Hello, can anyone fix this code? I'm trying to change it from send email alert to send a push notification. Thanks!
And what is the problem?
Check your terminal: Ctrl+O => (tab) Notifications.
Have you got and do you know your MQ-ID? Have you read: https://www.mql5.com/en/articles/476.
Have you checked you logs? What do they tell you?
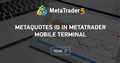
- www.mql5.com
-
Why did you post your MT4 question in the MT5 General section instead of the MQL4 section, (bottom of the Root page)?
General rules and best pratices of the Forum. - General - MQL5 programming forum?
Next time, post in the correct place. The moderators will likely move this thread there soon. -
Perhaps you should read the manual. SendNotification - Common Functions - MQL4 Reference
How To Ask Questions The Smart Way. (2004)
How To Interpret Answers.
RTFM and STFW: How To Tell You've Seriously Screwed Up.Your code Documentation SendNotification( "ADX Line PlusDI has snapped", "ADX Line PlusDI has snapped at Ask=… );
bool SendNotification( string text // Text of the notification );
Check your terminal: Ctrl+O => (tab) Notifications. Checked, everything okay.
Have you got and do you know your MQ-ID? Have you read: https://www.mql5.com/en/articles/476. Absolutely, been using the same ID for years, receiving all other alerts no problem.
Have you checked you logs? What do they tell you? Nothing to see in logs, problem is with the code.
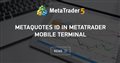
- www.mql5.com
Why did you post your MT4 question in the MT5 General section instead of the MQL4 section, (bottom of the Root page)?
General rules and best pratices of the Forum. - General - MQL5 programming forum?
Next time, post in the correct place. The moderators will likely move this thread there soon.

- Free trading apps
- Over 8,000 signals for copying
- Economic news for exploring financial markets
You agree to website policy and terms of use
Hello, can anyone fix this code? I'm trying to change it from send email alert to send a push notification. Thanks!