Tick Size and Point Size can be very different especially on stocks and other symbols besides forex.
Always use Tick Size to adjust and align your prices, not the point size. In essence, make sure that your price quotes, are properly aligned to the Tick size (see following examples).
... double tickSize = SymbolInfoDouble( _Symbol, SYMBOL_TRADE_TICK_SIZE ); ... double normalised_price = round( price / tick_size ) * tick_size; ... // Or use a function double Round2Ticksize( double price ) { double tick_size = SymbolInfoDouble( _Symbol, SYMBOL_TRADE_TICK_SIZE ); return( round( price / tick_size ) * tick_size ); };
Tick Size and Point Size can be very different especially on stocks and other symbols besides forex.
Always use Tick Size to adjust and align your prices, not the point size. In essence, make sure that your price quotes, are properly aligned to the Tick size (see following examples).
That is starting to make sense, but don't you think dividing the number by a number let say x then multiplying the result by the same number x, does not make sense mathematically, the result will always be the same as the original number (what you did)
( price / tick_size ) * tick_size
maybe the round function will make the difference there which I'm not sure the result may be very accurate
PIP, Point, or Tick are all different in general.
What is a TICK? - MQL4 programming forum (2014)
Unless you manually adjust your SL/TP for each separate symbol, using Point means code breaks on 4 digit brokers, exotics (e.g. USDZAR where spread is over 500 points), and metals. Compute what a PIP is and use it, not points.
How to manage JPY pairs with parameters? - MQL4 programming forum (2017)
Slippage defined in index points - Expert Advisors and Automated Trading - MQL5 programming forum (2018)
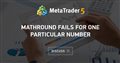
- 2017.12.31
- www.mql5.com
PIP, Point, or Tick are all different in general.
What is a TICK? - MQL4 programming forum (2014)
Unless you manually adjust your SL/TP for each separate symbol, using Point means code breaks on 4 digit brokers, exotics (e.g. USDZAR where spread is over 500 points), and metals. Compute what a PIP is and use it, not points.
How to manage JPY pairs with parameters? - MQL4 programming forum (2017)
Slippage defined in index points - Expert Advisors and Automated Trading - MQL5 programming forum (2018)
Thanks for the response but, from my case this remains constant. I have already normalized my stoploss and tp to fit the digits brokers but regardless of this there is always a moment where point() will be handy when calculating the trading targets, so would you use between a tick size or point
Thanks for the response but, from my case this remains constant. I have already normalized my stoploss and tp to fit the digits brokers but regardless of this there is always a moment where point() will be handy when calculating the trading targets, so would you use between a tick size or point
Always normalise your prices to the tick size, not to point nor the number of "digits". And Yes, I do everything aligned to the tick size not the point size ...
Forum on trading, automated trading systems and testing trading strategies
Fernando Carreiro, 2017.09.23 00:41
Just as a side note.
Initially, when I started with MQL, I would manipulate prices as "doubles". Nowadays, especially in the more complex EA's, I manipulate prices as "ints". At the very first opportunity, I convert a price into ticks:
int priceTicks = (int) round( price / tickSize );From then on, all my calculations and manipulations are done with "ints". Not only is it more memory compact and much faster, but comparisons are much easier to handle. Doing a "priceA == priceB" for "doubles" is quite problematic, but not for "ints" because it gives exact matches. Not to mention, that in this way prices, stop sizes, etc. are ALWAYS aligned.
Then, just before I have to place or modify an order, I then convert it back:
priceTicks * tickSizeEDIT: I do the same for volume/lots by using the broker's Lot-Step.
Forum on trading, automated trading systems and testing trading strategies
How to calculate lots using multiplier according to number of opened orders?
Fernando Carreiro, 2017.09.01 21:57
Don't use NormalizeDouble(). Here is some guidance (code is untested, just serves as example):
// Variables for Symbol Volume Conditions double dblLotsMinimum = SymbolInfoDouble( _Symbol, SYMBOL_VOLUME_MIN ), dblLotsMaximum = SymbolInfoDouble( _Symbol, SYMBOL_VOLUME_MAX ), dblLotsStep = SymbolInfoDouble( _Symbol, SYMBOL_VOLUME_STEP ); // Variables for Geometric Progression double dblGeoRatio = 2.8, dblGeoInit = dblLotsMinimum; // Calculate Next Geometric Element double dblGeoNext = dblGeoInit * pow( dblGeoRatio, intOrderCount + 1 ); // Adjust Volume for allowable conditions double dblLotsNext = fmin( dblLotsMaximum, // Prevent too greater volume fmax( dblLotsMinimum, // Prevent too smaller volume round( dblGeoNext / dblLotsStep ) * dblLotsStep ) ); // Align to Step value
Tick Size and Point Size can be very different especially on stocks and other symbols besides forex.
Always use Tick Size to adjust and align your prices, not the point size. In essence, make sure that your price quotes, are properly aligned to the Tick size (see following examples).
Oh well, it seems I too am bumping into this issue... That's a good call indeed, Fernando, however how do you handle situations where the tick size / tick value in the broker's symbol specs is zero?
Sometimes the values apear as zero in the symbol's contract specifications presented by MetaTrader, but not when using the MQL functions to retrieve those values. So, make sure of this first.
However, should those values still be zero, then the broker is not being reliable. Contact them and have them fix the issue. If they refuse to do that or keep stalling, then change your broker. They are not reliable.
Sometimes the values apear as zero in the symbol's contract specifications presented by MetaTrader, but not when using the MQL functions to retrieve those values. So, make sure of this first.
However, should those values still be zero, then the broker is not being reliable. Contact them and have them fix the issue. If they refuse to do that or keep stalling, then change your broker. They are not reliable.
You're right again, Fernando... I was starting to suspect that myself and was in the middle of coding a script to confirm these discrepancies -- attached if others find it useful to gain insights on details of different symbols, and also compare them across brokers.
Another question, in the meantime -- why is the tick value for profitable positions differ from losing ones? I haven't had time yet to delve deeper into this, but any answers or pointers appreciated.

- Free trading apps
- Over 8,000 signals for copying
- Economic news for exploring financial markets
You agree to website policy and terms of use
is the same as Point() for a specific symbol,100% though I'm not sure why they are being called and defined as different things on the docs, but the point I'm trying to make is that using Point() to calculate stoplosses and takeprofits could lead to Inaccurate results when dealing with multicurrency EA's that work on different symbols and instruments
Since point() Returns the point size of the current symbol in the quote currency. Any thought or doubts about that, take a look at the following case that occurred to me recently