An example for NETTING! The choice of position is by SYMBOL !!!
You need to: get the position ID, then you must get all transactions that refer to this ID. Additionally, you need to summarize the profit of the current position.
//+------------------------------------------------------------------+ //| Profit of a position by trading history.mq5 | //| Copyright © 2021-2022, Vladimir Karputov | //| https://www.mql5.com/en/users/barabashkakvn | //+------------------------------------------------------------------+ #property copyright "Copyright © 2021-2022, Vladimir Karputov" #property link "https://www.mql5.com/en/users/barabashkakvn" #property version "1.001" #property script_show_inputs #include <Trade\PositionInfo.mqh> CPositionInfo m_position; //+------------------------------------------------------------------+ //| Script program start function | //+------------------------------------------------------------------+ void OnStart() { if(m_position.Select(Symbol())) // select the position for further work { double profit=m_position.Profit(); //--- request trade history if(!HistorySelectByPosition(m_position.Identifier())) { Print("Error HistorySelectByPosition"); return; } //--- uint history_deals_total=HistoryDealsTotal(); //--- for all deals for(uint i=0; i<history_deals_total; i++) { ulong ticket=HistoryDealGetTicket(i); if(ticket) { profit+=HistoryDealGetDouble(ticket,DEAL_COMMISSION)+HistoryDealGetDouble(ticket,DEAL_SWAP)+HistoryDealGetDouble(ticket,DEAL_PROFIT); } } PrintFormat("position Ticket %d, position ID %d, profit %+.2f: ",m_position.Ticket(),m_position.Identifier(),profit); } } //+------------------------------------------------------------------+
Now you can get the price of the very first trade and get the difference between it and the position price . And if there is a difference, then it will be easy to make a profit in 'Points'.
Like that:
//+------------------------------------------------------------------+ //| Points profit of a position by trading history.mq5 | //| Copyright © 2022, Vladimir Karputov | //| https://www.mql5.com/en/users/barabashkakvn | //+------------------------------------------------------------------+ #property copyright "Copyright © 2022, Vladimir Karputov" #property link "https://www.mql5.com/en/users/barabashkakvn" #property version "1.003" #property script_show_inputs #include <Trade\PositionInfo.mqh> CPositionInfo m_position; //+------------------------------------------------------------------+ //| Script program start function | //+------------------------------------------------------------------+ void OnStart() { if(m_position.Select(Symbol())) // select the position for further work { double profit=m_position.Profit(); //--- request trade history if(!HistorySelectByPosition(m_position.Identifier())) { Print("Error HistorySelectByPosition"); return; } //--- uint history_deals_total=HistoryDealsTotal(); double price_in=0.0; long time_in=TimeCurrent()+3600*24*3; //--- for all deals for(uint i=0; i<history_deals_total; i++) { ulong ticket=HistoryDealGetTicket(i); if(ticket) { profit+=HistoryDealGetDouble(ticket,DEAL_COMMISSION)+HistoryDealGetDouble(ticket,DEAL_SWAP)+HistoryDealGetDouble(ticket,DEAL_PROFIT); if(HistoryDealGetInteger(ticket,DEAL_ENTRY)==DEAL_ENTRY_IN) { long deal_time=HistoryDealGetInteger(ticket,DEAL_TIME); double deal_price=HistoryDealGetDouble(ticket,DEAL_PRICE); if(deal_time<time_in) { time_in=deal_time; price_in=deal_price; } } } } if(price_in>0.0) { double price_diff=MathAbs(m_position.PriceCurrent()-price_in); int points_profit=(int)(price_diff/Point()); PrintFormat("position Ticket %d, position ID %d, profit %.2f, points profit %d: ", m_position.Ticket(),m_position.Identifier(),profit,points_profit); } } } //+------------------------------------------------------------------+
Result:
2022.01.11 06:57:32.678 Points profit of a position by trading history (XAUUSD,M15) position Ticket 1235269798, position ID 1235269434, profit -0.63, points profit 71:
Thanks. I will study the files you sent.
But there is one thing I don't understand:
According the MQL5 Reference
SYMBOL_TRADE_TICK_VALUE
Value of SYMBOL_TRADE_TICK_VALUE_PROFIT
- SYMBOL_TRADE_TICK_VALUE – one-tick price change value for a profitable position
Why some CFD get SYMBOL_TRADE_TICK_VALUE = 0.1 , but In fact every 1 point value is 1.
Isn't SYMBOL_TRADE_TICK_VALUE the value corresponding to every 1 point fluctuation?
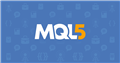
- www.mql5.com
Thanks. I will study the files you sent.
But there is one thing I don't understand:
According the MQL5 Reference
SYMBOL_TRADE_TICK_VALUE
Value of SYMBOL_TRADE_TICK_VALUE_PROFIT
- SYMBOL_TRADE_TICK_VALUE – one-tick price change value for a profitable position
Why some CFD get SYMBOL_TRADE_TICK_VALUE = 0.1 , but In fact every 1 point value is 1.
Isn't SYMBOL_TRADE_TICK_VALUE the value corresponding to every 1 point fluctuation?
Anyone can help me ask this question ?
To calculate the profit in points on a position, my method is:
int Ticket_ProfitPoint( ulong ticket) { if (!PositionInfo.SelectByTicket(ticket)) return ( 0 ); double profit = PositionInfo.Profit(); double volume = PositionInfo.Volume(); double tickvalue = SymbolInfoDouble (PositionInfo. Symbol (), SYMBOL_TRADE_TICK_VALUE ); return (( int )(profit / volume / tickvalue)); });
The profit in points calculated by foreign exchange varieties is correct, but for some CFDs, such as XAUUSD, the result is wrong.
For example, XAUUSD, profit 0.02, position 0.01, tick_value=0.1. Points are calculated as = 20, but actual points = 2.
I want to know what is wrong? Is it wrong to use SYMBOL_TRADE_TICK_VALUE in my formula? Doesn't SYMBOL_TRADE_TICK_VALUE refer to the profit corresponding to a position fluctuation?
======
I know that the profit in points can be calculated from the price difference, but I would like to know where is my formula wrong?
If my formula is wrong, then assuming you know the profit in pips and the size of the position, it is impossible to reverse the profit.
To calculate the profit in points on a position, my method is:
The profit in points calculated by foreign exchange varieties is correct, but for some CFDs, such as XAUUSD, the result is wrong.
For example, XAUUSD, profit 0.02, position 0.01, tick_value=0.1. Points are calculated as = 20, but actual points = 2.
I want to know what is wrong? Is it wrong to use SYMBOL_TRADE_TICK_VALUE in my formula? Doesn't SYMBOL_TRADE_TICK_VALUE refer to the profit corresponding to a position fluctuation?
======
I know that the profit in points can be calculated from the price difference, but I would like to know where is my formula wrong?
If my formula is wrong, then assuming you know the profit in pips and the size of the position, it is impossible to reverse the profit.
search the website, forum god: william roeder has made many posts regarding this.
The function correct when used for Forex calculations, but wrong when used for XAUUSD or CFDs?
I want to turn position profit values to profit points,
How to calculate XAUUSD or CFDs ?
#property copyright "Spase" #property link "https://www.mql5.com" #property version "1.00" // Following code calculates the total profit of the open positions for the current symbol or chart only. // You can change the RunningProfit function not to exclude other symbols. // I am an amateur hobyist coder so my code might not look pretty or make sense to seasoned programers, but hey it works. // I coudn't find a way of including commissions for those who trade with brokers who charge commissions. void OnDeinit(const int reason) { Comment(""); } void OnTick() { double RunningProfitCash = 0; int RunningProfitPips = 0; RunningProfit(RunningProfitCash,RunningProfitPips); Comment ("For Current Symbol: ",_Symbol,"\n", "Running Profit in Cash: $",DoubleToString(RunningProfitCash,2),"\n", "Running Profit in Pips: " ,RunningProfitPips/10,"\n" //Points converted to pips by dividing by 10. Lazy lol ); } void RunningProfit(double &RunningProfitCash , int &RunningProfitPips ) { double Ask=NormalizeDouble(SymbolInfoDouble(_Symbol,SYMBOL_ASK),_Digits); // Calculate the Ask Price double Bid=NormalizeDouble(SymbolInfoDouble(_Symbol,SYMBOL_BID),_Digits);// Calculate the Bid Price double SinglePositionCurrentProfitPips = 0.0; for (int i=PositionsTotal()-1; i>=0; i--)//Go through all the positions { string symbol=PositionGetSymbol(i); //Get the current symbol of each position if (_Symbol==symbol) // Include only positions of current symbol on chart. You can omit this if you want total profit of all positions. { double SinglePositionCurrentProfitCash =PositionGetDouble(POSITION_PROFIT)+ PositionGetDouble(POSITION_SWAP);//Get the position profit in Cash if (PositionGetInteger(POSITION_TYPE)==ORDER_TYPE_SELL) {SinglePositionCurrentProfitPips =PositionGetDouble(POSITION_PRICE_OPEN) - Ask ;//Get the Sell positions profit in price difference } if (PositionGetInteger(POSITION_TYPE)==ORDER_TYPE_BUY) {SinglePositionCurrentProfitPips = Bid-PositionGetDouble(POSITION_PRICE_OPEN); //Get the Buy positions profit price difference } RunningProfitCash = RunningProfitCash + NormalizeDouble(SinglePositionCurrentProfitCash,2); // Adding profits of current symbol positions RunningProfitPips = RunningProfitPips + int (SinglePositionCurrentProfitPips/_Point); // Divide price difference then convert to points. Then add all profits in points }// End If loop }// End for loop RunningProfitCash = RunningProfitCash; RunningProfitPips = RunningProfitPips; }This is a code I made as part of a larger expert. It works for your purpose. Hope you find it useful.
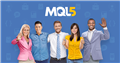
- 2023.01.17
- www.mql5.com
a small modification give real points of profit
int cs = SymbolInfoDouble(Symbol(),SYMBOL_TRADE_CONTRACT_SIZE);
RunningProfitPips = RunningProfitPips + int (SinglePositionCurrentProfitPips * cs );

- Free trading apps
- Over 8,000 signals for copying
- Economic news for exploring financial markets
You agree to website policy and terms of use
The function correct when used for Forex calculations, but wrong when used for XAUUSD or CFDs?
I want to turn position profit values to profit points,
How to calculate XAUUSD or CFDs ?