Hello, I have the following piece of code:
Now I get the error " 'clickDatapoint' - objects are passed by reference only" at List<clickDatapoint> and I don't really know how to fix it.
Hello, I have the following piece of code:
Now I get the error " 'clickDatapoint' - objects are passed by reference only" at List<clickDatapoint> and I don't really know how to fix it.
Fix it by learning to program:
A struct is not a variable, it a kind of a type for variables and you haven't declared any variable of that type and finally you can only pass a variable to a function and of this type (struct) only by reference.
Actually it is a custom lib: https://github.com/dingmaotu/mql4-lib
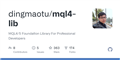
- github.com
Fix it by learning to program:
A struct is not a variable, it a kind of a type for variables and you haven't declared any variable of that type and finally you can only pass a variable to a function and of this type (struct) only by reference.
"learning to program", thats why I am asking questions...
I didn't really get it: When I create a list, I define which type its elements should have, for datetime for example List<datetime>. What is the difference to saying List<clickDatapoint> which to me means I am want a list that only contains clickDatapoint-structs. Or do I have to define my datapoint as a class to do this?
https://github.com/dingmaotu/mql4-lib/blob/master/Collection/Collection.mqh#L142
One of the problems is this line, the template just substitutes the type and it becomes in your example:
virtual bool contains(const clickDatapoint value) const
Which is invalid for MQL since clickDatapoint is an object, it should be passed as reference:
"MQL5 uses both methods, with one exception: arrays, structure type variables and class objects are always passed by reference. In order to avoid changes in actual parameters (arguments passed at function call) use the access specifier const. When trying to change the contents of a variable declared with the const specifier, the compiler will generate an error."
virtual bool contains(const clickDatapoint & value) const
The easiest solution without having to modify the template is to use clickDatapoint pointers:
datetime minimum(List<clickDatapoint*> &data)
But you would have to remember to delete them.
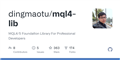
- github.com
Okay thank you so far. Anyways I decided to switch to a class and also to save the price data as MqlRates:
class ClickDatapoint { private: const MqlRates priceData; const CLICK_TYPE clickType; public: ClickDatapoint(const MqlRates &price_data, const CLICK_TYPE &click_type); ~ClickDatapoint(); const MqlRates getPriceData() const; const CLICK_TYPE getClickType() const; }; ClickDatapoint::ClickDatapoint(const MqlRates &price_data, const CLICK_TYPE &click_type): priceData(price_data) ,clickType(click_type) {}
Now in the constructor I get this error:
'MqlRates' - function not defined
Okay thank you so far. Anyways I decided to switch to a class and also to save the price data as MqlRates:
Now in the constructor I get this error:
'MqlRates' - function not defined
MqlRates b; MqlRates a(b);And it shows the same error, I consider it a bug, not sure if it is fixed in the latest metatrader build.
Looks like MqlRates does not have a copy constructor, I tried:
And it shows the same error, I consider it a bug, not sure if it is fixed in the latest metatrader build.
This means my only way to solve this is to remove const from priceData and init it like this:
ClickDatapoint::ClickDatapoint(const MqlRates &price_data, const CLICK_TYPE &click_type): clickType(click_type) { priceData = price_data; }
Or is there a better way to do it?
This means my only way to solve this is to remove const from priceData and init it like this:
Or is there a better way to do it?
An alternative would be to create a wrapper class that accepts the MqlRates in its constructor and expose the members you want,
that way you could code it to work in that case.

- Free trading apps
- Over 8,000 signals for copying
- Economic news for exploring financial markets
You agree to website policy and terms of use
Hello, I have the following piece of code:
Now I get the error " 'clickDatapoint' - objects are passed by reference only" at List<clickDatapoint> and I don't really know how to fix it.