So you don't think it should be done by cycling back through history? I just thought that given history is as current as the previous bar if the deal has been closed, that there's the complete information contained there, it just needs to be filtered. I see what you're getting at though and maybe you're right, especially if I'm already counting grids and the profit from each, that marker essentially becomes the close coordinates with any trades opened after that being the trigger point for commencement of a new marker.
Hedging, grid trading, same as Martingale.
Martingale, Hedging and Grid : MHG - General - MQL5 programming forum (2016)
Martingale, guaranteed to blow your account eventually. If it's not profitable without, it is definitely not profitable with.
Martingale vs. Non Martingale (Simplified RoR vs Profit and the Illusions) - MQL5 programming forum (2015)
Why it won't work:
Calculate Loss from Lot Pips - MQL5 programming forum (2017)
THIS Trading Strategy is a LIE... I took 100,000 TRADES with the Martingale Strategy - YouTube (2020.12.12)
Hedging, grid trading, same as Martingale.
Martingale, Hedging and Grid : MHG - General - MQL5 programming forum (2016)
Martingale, guaranteed to blow your account eventually. If it's not profitable without, it is definitely not profitable with.
Martingale vs. Non Martingale (Simplified RoR vs Profit and the Illusions) - MQL5 programming forum (2015)
Why it won't work:
Calculate Loss from Lot Pips - MQL5 programming forum (2017)
THIS Trading Strategy is a LIE... I took 100,000 TRADES with the Martingale Strategy - YouTube (2020.12.12)
Hi William, thanks for the links and I'm acutely aware of the risks of pure Martingale, having researched it for many years (I've been researching the topic since 2013).
I knew as soon as I mentioned anything to do with Martingale that this would be the response, so you're welcome to share my honey pot! :)
My strategy is far more complex than just solely a standard "doubling grid" trader, so I'm not too concerned about that. If I wanted just a simple grid trader it would only be 200 lines to code! :) This isn't your normal "run of the mill" sold on MQL5 marketplace for $100 type of robot; it's the culmination of almost 10 years of research and work.
My system uses filters on when to create a grid based on ATR and smoothed MAs, along with only trading less volatile market sessions and times. It also has the option to omit specific trade days and dates, not trade NFP and Thursday before NFP and other extremely volatile days, along with tracking and creating grids based on present ranges rather than solely "dumb" equidistant grids. There are also RSI and Stochastic filters to alert when volatility and momentum are going to extremes.
It uses a "core grid" and "outer grid" system along with a break-even exit strategy for recovery mode should the drawdown go past certain thresholds, basically hedging some losing positions against winning ones of similar amounts of profit vs loss to cancel them out, which keeps drawdown to a threshold which is able to be set in the EA.
It also uses what I term a "collective profit model" to group trades so aside from needing a reasonable balance to handle drawdown, the drawdown is normally less than 3% on account sizes about $5,000 btw, and the system basically never loses a "grid".
As an emergency stop, one can also set a hard limit to close all positions should the drawdown get to an emergency level, e.g. 20+%. With that said, I've even tested it on accounts of only $100 starting balance and it still works, though of course minimum lot sizes make using a grid trading system of any kind hard with so small a balance, though maximum drawdown tends to consistently be around 10-15%.
My system also doesn't seek to make large profits, instead normally being set to a profit target of $0.50 per minimum lot (in most cases 0.01 lot size on most symbols), which is fine when the system trades around 0.03 per grid (so $1.50 per trade) when trading microlots.
Sure, it will lose individual trades within each "group of trades", though it never loses on a "per grid" basis.
As it trades and builds its balance, over time it also has a slowly increasing lot size incrementer so it's not solely a static system.
It also has a profit guard system built with trailing stops and a percentage fluctuation allowance setting (e.g. so if a group of grid positions goes into profit, it trails the entire group and risks a percentage of the profit of that group position to allow for price fluctuations, rather than hard trailing stops) so when a grid does win, it can maximise profitability.
There's also a profit target system built into it that allows setting of daily, weekly and monthly targets, both for fixed dollar and percentage of account balance. If a target is exceeded, it deducts the difference from subsequent sessions and if it doesn't reach it's target, it adds the difference to the subsequent day (so if you set a 1% per day target, it will make only that across the board). I'm thinking of writing some code to have it set it's own profit targets based on trading also, though still a WIP.
It can also handle multiple symbols on different timeframes (up to 5 at the moment).
Anyway, my question is centred around how to draw these boxes, not the programming of a Martingale system; you're right, a system has to be profitable already to consider incorporating Martingale in some form, otherwise all you're doing is creating larger exposure risk and doubling down on losing positions with no understanding on how the market works in terms of support and resistance, differentiating between trend and ranging, etc.
My system works without Martingale also. i.e. if I set up my grids to use addition rather than multiplication of lot sizes so therefore taking Martingale out of the equation totally, and set it to use both a core and outer grid (the former being equidistant and the second being either equidistant or variable based on either step addition or multiplication, it is still profitable, just not as profitable... :) I also never use a 2.0 multiplier (per the Wheat and Chessboard story, which is long before anyone called it Martingale!).
In relation to Martingale, all Paul Levy (1934) and Ville (1939) did was reinvent something that was in fact first analysed mathematically around 1200 A.D. as a mathematicians joke on a king when requesting payment for the invention of the chess board, if the story is to be believed...
I'm studying financial planning at university so look at all my strategies from a risk vs. reward and RoR perspective.
This article you sent ( Martingale vs. Non Martingale (Simplified RoR vs Profit and the Illusions) - Simple Trading Strategies - Expert Advisors and Automated Trading - MQL5 programming forum) was very interesting actually and is now filed away in my reading list! :)
With all of this said, find a system that makes you 1% on your account balance daily, cumulative, and in 5 years you'll be the richest man in the world (if the size of your trades don't eventually start moving the market itself)...
Whilst everyone says "Martingale is bad", the reality is that Martingale simply amplifies the effect, like any good guitarist or singer can tell you. If you're a terrible singer, the microphone isn't going to make you sound any better, just louder!
Martingale is what banks do every day except it's rather termed compound interest instead. e.g. I'll loan you $xyz dollars for x term, at y% interest and at the end you owe us $abc. Risk + compounding amounts that will continue to increase based on current balance if you don't pay it down (aka Martingale, though very-much bastardised using a far lower multiplier, what is 7% p.a. except a mulitiplier of 1.07 spaced over 365 days) and we're all being stung by the world's largest Martingale strategy every day, which is why very few people ever pay off their credit cards... banks basically use a "slow burn martingale" every day... the multiplier they're really basing their strategy on is the number of poeple accepting their offers... (principle x slow burn rate x 5,000,000,000 customers... lots of money)...
Pure Martingale at it's finest, also known as the "Wheat and Chessboard problem". :)
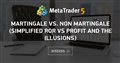
- 2015.02.08
- www.mql5.com
Hello there Charles,
The system you describe sounds pretty much like some ideas I started toying with a couple of years ago, however it looks like you've done some very serious work in terms of R&D here... I am truly impressed by the level of detail and nuances you described above.
Did you complete the project and launched the system live? Is it giving positive results as you expected? I'd love to exchange some impressions further about it, if you'd be so inclined.
Finally, did you manage to resolve the programming issue you were facing? If not, I'd be happy to give you a hand with the coding.

- Free trading apps
- Over 8,000 signals for copying
- Economic news for exploring financial markets
You agree to website policy and terms of use
Hi guys,
I'm creating a fairly complex Martingale grid trading system and would like some advice.
The desired outcome of the present component I'm programming is to colourize what I term "grids" (probably not the traditional understanding of what constitutes a grid in most Martingale strategies).
This "grid" I'm referring to is the creation of multiple entry points, followed by closure of the collective trades at a single point for a collective profit as a "grid", rather than referring to the method of placing limit orders in ever-increasing order sizes either equidistantly or at ever-increasing intervals.
What I would like to do is create a transparent box over the top of any "grids" that opened multiple entry points, drawing a semi-transparent green box for long trades and a red box for short trades (screenshot following).
This is only for any trades that have multiple entry points, not trades that have just a single entry and exit point.
Eventually as part of my next phase of programming I'd also like to colourize partially closed trades based on a rescue strategy in the event where drawdown is starting to becoming too great (as we all know this is the problem with pure martingale strategies) (i.e. the idea being something along the lines of a partial close method for individual trades that go into profit/break-even where multiple entry trades have been open for greater than a certain amount of time collectively, and to then colour these as a different colour than the single exit point trades (i.e. maybe an amber colour so they'll stand out visibly and can be counted to measure percentage execution where drawdown became a problem, so this can be analysed further).
Thus far I've been working through the following code (the entire robot is about 8,000 lines of code so far) and I already have entry and exit prices displaying correctly and colouring themselves correctly, along with being of different types based on whether they're entry points to trades or not.
So, my question is realistically in relation to best programming technique and whether it is easier/faster to start a counter based on which side and colour the screen object is on (i.e. BuyColour/SellColour and OBJ_ARROW_RIGHT_PRICE/LEFT_PRICE) to count when there are multiples of the same side object and colour to denote when to start drawing the box (i.e. when there's a RIGHT_PRICE and it's BuyColour, which signifies the closure of a short position grid, start counting LEFT_PRICE objects of the opposite colour until it changes) followed by triggering an event to get the coordinates for the close event, to allow for A-to-B drawing of the rectangle.
I've included the code I'm working through at the moment, plus have a screenshot with manually drawn boxes to display the type of arrangement I'd like to create.
Is it necessary to store this information into arrays and run different loops of lookbacks, or can anyone suggest a direct way through querying HistoryDeals that I may be able to use?
It's pretty mind-boggling stuff and I'm still somewhat trying to get my head around how I would essentially query a table/array to get enough information to draw the box from start to finish from point A to B based on the above.
Anyway, any assistance that anyone may be able to offer in suggesting a methodology would be greatly appreciated.
Regards,
Christian
P.S.: Programmers dilemma, "I just want it to draw a box from here to here...", always the hardest types of issues, it sounds simple though!