maVal) || (!MAFilter || CCIFilter == false)) { //here we are assuming that the TakeProfit and StopLoss are entered in Pips ticket = OrderSend(Symbol(), OP_SELL, Lots, Bid, vSlippage, Ask+StopLoss*vPoint, Ask-TakeProfit*vPoint, "Set by Advisor", Magic,0,Blue); if(ticket < 0) { comment = comment + " " + "Error Sending SELL Order"; } else { comment = comment + " " + "SELL Order Executed Successfully"; } }
You're not really doing anything with the MAFilter and CCFilter booleans other than checking if the user has them set to true / false, and using that to override the first part of your if-statement condition if the first half evaluates to false.
Also, as of right now, if both MAFilter and CCFilter are set to true, then that section of your if-statement ( (!MAFilter || CCFilter == false) ) is false.
I'm guessing you want it to be like:
1. User has option to validate the signal further by checking MA and CCI.
2. So, if MAFilter == true OR CCIFilter == true, run a check for them before proceeding with opening an order.
If that's the case, then you should remove the check for the booleans and the filters in the following if-statement:
if((remain==1 && Bid<maVal) || (!MAFilter || CCIFilter == false))
This way, the first half of the if-statement must be true before proceeding.
This is how I would re-write the logic according to how I interpreted your intentions.
const bool isValidSignal = remain==1 && Bid < maVal; if (isValidSignal) { if (MAFilter) { //--- run check on MAFilter, exit using "return" statement if it doesn't validate the signal } if (CCIFilter) { //--- run check on CCIFilter, exit using "return" statement if it doesn't validate the signal } //--- Everything checks out, send order }You should also consider placing blocks of code inside functions. This will help keep things organized and make it easier to debug. Right now, most of your code is inside the OnTick function.
I would really appreciate you showing me how I must implement the return condition as it is leaving me in the mist, and have been trying to pass this condition with no success.
The return operator allows you to exit a function prematurely.
void greeting(string message) { if (message == "") { return; //--- no reason to continue further } Print(greeting); }
In cases where you created a function that is of a type other than "void", the return operator is required.
int add(int a, int b) { return a + b; //--- returns an int }
Here's the documentation for the return operator.
In your case, I would create two functions, one for MAFilterCheck and one for CCIFilterCheck.
bool MAFilterCheck() { const bool condition = ... //--- enter your MA condition if (condition) return true; return false; } bool CCIFilterCheck() { const bool condition = ... //--- enter your CCI condition if (condition) return true; return false; } //--- this is from the code I posted before: const bool isValidSignal = remain==1 && Bid < maVal; if (isValidSignal) { if (MAFilter) { const bool isMAFilterValid = MAFilterCheck(); //--- if false if (!isMAFilterValid) { return; } } if (CCIFilter) { const bool isCCIFilterValid = CCIFilterCheck(); //--- if false if (!isCCIFilterValid) { return; } } //--- Everything checks out, send order }EDIT: since you're a beginner, I figure the use of "const" should be explained. The keyword const means that the value stored in the variable cannot be changed any further once the variable has been initialized. It has some advantages and is always good practice to declare variables with the const keyword if you know for certain that there's no reason that the value needs to be changed.
The return operator allows you to exit a function prematurely.
In cases where you created a function that is of a type other than "void", the return operator is required.
Here's the documentation for the return operator.
In your case, I would create two functions, one for MAFilterCheck and one for CCIFilterCheck.
EDIT: since you're a beginner, I figure the use of "const" should be explained. The keyword const means that the value stored in the variable cannot be changed any further once the variable has been initialized. It has some advantages and is always good practice to declare variables with the const keyword if you know for certain that there's no reason that the value needs to be changed.Do I add these functions at the start of my "Check MA" replacing the current code before my order send function?
if((remain ==0 && Ask > maVal) { //here we are assuming that the TakeProfit and StopLoss are entered in Pips ticket = OrderSend(Symbol(), OP_BUY, Lots, Ask, vSlippage, Bid-StopLoss*vPoint, Bid+TakeProfit*vPoint, "Set by Advisor", Magic,0,Blue); if(ticket < 0) ----------------------------------------------------------------------- if((remain==1 && Bid<maVal) { //here we are assuming that the TakeProfit and StopLoss are entered in Pips ticket = OrderSend(Symbol(), OP_SELL, Lots, Bid, vSlippage, Ask+StopLoss*vPoint, Ask-TakeProfit*vPoint, "Set by Advisor", Magic,0,Blue); if(ticket < 0)
Functions are placed on a global scope.
The function calls to further validate the signal should be placed in the if-statement where the signal first becomes valid.
Hei Alexander,
I see, I however get the following errors for the "if" statement: 'if' - semicolon expected for these two parts of the code. The error remains in effect even if I do add the expected object.
bool MAFilterCheck() { const bool condition = ... //--- enter your MA condition if (condition) return true; return false; } bool CCIFilterCheck() { const bool condition = ... //--- enter your CCI condition if (condition) return true; return false; }
Hei Alexander,
I see, I however get the following errors for the "if" statement: 'if' - semicolon expected for these two parts of the code. The error remains in effect even if I do add the expected object.
Share that section of the code you have so we can see exactly what's going on.
Also
const bool condition = ... //--- enter your MA condition
const bool condition = ... //--- enter your CCI condition
You entered the condition for each function, right?
Share that section of the code you have so we can see exactly what's going on.
Also
You entered the condition for each function, right?
bool MAFilterCheck() { const bool maVal = iMA(Symbol(),0, MA_Period,MA_Shift,MA_Method,MA_Price,1); //--- enter your MA condition if (Close[0] > maVal || Close[0] < maVal) return true; return false; } bool CCIFilterCheck() { const bool isTradeAllowed() = ... //--- enter your CCI condition if (LastOpenTime>=Time[0] - Period()*60) return true; return false; }
However, I'm not certain of the settings. The conditioned layout has significantly altered the simplified layout. I'd appreciate some assistance with this part.
I tried again, and this is what I came up with.
Now the difficult part is with the "if" statements: semicolon expected
And the "if" statements preceding my entry conditions are also confusing me as to whether they should be there.
bool MAFilterCheck() { const bool maVal = iMA(Symbol(),0, MA_Period,MA_Shift,MA_Method,MA_Price,1) //--- enter your MA condition if (Close[0] > maVal && Close [0] <= maVal) return true; return false; } bool CCIFilterCheck() { const bool CheckFilters = iCCI(Symbol(),_Period,50,PRICE_TYPICAL,0)>iCCI(Symbol(),_Period,50,PRICE_TYPICAL,1) //--- enter your CCI condition if (x1>-100 && x2<=-100 && x1<100 && x2>=100) return true; return false; } //+------------------------------------------------------------------+ //| Expert initialization function | //+------------------------------------------------------------------+ int OnInit() { //--- // Detect 3/5 digit brokers for Point and Slippage myPoint = GetPipPoint(Symbol()); if(Point == 0.00001) { vPoint = 0.0001; vSlippage = Slippage *10;} else { if(Point == 0.001) { vPoint = 0.01; vSlippage = Slippage *10;} else vPoint = Point; vSlippage = Slippage; if(TrailingStop && TrailingStart <= 0) { Alert("Parameters incorrect"); return(INIT_PARAMETERS_INCORRECT); } } { Print("Free margin for 1 lot=",MarketInfo(Symbol(), MODE_MARGINREQUIRED)); Print("Freezing Distance[pts]=",MarketInfo(Symbol(), MODE_FREEZELEVEL)); Print("Min Allowed Distance[pts]=",MarketInfo(Symbol(), MODE_STOPLEVEL)); Print("MODE_LOTSIZE = ", MarketInfo(Symbol(), MODE_LOTSIZE)); Print("MODE_MINLOT = ", MarketInfo(Symbol(), MODE_MINLOT)); Print("MODE_LOTSTEP = ", MarketInfo(Symbol(), MODE_LOTSTEP)); Print("MODE_MAXLOT = ", MarketInfo(Symbol(), MODE_MAXLOT)); } //--- return(INIT_SUCCEEDED); } double x1,x2; //+------------------------------------------------------------------+ //| Tick Functions | //+------------------------------------------------------------------+ void OnTick() { bool EnterOpenBar = true; static bool OpenBar=true; static int ticket = 0; string comment; setTrailingStop(Magic); double maVal = iMA(Symbol(),0, MA_Period,MA_Shift,MA_Method,MA_Price,1); if(Hour() == StartHour) { comment = GetDateAndTime(); if(OpenBar == true) { OpenBar=false; if(iVolume(NULL,0,0)>1) //FindTicket makes sure that the EA will pick up its orders //even if it is relaunched ticket = FindTicket(Magic); bool res; res = OrderSelect(ticket, SELECT_BY_POS,MODE_TRADES); if(res == true) { if(OrderCloseTime() == 0) { bool res2; res2 = OrderClose(ticket, Lots, OrderClosePrice(), 10); if(res2 == false) { Alert("Error Closing Order #", ticket); } } } if(Open[0] < Open[StartHour] - MinPipLimit*vPoint) //v3.0 { // && All Conditions True // || 1 Condition True //check ma if(Close[0] > maVal || MAFilter == false) { //here we are assuming that the TakeProfit and StopLoss are entered in Pips ticket = OrderSend(Symbol(), OP_BUY, Lots, Ask, vSlippage, Bid-StopLoss*vPoint, Bid+TakeProfit*vPoint, "Set by Process Expert Advisor", Magic,0,Blue); if(ticket < 1) { comment = comment + " " + "Error Sending BUY Order"; } else { comment = comment + " " + "BUY Order Executed Succesfuly"; } } else { comment = comment + " " + "Reason Order Not Opened: MA Filter Not Passed"; } } else if(Open[0] > Open[StartHour] + MinPipLimit*vPoint) //v3.0 { //check ma if(Close[1] < maVal || MAFilter == false) { //here we are assuming that the TakeProfit and StopLoss are entered in Pips ticket = OrderSend(Symbol(), OP_SELL, Lots, Bid, vSlippage, Ask+StopLoss*vPoint, Ask-TakeProfit*vPoint, "Set by Process Expert Advisor", Magic,0,Blue); if(ticket < 1) { comment = comment + " " + "Error Sending SELL Order"; } else { comment = comment + " " + "SELL Order Executed Succesfuly"; } } else { comment = comment + " " + "Reason Order Not Opened: MA Filter Not Passed"; } } else { comment = comment + " " + "Reason Order Not Opened: MinPipLimit Filter Not Passed"; } Comment(comment); Print(comment); } } else { OpenBar = true; } const bool isValidSignal = Close[0] && Bid < maVal; if (isValidSignal) { if (MAFilter) { const bool isMAFilterValid = MAFilterCheck(); //--- if false if (!isMAFilterValid) { return; } } if (CCIFilter) { const bool isCCIFilterValid = CCIFilterCheck(); //--- if false if (!isCCIFilterValid) { return; } } //--- Everything checks out, send order } } //+------------------------------------------------------------------+ //| Magic Munber | //+------------------------------------------------------------------+ int FindTicket(int M) { int ret = 0; int total = OrdersTotal(); for(int i = 0 ; i < total ; i++) { bool res; res = OrderSelect(i, SELECT_BY_POS,MODE_TRADES); if(res == true) { if(OrderMagicNumber() == M) { ret = OrderTicket(); break; } } } return(ret); } //+------------------------------------------------------------------+ //| Time Function | //+------------------------------------------------------------------+ string GetDateAndTime() { return(string(Year())+"-"+StringFormat("%02d",Month())+"-"+StringFormat("%02d",Day())+" "+StringFormat("%02d",Hour())+":"+StringFormat("%02d",Minute())); }
Here's your first issue:
const bool isTradeAllowed() = ... //--- enter your CCI condition
1. You're using a function call (isTradeAllowed()) as a variable name. Change that to a valid variable name.
2. You haven't defined a valid bool condition (...). The three dots were meant to signify that you need to enter your condition for a valid CCIFilterCheck, whatever that may be.
Another issue:
if (Close[0] > maVal || Close[0] < maVal)
This is going to be true 99.9% of the time, which is probably not what you want; it's very rare for Close[0] to equal maVal.
If you can describe in detail what it is what you want to accomplish, we can help you out.
--------------------------------
EDIT: Just saw your other post.
These two variables (x1, x2) are not initialized in the code you posted.
double x1,x2;
Because they're not initialized, this will give you random results.bool CCIFilterCheck() { const bool CheckFilters = iCCI(Symbol(),_Period,50,PRICE_TYPICAL,0)>iCCI(Symbol(),_Period,50,PRICE_TYPICAL,1) //--- enter your CCI condition if (x1>-100 && x2<=-100 && x1<100 && x2>=100) return true; return false; }
The following is an invalid boolean condition:
const bool CheckFilters = iCCI(Symbol(),_Period,50,PRICE_TYPICAL,0)>iCCI(Symbol(),_Period,50,PRICE_TYPICAL,1) //--- enter your CCI condition
iCCI returns a double value:
double iCCI( string symbol, // symbol int timeframe, // timeframe int period, // averaging period int applied_price, // applied price int shift // shift );
You're missing a semi-colon at the end of the following statement.
bool MAFilterCheck() { const bool maVal = iMA(Symbol(),0, MA_Period,MA_Shift,MA_Method,MA_Price,1) //--- enter your MA condition if (Close[0] > maVal && Close [0] <= maVal) return true; return false; }
Also, iMA returns a double value, not a boolean value.
The following code is executed AFTER your order has been sent, which is wrong:
const bool isValidSignal = Close[0] && Bid < maVal; if (isValidSignal) { if (MAFilter) { const bool isMAFilterValid = MAFilterCheck(); //--- if false if (!isMAFilterValid) { return; } } if (CCIFilter) { const bool isCCIFilterValid = CCIFilterCheck(); //--- if false if (!isCCIFilterValid) { return; } } //--- Everything checks out, send order }Since this code further validates a signal, you want to place it after a signal is valid, but before the order is sent.
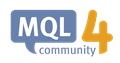
- docs.mql4.com
int _Sell = -1; int _Buy = 1; int LastOpenTime = 0; { int signal = signal_cci(); if (!CheckFilters(signal) && isUseFilters) return; if (isTradeAllowed()){ if(signal== _Buy) { buy(); } if(signal== _Sell){ sell(); } } } int signal_cci() { // CCI crossing 100 level - buy; crossing -100 level - sell enter market double x1,x2; x1 = iCCI(Symbol(),_Period,50,PRICE_TYPICAL,0); x2 = iCCI(Symbol(),_Period,50,PRICE_TYPICAL,1); if(x1>-100 && x2<=-100) return _Sell; if(x1<100 && x2>=100) return _Buy; return 0; } extern bool isUseFilters = true; bool CheckFilters (int signal) { //true - you can trade if(signal==_Buy) { return iATR(NULL,_Period,14,0)<0.0002; } if(signal==_Sell) { return iATR(NULL,_Period,14,0)<0.0002; } return true; } //--------------------------------isTradenAllowed-------------------------------- bool isTradeAllowed() { if (LastOpenTime>=Time[0] - Period()*60) return false; // Do not open order twice on the same bar return true; }Alexander Martinez #:
Here's your first issue:
1. You're using a function call (isTradeAllowed()) as a variable name. Change that to a valid variable name.
2. You haven't defined a valid bool condition (...). The three dots were meant to signify that you need to enter your condition for a valid CCIFilterCheck, whatever that may be.
Another issue:
This is going to be true 99.9% of the time, which is probably not what you want; it's very rare for Close[0] to equal maVal.
If you can describe in detail what it is what you want to accomplish, we can help you out.
Hei Alexander Martinez,
I appreciate your patience in answering my questions. Before entering a trade, I want to confirm two conditions by looking for both the MA and the CCI to give a confirmed signal on either bullish momentum or bearish conditions.
With this in mind, I'd like to be able to set these in my EA's Settings as "true" or "false."
I hope my idea is clear for your support with this plan
The code attached is what I'm trying to implement in my EA

- Free trading apps
- Over 8,000 signals for copying
- Economic news for exploring financial markets
You agree to website policy and terms of use
Hei MQL5 Community,
I've been working on two entry conditions for my EA. I am still new to coding and would appreciate your assistance in providing your expertise where my code lacks the necessary conditions.
The Strategy:
1. The MA filter check is a Boolean that can be either true or false.
2. As with point 1, the CCI filter check is also a Boolean condition.
When both are set to true, I want to use both at the same time to confirm entry.
Your assistance with this task is greatly appreciated.