to stress it I will say again I do not want to use ArrayCopy , this function will copy data, I want to reference b to a.
There are no pointers in MTx; only handles to classes, nothing to POD (arrays). What you want can not be done.
Either ArrayCopy and ArrayFree b, or free a and just use b
There are no pointers in MTx; only handles to classes, nothing to POD (arrays). What you want can not be done.
Either ArrayCopy and ArrayFree b, or free a and just use b
Thanks for your response there is a function named GetPointer which get class pointers! I couldn't understand what reference said, but I think there may be a way to crack it.
this is as fur as i could go:
//+------------------------------------------------------------------+ //| Script program start function | //+------------------------------------------------------------------+ void OnStart() { //--- A a[]; A *ptr; ArrayResize(a, 2); a[0].intA = 0; a[1].intA = 1; ptr = CalculateFunction(a); Print(ptr.intA); // log should output 3 and a array sie should be 3 and old a should be derefrenced to free up memory and b should be defrefrenced to avid resource leak. } //+------------------------------------------------------------------+ //| | //+------------------------------------------------------------------+ class A { public: int intA; A() { intA = 1; } }; //+------------------------------------------------------------------+ //| | //+------------------------------------------------------------------+ A * CalculateFunction(A &localA[]) { A b; b[0].intA = 2; b[1].intA = 3; b[2].intA = 4; A * c[] = GetPointer(b); return c; }https://www.mql5.com/en/docs/common/getpointer
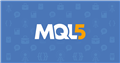
- www.mql5.com
But you also need a class to capsule your array.
A b; ⋮ A * c[] = GetPointer(b); return c;
The moment you return, b goes out of scope and your pointer is invalid.
But you also need a class to capsule your array.
There is no benefit to that, I want to do so, in order to boost calculation speed, your approach will raise complexity and reduce speed way more than using ArrayCopy.
Btw, overloading an Operator or calling a function is in fact the same.
I guess, it's a question of viewpoint. Maybe my imagination of the code is different than yours.
My idea would include a templated class and two overloaded assignment operators to capsule your requested feature.
I am not sure if the calling of these functions are significant to the speed of execution, compared to a procedural approach.
In fact I doubt it.

- Free trading apps
- Over 8,000 signals for copying
- Economic news for exploring financial markets
You agree to website policy and terms of use
Hi, I have an array object named "a" , after Appling a function to it, array object named "b" will be produced, for speed up proposes I want to assign b pointer to a and free up memory used by "a".
can any body help please.
log should output 3 and a array size should be 3 and old a should be dereferenced to free up memory and b should be dereferenced to avid resource leak.
to stress it I will say again I do not want to use ArrayCopy , this function will copy data, I want to reference b to a.