- pennyhunter: I am searching for a way to Fill an Array with some values in brackets
There is no searching; there is no way. Brackets only initialize an array with literal constants.
- pennyhunter: one string, then slices the subsrtings apart
StringSplit.
-
There is no searching; there is no way. Brackets only initialize an array with literal constants.
-
StringSplit.
Is there something like "array[]={value1, value2, value3, ...,valueN};" in MQL5
This is possible for constant values. You probably knew it but I'm pointing this out to not confuse beginners.
Example with constant strings:
string values[] = { "string1", "string2", "string3" }; // valid MQL
For non-constant values, like inputs etc, there's no clean solution. You can do this, utilizing string concatenation / StringSplit:
string fmt="%s"; for(int i=1; i<60; i++) fmt+="\001%s"; string str=StringFormat(fmt,String1,String2,String3); // <-- your inputs go here string values[]; StringSplit(str,'\001',values); ArrayPrint(values);
The number of strings you can add on the colored line can be arbitrary, up to 60. StringFormat will replace missing arguments with '(missed string parameter)'.
It's left to you as an exercise to determine the true number of entries and to resize the result array accordingly.
Another possibility would be to use a template with default parameters. You mentioned that above so I don't stretch it out here.
Or you can use ArrayInsert(), e.g. in OOP:
class CTest { private: double m_Coeff[9]; // cannot be set here ... } CTest::CTest (...) { double a[] = {0.95,0.97,0.98,0.99,1.0,1.01,1.03,1.05,1.1}; ArrayInsert(m_Coeff, a, 0); ... }
This way one don't need neither a conversion string => double nor a loop over all elements.
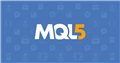
- www.mql5.com
Thank you guys!
Although I haven't got Carl's Approach to run, it served me as a welcomed inspiration.
double array[] = {0.95,0.97,0.98,0.99,1.0,1.01,1.03,1.05,1.1}; int index = 5; double result = 0.0; //+------------------------------------------------------------------+ void OnTick() { //--- result=Issue(array,index); Comment("RESULT = ",result); } //+------------------------------------------------------------------+ double Issue(const double &a[] , const int &i) { double res; res = a[i]; return(res); }
I am just not good with class Synthax yet. But maybe you have a tip for me. Kept playing around with it using scraps of knowledge from other classes until here (and it runs):
double array[] = {0.95,0.97,0.98,0.99,1.0,1.01,1.03,1.05,1.1}; int index = 5; double result = 0.0; //+------------------------------------------------------------------+ //| Expert tick function | //+------------------------------------------------------------------+ void OnTick() { //--- CTest test(array); result=test.Issue(index); index++; index*=(index<ArraySize(array)); Comment("RESULT = ",result); } //+------------------------------------------------------------------+ class CTest { private: double m_Coeff[]; // cannot be set here public: void CTest(const double &a[]); void ~CTest(void); double Issue(const int &i); }; CTest::CTest (const double &a[]) { ArrayResize(m_Coeff,ArraySize(a),1); ArrayInsert(m_Coeff, a, 0); } CTest::~CTest(void) { } double CTest::Issue(const int &i) { return(m_Coeff[i]); }
Even shows all the ingredients of m_Coeff[]
But for practicality reasons I would like to write the Issue() method in the class header like so:
double Issue(const int &i); const {return(m_Coeff[i]); }
But it won't compile, say something about declaration without type. Looking at other classes I can't see why tbh.
double Issue(const int &i); const {return(m_Coeff[i]); }
Just remove this.
Instead of using a named function you could overload the array operator:
double operator[](const int i) const { return m_Coeff[i]; }

- Free trading apps
- Over 8,000 signals for copying
- Economic news for exploring financial markets
You agree to website policy and terms of use
I am searching for a way to Fill an Array with some values in brackets so I don't have to mess around with indices for doing that like
string array[1]="name1";
string array[2]="name2";
string array[3]="name3";
and so on...
I got the idea in a python tutorial and now I have a similar problem in MQL5 and I can't imagine nobody ever came across this and said "Huh this looks kind of clunky and by the way it defies all efforts to not copy code..."
I am thinking about building an overload function for this, but I would still have to do it for a certain amount of overloads I wanted to use an arbitrary amount of overloads, would I use a Custom Indicator that just returns its inputs as variables in Oninit?
One other idea is to build a small kind of overload interpreter where I can input the whole soon to be array as one string, then slices the subsrtings apart at the separators and puts them in individual array variables after Upping ArraySize at every iteration... suggestions?
And yes I would skip all of that if there is an easy existing way but if there isn't I will seriously build it myself and post it here. I am not kidding.