Get Highest/Lowest value of Fractals of the last x bars ---- result is 3.0 or 4.0 or 15.0 instead of the real value of the fractals
Hello,
i try to get highest/lowest Fractal of the last x bars, it will compile but onTick gives back array out of range error. Does this happen because at first bar of backtest we do not have access to the last 15 bars? If yes, how can i fix that? Any help would be very appreciated :)
greetz
Array out of range error is because size of upper and lower arrays is not set.
input int PSAR_max_bar = 15; // bars on which you want to find fractals, max = 20 int handle_iFractals; // fractal handle int OnInit() { handle_iFractals=iFractals(Symbol(),Period()); if(handle_iFractals==INVALID_HANDLE) { PrintFormat("Failed to create handle of the iFractals indicator for the symbol %s/%s, error code %d", m_symbol.Name(), EnumToString(Period()), GetLastError()); return(INIT_FAILED); } return(INIT_SUCCEEDED); } void OnTick() { double upper[20]; double lower[20]; for(int i=3; i<PSAR_max_bar; i++) { upper[i]=iFractalsGet(UPPER_LINE,i); lower[i]=iFractalsGet(LOWER_LINE,i); } double maxUpper = ArrayMaximum(upper,WHOLE_ARRAY,0); double maxLower = ArrayMinimum(lower,WHOLE_ARRAY,0); Print(maxUpper," - ",maxLower); } double iFractalsGet(const int buffer,const int index) { double Fractals[1]; //--- reset error code ResetLastError(); //--- fill a part of the iFractalsBuffer array with values from the indicator buffer that has 0 index if(CopyBuffer(handle_iFractals,buffer,index,1,Fractals)<0) { //--- if the copying fails, tell the error code PrintFormat("Failed to copy data from the iFractals indicator, error code %d",GetLastError()); //--- quit with zero result - it means that the indicator is considered as not calculated return(0.0); } return(Fractals[0]); }
Hello Navdeep,
thank you for your help, the EA does not giva any array error anymore. But the function does not give back the right value of the fractals, the result for maxUpper is 3.0 or 4.0, while maxLower is 15.0. The real value is about 0,71150 because its NZDUSD chart.
greetz
Hello Navdeep,
thank you for your help, the EA does not giva any array error anymore. But the function does not give back the right value of the fractals, the result for maxUpper is 3.0 or 4.0, while maxLower is 15.0. The real value is about 0,71150 because its NZDUSD chart.
greetz
Your parameters for ArrayMaximum/Minimum functions are wrong. You are trying to use the MQL4 function in MQL5. See correct example here
Also if you are only filling from the 4th element up to the 15th element then keep your search within that range
Or
write proper code and only copy non-empty values from fractals handle into the arrays.
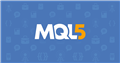
- www.mql5.com

- Free trading apps
- Over 8,000 signals for copying
- Economic news for exploring financial markets
You agree to website policy and terms of use
Hello,
i try to get highest/lowest Fractal of the last x bars, it will compile but onTick gives back array out of range error. Does this happen because at first bar of backtest we do not have access to the last 15 bars? If yes, how can i fix that? Any help would be very appreciated :)
greetz