- Help, I don't know how to open trade based on minute.
- Please HELP!! with this EA
- Time filters, ETC.
Is there anyone in this group who can help me. I want my EA to open trades only at certain hours (not all the time). For example: I want the EA to be able to open trades only at 10.00 to 15.00
I use the following settings in my Expert Advisors:
input group "Time control" input bool InpTimeControl = true; // Use time control input uchar InpStartHour = 10; // Start Hour input uchar InpStartMinute = 01; // Start Minute input uchar InpEndHour = 15; // End Hour input uchar InpEndMinute = 02; // End Minute
more details can be seen in the article ( An attempt at developing an EA constructor for MetaTrader 5 ) and updated code (Trading engine 4 )
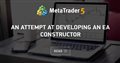
- www.mql5.com
I use the following settings in my Expert Advisors:
more details can be seen in the article ( An attempt at developing an EA constructor for MetaTrader 5 ) and updated code (Trading engine 4 )
Does this command work right after entering into the list of EA codes?
***Those are just parameters. They will have no effect on the EA at all until you actually implement the logic inside the EA code. You have to program that logic yourself of hire someone to do it for you.
@Vladimir Karputov gave you links for an article giving some explanation on to code it yourself, but if you have no idea about your EA's logic then you will have to take some time to learn.
Code:
string StartTime = "10:00";
string EndTime = "15:00";
if (TimeCurrent() >= StringToTime(TimeToString(TimeCurrent(),TIME_DATE)+" "+StartTime) && TimeCurrent() < StringToTime(TimeToString(TimeCurrent(),TIME_DATE)+" "+EndTime))
{
// do something here
}
should work on MT4 and MT5.
Please note: You are on the MQL5 forum.
You mixed up the section - you showed an example of the old code (The old terminal is discussed here: MQL4 and MetaTrader 4 ).
Yes! You will have to program that functionality in your EA, to read the data from the calender and act accordingly. However, given that you have shown not to know how to program a simple time filter, it will be much to complex for you to code yourself. It may be best to hire someone in the Freelance section.
string StartTime = "10:00";
string EndTime = "15:00";
if (TimeCurrent() >= StringToTime(TimeToString(TimeCurrent(),TIME_DATE)+" "+StartTime) && TimeCurrent() < StringToTime(TimeToString(TimeCurrent(),TIME_DATE)+" "+EndTime))
{
// do something here
}
When dealing with time, a lot of people use strings; they can not be optimized. Using (int) seconds or (double) hours and minutes can be.
See also Dealing with Time (Part 1): The Basics - MQL5 Articles (2021.10.01)
Dealing with Time (Part 2): The Functions - MQL5 Articles (2021.10.08)
ahuh
input int StartHour = 10; input int StartMinute = 00; input int EndHour = 15; input int EndMinute = 00; if (TimeCurrent() >= StringToTime(TimeToString(TimeCurrent(),TIME_DATE)+" "+StartHour+":"+StartMinute) && TimeCurrent() < StringToTime(TimeToString(TimeCurrent(),TIME_DATE)+" "+EndHour+":"+EndMinute) ) { // do something here }
Another way to do this
input string StartTime = "01:00"; // Start Trading Time input string EndTime = "23:00"; // End Trading Time static MqlDateTime Time; int OnInit() { /* Your code... */ TimeToStruct(TimeCurrent(), Time); return(INIT_SUCCEEDED); } // Trading time checking function bool IsTradingTime() { TimeCurrent(Time); string start[]; string end[]; StringSplit(StartTime, StringGetCharacter(":", 0), start); StringSplit(EndTime, StringGetCharacter(":", 0), end); int timeNow = Time.hour * 60 + Time.min; int timeStart = (int)start[0] * 60 + (int)start[1]; int timeEnd = (int)end[0] * 60 + (int)end[1]; return (timeNow >= timeStart && timeNow <= timeEnd); }

- Free trading apps
- Over 8,000 signals for copying
- Economic news for exploring financial markets
You agree to website policy and terms of use