For the first trade the lot size should be 0.01 for the second 0.03 for the third 0.08 for the forth 0.22 for the fifth 0.61 and so on.
I know if I use a variable to keep last order's lot size I can achieve this using multiplier of 2.8. But you know I want to use as less as possible variables.
So if I have 3 opened orders so far for instance if I want to get for the forth order lot size I should multiply 4 by 0.01 by 2.8 but it equals to 0.112 which is not correct.
I'm a little confused.
So please help.
And so are we! It will be helpful if show some code so that we can understand what it is you want to achieve.
And for the math, some algebra might help as well explain your logic!
0.01, 0.03, 0.08, 0.22, 0.61 is a geometric series based around a common ratio ("r") of 2.8 as you yourself have stated. So, use the Geometric Sequence basics to calculate the elements, but remember to use rounding or ceiling/floor to get the actual final value.
Also, you should always check the brokers conditions such as Minimum Lot Size, Maximum Lot Size and Lot Step Value to adjust your volume.
PS! There is nothing wrong with using variables and it is highly recommend! They help make your code more readable and believe it or not, can make your code much more efficient and faster.
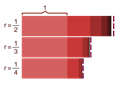
- en.wikipedia.org
And so are we! It will be helpful if show some code so that we can understand what it is you want to achieve.
And for the math, some algebra might help as well explain your logic!
0.01, 0.03, 0.08, 0.22, 0.61 is a geometric series based around a common ratio ("r") of 2.8 as you yourself have stated. So, use the Geometric Sequence basics to calculate the elements, but remember to use rounding or ceiling/floor to get the actual final value.
Also, you should always check the brokers conditions such as Minimum Lot Size, Maximum Lot Size and Lot Step Value to adjust your volume.
PS! There is nothing wrong with using variables and it is highly recommend! They help make your code more readable and believe it or not, can make your code much more efficient and faster.
Thank you.
So it should be:
double nextLotSize=0.01*2.8^OpenedTrades();
The function OpenedTrades() explains itself...
uff... Forgot rounding..
toie: So it should be:
double nextLotSize=0.01*2.8^OpenedTrades();
The function OpenedTrades() explains itself...
No! You must use proper MQL maths notation. In MQL, the "^" symbol is not "raised to the power of". you have to use either "MathsPow()" function or "pow()".
However, it is not that "simple" because you still have to align your volume by the "Lot Step" and check "Lots Minimum" and "Lots Maximum"!
And please use variables. Don't Hard-Code. That is messy coding!
My prefinal result is:
double nextLotSize=NormalizeDouble(0.01*MathPow(2.8,OpenedTrades()),2);
I say prefinal because you mentioned I should check my brokers requirements.
Don't use NormalizeDouble(). Here is some guidance (code is untested, just serves as example):
// Variables for Symbol Volume Conditions double dblLotsMinimum = SymbolInfoDouble( _Symbol, SYMBOL_VOLUME_MIN ), dblLotsMaximum = SymbolInfoDouble( _Symbol, SYMBOL_VOLUME_MAX ), dblLotsStep = SymbolInfoDouble( _Symbol, SYMBOL_VOLUME_STEP ); // Variables for Geometric Progression double dblGeoRatio = 2.8, dblGeoInit = dblLotsMinimum; // Calculate Next Geometric Element double dblGeoNext = dblGeoInit * pow( dblGeoRatio, intOrderCount + 1 ); // Adjust Volume for allowable conditions double dblLotsNext = fmin( dblLotsMaximum, // Prevent too greater volume fmax( dblLotsMinimum, // Prevent too smaller volume round( dblGeoNext / dblLotsStep ) * dblLotsStep ) ); // Align to Step value

- Free trading apps
- Over 8,000 signals for copying
- Economic news for exploring financial markets
You agree to website policy and terms of use
Hello guys.
I'd like to place every consequitive order using multiplier. Now I've hardcoded my prefered lots using an array. I don't like it.
For the first trade the lot size should be 0.01 for the second 0.03 for the third 0.08 for the forth 0.22 for the fifth 0.61 and so on.
I know if I use a variable to keep last order's lot size I can achieve this using multiplier of 2.8. But you know I want to use as less as possible variables.
So if I have 3 opened orders so far for instance if I want to get for the forth order lot size I should multiply 4 by 0.01 by 2.8 but it equals to 0.112 which is not correct.
I'm a little confused.
So please help.
Thanx.